C语言用链表存储读取文件信息并打印
时间: 2023-08-04 17:28:45 浏览: 101
下面是一个使用链表存储文件信息并打印的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct node {
int data;
struct node* next;
};
struct list {
struct node* head;
struct node* tail;
};
void print_list(struct list mylist) {
struct node* current = mylist.head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main() {
FILE* fp;
fp = fopen("data.txt", "r");
if (fp == NULL) {
printf("Failed to open file.\n");
return 0;
}
struct list mylist;
mylist.head = NULL;
mylist.tail = NULL;
int data;
while (fscanf(fp, "%d", &data) != EOF) {
struct node* new_node = (struct node*)malloc(sizeof(struct node));
new_node->data = data;
new_node->next = NULL;
if (mylist.head == NULL) {
mylist.head = new_node;
mylist.tail = new_node;
} else {
mylist.tail->next = new_node;
mylist.tail = new_node;
}
}
fclose(fp);
print_list(mylist);
return 0;
}
```
这个程序打开名为"data.txt"的文件,读取其中的整数并将它们存储在链表中。然后,它调用"print_list"函数遍历链表并打印所有元素。
阅读全文
相关推荐



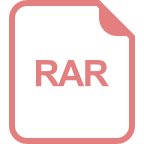











