重新编写 Vec2D 类,并在其中实现静态方法 convert2Vec2D(other),当 other 是元组或列表时将其转换为 Vec2D 对象并返回转换后的 Vec2D 对象;另外实现必要的特殊方法。
时间: 2023-06-15 08:07:09 浏览: 142
以下是重新编写后的 Vec2D 类:
```python
class Vec2D:
def __init__(self, x, y):
self.x = x
self.y = y
def __str__(self):
return f"({self.x}, {self.y})"
def __repr__(self):
return f"Vec2D({self.x}, {self.y})"
def __eq__(self, other):
if isinstance(other, Vec2D):
return self.x == other.x and self.y == other.y
return False
def __add__(self, other):
if isinstance(other, Vec2D):
return Vec2D(self.x + other.x, self.y + other.y)
raise TypeError("unsupported operand type(s) for +: 'Vec2D' and " + str(type(other)))
def __sub__(self, other):
if isinstance(other, Vec2D):
return Vec2D(self.x - other.x, self.y - other.y)
raise TypeError("unsupported operand type(s) for -: 'Vec2D' and " + str(type(other)))
def __mul__(self, other):
if isinstance(other, (int, float)):
return Vec2D(self.x * other, self.y * other)
raise TypeError("unsupported operand type(s) for *: 'Vec2D' and " + str(type(other)))
def __rmul__(self, other):
return self.__mul__(other)
def __truediv__(self, other):
if isinstance(other, (int, float)):
return Vec2D(self.x / other, self.y / other)
raise TypeError("unsupported operand type(s) for /: 'Vec2D' and " + str(type(other)))
@staticmethod
def convert2Vec2D(other):
if isinstance(other, (tuple, list)) and len(other) == 2:
return Vec2D(other[0], other[1])
raise TypeError("cannot convert " + str(type(other)) + " to Vec2D")
```
在上述代码中,我们实现了以下特殊方法:
- `__eq__(self, other)`:用于比较两个 Vec2D 对象是否相等。
- `__add__(self, other)`:用于向量加法。
- `__sub__(self, other)`:用于向量减法。
- `__mul__(self, other)`:用于向量数乘。
- `__rmul__(self, other)`:用于右乘操作。
- `__truediv__(self, other)`:用于向量数除。
除此之外,我们还实现了一个静态方法 `convert2Vec2D(other)`,当 `other` 是元组或列表时将其转换为 Vec2D 对象并返回转换后的 Vec2D 对象。
可以使用以下代码测试 Vec2D 类的功能:
```python
v1 = Vec2D(1, 2)
v2 = Vec2D(3, 4)
# 测试向量加法
print(v1 + v2) # 输出 (4, 6)
# 测试向量减法
print(v1 - v2) # 输出 (-2, -2)
# 测试向量数乘
print(v1 * 2) # 输出 (2, 4)
# 测试向量数除
print(v2 / 2) # 输出 (1.5, 2.0)
# 测试静态方法
print(Vec2D.convert2Vec2D((5, 6))) # 输出 (5, 6)
# 测试相等性
print(Vec2D(1, 2) == Vec2D(1, 2)) # 输出 True
print(Vec2D(1, 2) == Vec2D(2, 3)) # 输出 False
```
相关推荐
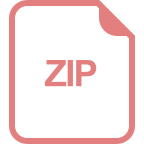
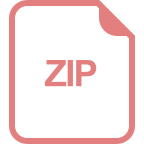
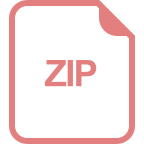














