posix thread 封装成 c++类
时间: 2023-08-31 10:09:49 浏览: 91
以下是一个简单的 POSIX 线程封装类的示例代码:
```c++
#include <pthread.h>
class Thread {
public:
Thread() : m_tid(0), m_running(false) {}
virtual ~Thread() {}
bool start() {
int err = pthread_create(&m_tid, NULL, Thread::thread_func, this);
if (err != 0) {
return false;
}
m_running = true;
return true;
}
void join() {
if (m_tid > 0) {
pthread_join(m_tid, NULL);
}
}
bool is_running() const {
return m_running;
}
protected:
virtual void run() = 0;
private:
static void* thread_func(void* arg) {
Thread* thread = static_cast<Thread*>(arg);
thread->run();
thread->m_running = false;
return NULL;
}
private:
pthread_t m_tid;
bool m_running;
};
```
这个类封装了 POSIX 线程的创建、销毁和等待等操作,使用起来非常简单。只需要继承 `Thread` 类并实现 `run()` 方法即可。
例如,我们可以创建一个简单的打印线程:
```c++
#include <iostream>
class PrintThread : public Thread {
public:
PrintThread(int count) : m_count(count) {}
protected:
void run() {
for (int i = 0; i < m_count; ++i) {
std::cout << "Hello, world!" << std::endl;
}
}
private:
int m_count;
};
int main() {
PrintThread thread(3);
thread.start();
thread.join();
return 0;
}
```
这个程序会创建一个打印线程,该线程会打印 3 次 `"Hello, world!"`,然后退出。在主线程中,我们通过调用 `start()` 方法启动线程,然后等待线程结束,最后退出。
阅读全文
相关推荐

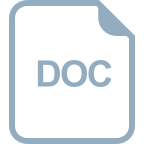
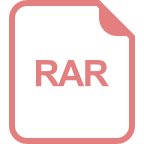











