python 用ad域校验用户
时间: 2023-07-12 18:21:42 浏览: 197
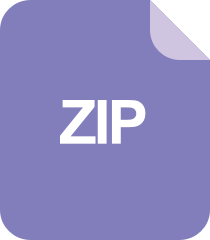
一个基于Python + Django的AD管理系统源码.zip

可以使用 Python 的 `ldap` 模块连接 AD 域,实现用户的校验。
以下是一个简单的示例代码:
```python
import ldap
# AD 域服务器地址和端口
AD_SERVER = 'ldap://your-ad-server:389'
# AD 域管理员账号和密码
AD_ADMIN_USERNAME = 'your-admin-username'
AD_ADMIN_PASSWORD = 'your-admin-password'
# AD 域中的用户基础 DN
AD_BASE_DN = 'dc=your-domain,dc=com'
def authenticate(username, password):
# 连接 AD 域
conn = ldap.initialize(AD_SERVER)
conn.simple_bind_s(AD_ADMIN_USERNAME, AD_ADMIN_PASSWORD)
# 搜索指定用户
filter_str = f'(sAMAccountName={username})'
search_res = conn.search_s(AD_BASE_DN, ldap.SCOPE_SUBTREE, filter_str, ['memberOf'])
# 如果搜索结果为空,则认证失败
if not search_res:
return False
# 组装用户 DN
user_dn, user_attrs = search_res[0]
if isinstance(user_dn, bytes):
user_dn = user_dn.decode()
user_dn = user_dn.replace('\\', '\\\\').replace(',', '\\,').replace('+', '\\+').replace('"', '\\"')
user_dn = f'cn={username},{user_dn}'
# 尝试使用用户 DN 和密码认证
try:
conn.simple_bind_s(user_dn, password)
except ldap.INVALID_CREDENTIALS:
return False
# 认证成功
return True
```
调用 `authenticate` 函数即可校验用户。例如:
```python
if authenticate('your-username', 'your-password'):
print('Authentication success')
else:
print('Authentication failed')
```
当然,使用 `ldap` 模块需要先安装:`pip install python-ldap`。
阅读全文
相关推荐
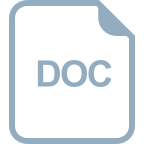
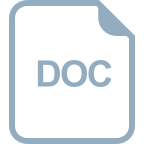

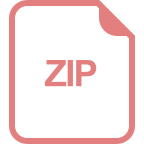
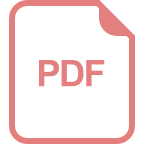
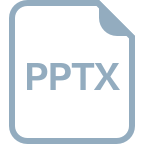
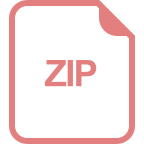
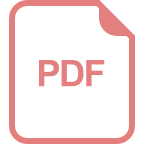
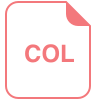
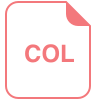
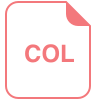
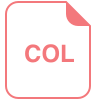
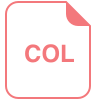
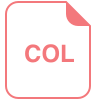
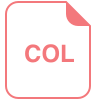


