用栈写一个迷宫的源代码附带注释
时间: 2023-04-03 20:04:19 浏览: 71
好的,以下是用栈写一个迷宫的源代码,附带注释:
#include <stdio.h>
#include <stdlib.h>
#define MAX_ROW 5
#define MAX_COL 5
// 定义迷宫的结构体
struct point {
int row, col;
} stack[512];
int top = 0;
// 定义迷宫的地图
int maze[MAX_ROW][MAX_COL] = {
0, 1, 0, 0, 0,
0, 1, 0, 1, 0,
0, 0, 0, 0, 0,
0, 1, 1, 1, 0,
0, 0, 0, 1, 0,
};
// 定义栈的操作函数
void push(struct point p)
{
stack[top++] = p;
}
struct point pop(void)
{
return stack[--top];
}
int is_empty(void)
{
return top == 0;
}
// 定义迷宫的搜索函数
void print_maze(void)
{
int i, j;
for (i = 0; i < MAX_ROW; i++) {
for (j = 0; j < MAX_COL; j++)
printf("%d ", maze[i][j]);
putchar('\n');
}
printf("*********\n");
}
// 判断当前位置是否可以通过
int can_go(struct point p)
{
if (p.row < 0 || p.row >= MAX_ROW ||
p.col < 0 || p.col >= MAX_COL)
return 0;
if (maze[p.row][p.col] != 0)
return 0;
return 1;
}
// 搜索迷宫
int main(void)
{
struct point p = { 0, 0 };
maze[p.row][p.col] = 2;
push(p);
while (!is_empty()) {
p = pop();
if (p.row == MAX_ROW - 1 && p.col == MAX_COL - 1)
break;
if (can_go((struct point) { p.row - 1, p.col })) {
maze[p.row - 1][p.col] = 2;
push((struct point) { p.row - 1, p.col });
}
if (can_go((struct point) { p.row + 1, p.col })) {
maze[p.row + 1][p.col] = 2;
push((struct point) { p.row + 1, p.col });
}
if (can_go((struct point) { p.row, p.col - 1 })) {
maze[p.row][p.col - 1] = 2;
push((struct point) { p.row, p.col - 1 });
}
if (can_go((struct point) { p.row, p.col + 1 })) {
maze[p.row][p.col + 1] = 2;
push((struct point) { p.row, p.col + 1 });
}
print_maze();
}
if (p.row == MAX_ROW - 1 && p.col == MAX_COL - 1)
printf("(%d, %d)\n", p.row, p.col);
else
printf("No path!\n");
return 0;
}
相关推荐
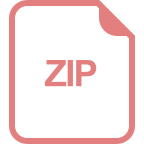
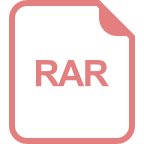














