给我一份使用mfc实现图像分割的程序
时间: 2024-03-26 13:42:15 浏览: 64
以下是一个使用 MFC 实现图像分割的示例程序,基于 OpenCV 库,可以实现基于 K-Means 算法的图像分割:
```
#include "stdafx.h"
#include "ImageSegmentation.h"
#include <opencv2/opencv.hpp>
using namespace cv;
// 定义图像分割的函数
void kmeans_segmentation(Mat& src, Mat& dst, int clusters);
int main()
{
// 读入图像
Mat src = imread("test.jpg", IMREAD_COLOR);
// 定义分割后的图像
Mat dst;
// 调用 kmeans_segmentation 函数进行分割
kmeans_segmentation(src, dst, 3);
// 显示原始图像和分割后的图像
imshow("Original Image", src);
imshow("Segmented Image", dst);
// 等待按键
waitKey(0);
return 0;
}
void kmeans_segmentation(Mat& src, Mat& dst, int clusters)
{
// 转换图像为二维矩阵
Mat samples(src.rows * src.cols, 3, CV_32F);
for (int i = 0; i < src.rows; i++) {
for (int j = 0; j < src.cols; j++) {
samples.at<float>(i * src.cols + j, 0) = src.at<Vec3b>(i, j)[0];
samples.at<float>(i * src.cols + j, 1) = src.at<Vec3b>(i, j)[1];
samples.at<float>(i * src.cols + j, 2) = src.at<Vec3b>(i, j)[2];
}
}
// 对样本进行 K-Means 聚类
Mat labels;
kmeans(samples, clusters, labels, TermCriteria(TermCriteria::EPS + TermCriteria::COUNT, 10, 1.0), 3, KMEANS_PP_CENTERS);
// 将每个像素点的标签转换为颜色
Mat colors(clusters, 1, CV_8UC3);
for (int i = 0; i < clusters; i++) {
int r = rand() % 256;
int g = rand() % 256;
int b = rand() % 256;
colors.at<Vec3b>(i, 0) = Vec3b(r, g, b);
}
// 创建输出图像
dst = Mat(src.size(), src.type());
for (int i = 0; i < src.rows; i++) {
for (int j = 0; j < src.cols; j++) {
int cluster_idx = labels.at<int>(i * src.cols + j);
dst.at<Vec3b>(i, j) = colors.at<Vec3b>(cluster_idx, 0);
}
}
}
```
这个程序可以读入一张图像并对其进行分割,分割后的图像将被显示在窗口中。其中,kmeans_segmentation 函数是实现图像分割的核心部分,它使用了 OpenCV 中的 kmeans 函数来进行 K-Means 聚类。
阅读全文
相关推荐

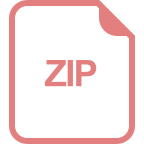
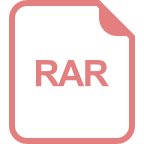
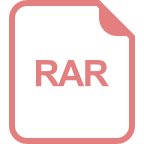
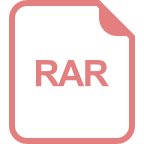
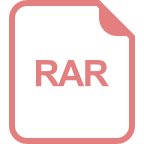
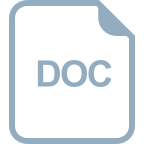
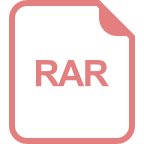
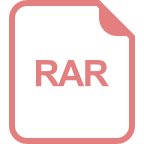
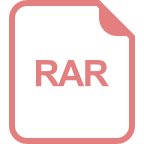
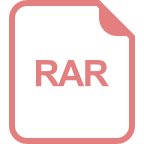
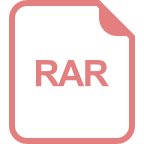
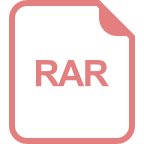
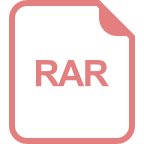
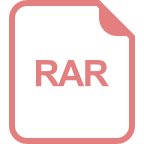
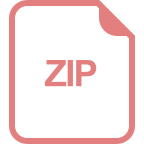