c语言代码实现arp字段解析
时间: 2024-02-19 08:02:31 浏览: 241
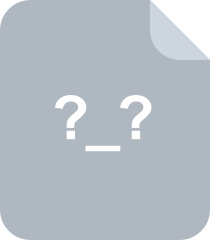
arp的C语言程序源代码

ARP(Address Resolution Protocol)是一种用于将IPv4地址解析为MAC地址的协议。以下是一段C语言代码,用于解析ARP数据包中的各个字段:
```c
#include <stdio.h>
#include <stdlib.h>
#include <netinet/in.h>
#include <arpa/inet.h>
/* 定义ARP报文格式 */
struct arp_hdr {
uint16_t hw_type; /* 硬件类型 */
uint16_t proto_type; /* 协议类型 */
uint8_t hw_len; /* 硬件地址长度 */
uint8_t proto_len; /* 协议地址长度 */
uint16_t op_code; /* 操作码 */
uint8_t src_hw_addr[6]; /* 源MAC地址 */
uint8_t src_ip_addr[4]; /* 源IP地址 */
uint8_t dst_hw_addr[6]; /* 目的MAC地址 */
uint8_t dst_ip_addr[4]; /* 目的IP地址 */
};
/* 解析ARP报文 */
void parse_arp_packet(const uint8_t* packet, size_t packet_len) {
const struct arp_hdr* arp = (const struct arp_hdr*)packet;
/* 打印各个字段的值 */
printf("Hardware type: %d\n", ntohs(arp->hw_type));
printf("Protocol type: %d\n", ntohs(arp->proto_type));
printf("Hardware address length: %d\n", arp->hw_len);
printf("Protocol address length: %d\n", arp->proto_len);
printf("Operation code: %d\n", ntohs(arp->op_code));
printf("Source MAC address: %02x:%02x:%02x:%02x:%02x:%02x\n",
arp->src_hw_addr[0], arp->src_hw_addr[1], arp->src_hw_addr[2],
arp->src_hw_addr[3], arp->src_hw_addr[4], arp->src_hw_addr[5]);
printf("Source IP address: %s\n", inet_ntoa(*(struct in_addr*)arp->src_ip_addr));
printf("Destination MAC address: %02x:%02x:%02x:%02x:%02x:%02x\n",
arp->dst_hw_addr[0], arp->dst_hw_addr[1], arp->dst_hw_addr[2],
arp->dst_hw_addr[3], arp->dst_hw_addr[4], arp->dst_hw_addr[5]);
printf("Destination IP address: %s\n", inet_ntoa(*(struct in_addr*)arp->dst_ip_addr));
}
/* 主程序 */
int main() {
/* 假设收到的ARP数据包保存在packet_buf中 */
uint8_t packet_buf[] = {
0x00, 0x01, 0x08, 0x00, 0x06, 0x04, 0x00, 0x01, /* ARP头部 */
0x08, 0x00, 0x27, 0x1a, 0x67, 0x1b, 0xc0, 0xa8, /* 源MAC地址和IP地址 */
0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0xc0, 0xa8, /* 目的MAC地址和IP地址 */
0x01, 0x01, 0x01, 0x01
};
size_t packet_len = sizeof(packet_buf);
parse_arp_packet(packet_buf, packet_len);
return 0;
}
```
上述代码中,我们定义了一个`struct arp_hdr`类型,用于表示ARP报文的各个字段。然后,我们通过强制类型转换将收到的ARP数据包转换为该类型,然后打印各个字段的值。最后,我们可以通过修改`packet_buf`数组中的数据,来测试不同的ARP数据包。
阅读全文
相关推荐

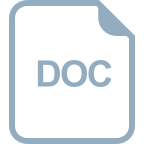
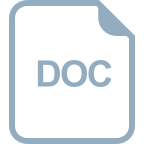
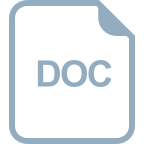
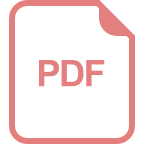
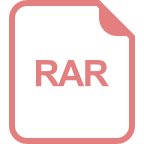
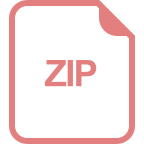
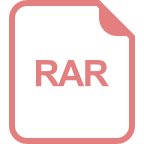
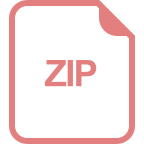
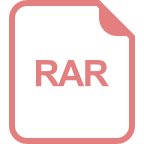
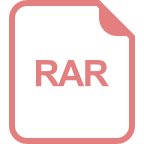
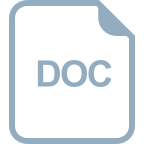
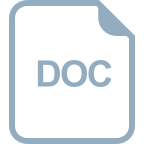
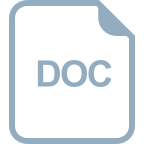
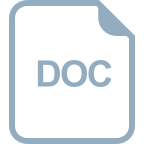
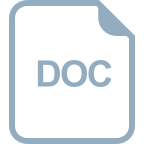