tf.keras.callbacks.earlystopping
时间: 2023-04-25 22:02:50 浏览: 172
tf.keras.callbacks.earlystopping是TensorFlow中的一个回调函数,用于在训练过程中监测模型的性能,并在模型性能不再提升时停止训练。它可以通过监测验证集的损失或准确率来判断模型是否过拟合,并在达到指定的停止条件时停止训练,从而避免浪费时间和计算资源。
相关问题
callbacks = [ tf.keras.callbacks.EarlyStopping(monitor='val_accuracy', patience=4, min_delta=0.02, restore_best_weights=True), tf.keras.callbacks.LearningRateScheduler(warmup, verbose=0), tf.keras.callbacks.ReduceLROnPlateau(monitor='val_accuracy', factor=1e-6, patience=2, verbose=0, mode='auto', min_delta=0.001, cooldown=0, min_lr=1e-6) ]
这段代码是使用 TensorFlow 中的 Keras 库来设置训练过程中的回调函数。
1. `EarlyStopping` 回调函数用于在训练过程中监控指定的指标(这里是验证集准确率 `val_accuracy`),如果连续 `patience` 轮的指标没有改善超过 `min_delta`,则提前停止训练,并恢复最佳权重。这有助于避免过拟合。
2. `LearningRateScheduler` 回调函数用于在训练过程中动态地调整学习率。它接受一个自定义的学习率调度函数 `warmup`,该函数根据训练的当前轮数来计算学习率的值。
3. `ReduceLROnPlateau` 回调函数用于在验证集上监控指定的指标(这里是验证集准确率 `val_accuracy`),如果指标在 `patience` 轮内没有改善超过 `min_delta`,则将学习率乘以 `factor` 来降低学习率。这有助于使训练过程更加稳定,并帮助模型找到更好的局部最优解。
这些回调函数可以通过将它们添加到模型的 `fit` 方法中的 `callbacks` 参数来使用,例如:
```python
model.fit(x_train, y_train, callbacks=callbacks)
```
通过使用这些回调函数,可以在训练过程中监控指标、动态调整学习率,并在需要时提前停止训练以获得更好的模型性能。
#train checkpoint_path = './model_pth/cnn.h5' checkpoint = tf.keras.callbacks.ModelCheckpoint(checkpoint_path, monitor='val_accuracy', verbose=1,save_best_only=True, mode='max') early_stopping = tf.keras.callbacks.EarlyStopping(monitor ='val_loss', patience=200, verbose=1) optimizer = tf.keras.optimizers.Adam(learning_rate=1e-4, epsilon=1e-7)
这段代码是用 TensorFlow 2.x 版本编写的卷积神经网络模型训练代码,包括了模型的保存、早停和优化器等功能。具体的代码实现如下(Python语言):
```python
# 导入需要的库
import tensorflow as tf
from tensorflow import keras
# 定义模型
model = keras.Sequential([
keras.layers.Conv2D(32, (3,3), activation='relu', input_shape=(28,28,1)),
keras.layers.MaxPooling2D((2,2)),
keras.layers.Flatten(),
keras.layers.Dense(10, activation='softmax')
])
# 编译模型
model.compile(optimizer=tf.keras.optimizers.Adam(learning_rate=1e-4, epsilon=1e-7),
loss='categorical_crossentropy',
metrics=['accuracy'])
# 定义回调函数
checkpoint_path = './model_pth/cnn.h5'
checkpoint = tf.keras.callbacks.ModelCheckpoint(checkpoint_path, monitor='val_accuracy', verbose=1, save_best_only=True, mode='max')
early_stopping = tf.keras.callbacks.EarlyStopping(monitor='val_loss', patience=200, verbose=1)
# 训练模型
model.fit(train_images, train_labels, epochs=1000, batch_size=128,
validation_data=(test_images, test_labels),
callbacks=[checkpoint, early_stopping])
```
其中,`tf.keras.callbacks.ModelCheckpoint` 和 `tf.keras.callbacks.EarlyStopping` 分别实现了模型保存和早停的功能,`tf.keras.optimizers.Adam` 则是优化器的设置。需要注意的是,这里的模型是一个简单的卷积神经网络,输入为 28x28 的灰度图像,输出为 10 类别的概率值。
阅读全文
相关推荐
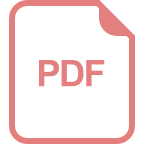
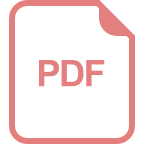
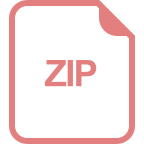











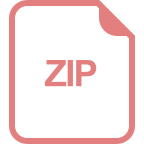
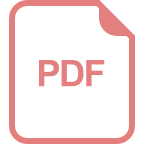