java swing 超市购物系统代码
时间: 2023-08-10 11:03:22 浏览: 184
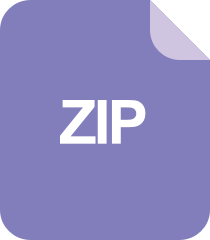
java+mysql+swing,包含完整代码报告数据库,可 理与购物系统超市管理与购物系统超市管理与购物系统超市管理与购物系统
好的,以下是一个简单的 Java Swing 超市购物系统的示例代码:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Supermarket extends JFrame implements ActionListener {
private JLabel nameLabel, priceLabel, quantityLabel, totalLabel;
private JTextField nameField, priceField, quantityField, totalField;
private JButton addButton, checkoutButton;
private JList<String> cartList;
private DefaultListModel<String> cartModel;
public Supermarket() {
// 设置窗口标题
setTitle("超市购物系统");
// 创建商品信息标签和文本框
nameLabel = new JLabel("商品名称:");
priceLabel = new JLabel("商品单价:");
quantityLabel = new JLabel("商品数量:");
totalLabel = new JLabel("总价:");
nameField = new JTextField(10);
priceField = new JTextField(10);
quantityField = new JTextField(10);
totalField = new JTextField(10);
totalField.setEditable(false);
// 创建添加按钮和结算按钮
addButton = new JButton("添加到购物车");
checkoutButton = new JButton("结算");
// 创建购物车列表
cartModel = new DefaultListModel<>();
cartList = new JList<>(cartModel);
// 创建面板,并添加组件
JPanel panel = new JPanel(new GridBagLayout());
GridBagConstraints c = new GridBagConstraints();
c.gridx = 0;
c.gridy = 0;
c.anchor = GridBagConstraints.LINE_START;
panel.add(nameLabel, c);
c.gridx = 1;
c.gridy = 0;
panel.add(nameField, c);
c.gridx = 0;
c.gridy = 1;
panel.add(priceLabel, c);
c.gridx = 1;
c.gridy = 1;
panel.add(priceField, c);
c.gridx = 0;
c.gridy = 2;
panel.add(quantityLabel, c);
c.gridx = 1;
c.gridy = 2;
panel.add(quantityField, c);
c.gridx = 0;
c.gridy = 3;
panel.add(totalLabel, c);
c.gridx = 1;
c.gridy = 3;
panel.add(totalField, c);
c.gridx = 0;
c.gridy = 4;
c.gridwidth = 2;
panel.add(addButton, c);
c.gridx = 0;
c.gridy = 5;
panel.add(checkoutButton, c);
// 添加组件到窗口
add(panel, BorderLayout.NORTH);
add(new JScrollPane(cartList), BorderLayout.CENTER);
// 注册按钮事件监听器
addButton.addActionListener(this);
checkoutButton.addActionListener(this);
// 设置窗口大小和可见性
setSize(400, 300);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == addButton) {
// 添加商品到购物车
String name = nameField.getText();
double price = Double.parseDouble(priceField.getText());
int quantity = Integer.parseInt(quantityField.getText());
double total = price * quantity;
String item = String.format("%s (%d x %.2f = %.2f)", name, quantity, price, total);
cartModel.addElement(item);
totalField.setText(String.format("%.2f", getTotalPrice()));
clearFields();
} else if (e.getSource() == checkoutButton) {
// 结算购物车
double totalPrice = getTotalPrice();
JOptionPane.showMessageDialog(this, String.format("总价:%.2f", totalPrice));
cartModel.clear();
totalField.setText("");
}
}
private double getTotalPrice() {
double totalPrice = 0;
for (int i = 0; i < cartModel.getSize(); i++) {
String item = cartModel.getElementAt(i);
int start = item.indexOf('=') + 2;
int end = item.length() - 1;
double total = Double.parseDouble(item.substring(start, end));
totalPrice += total;
}
return totalPrice;
}
private void clearFields() {
nameField.setText("");
priceField.setText("");
quantityField.setText("");
}
public static void main(String[] args) {
new Supermarket();
}
}
```
在这个示例代码中,我们创建了一个 `Supermarket` 类,继承了 `JFrame` 类,实现了 `ActionListener` 接口。在构造方法中,我们创建了商品信息标签和文本框、添加按钮、结算按钮、购物车列表等组件,并将它们添加到窗口中。同时,我们还注册了按钮事件监听器,实现了添加商品到购物车和结算购物车的功能。
在 `actionPerformed` 方法中,我们根据事件源判断用户是点击了添加按钮还是结算按钮。如果是添加按钮,我们获取用户输入的商品信息,计算出总价,并将商品信息添加到购物车列表中。同时,我们还调用 `getTotalPrice` 方法计算购物车中所有商品的总价,并更新总价文本框的值。如果是结算按钮,我们调用 `getTotalPrice` 方法计算购物车中所有商品的总价,并弹出一个消息框显示总价。然后,我们清空购物车列表和总价文本框的值。
在 `getTotalPrice` 方法中,我们遍历购物车列表中的每个商品,计算出该商品的总价,并累加到 `totalPrice` 变量中。最后,返回总价。
在 `clearFields` 方法中,我们将商品名称、单价和数量文本框的值清空。
在 `main` 方法中,我们创建了一个 `Supermarket` 对象,启动了超市购物系统。
当然,这只是一个简单的示例代码,真正的超市购物系统需要考虑更多的功能和细节,如商品库存、优惠券、运费、地址、支付方式等等。但这个示例代码可以帮助您了解 Java Swing 的基本组件和事件处理机制。
阅读全文
相关推荐

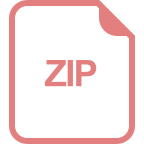
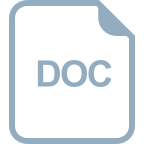
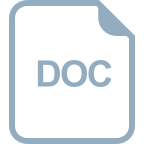
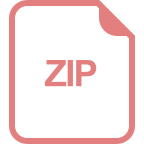
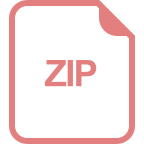
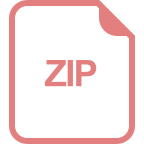
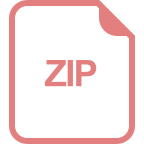
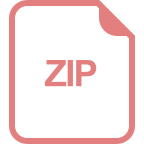
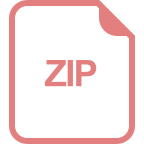
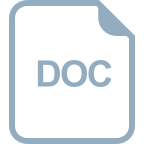
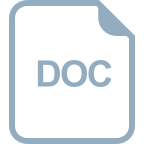
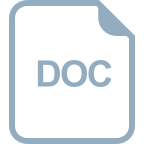
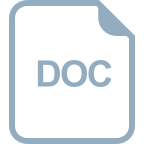
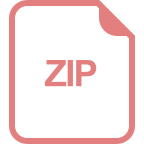
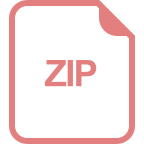