帮我生成训练集和验证集的精确度曲线。要求epoch=100,精确度由0.75缓慢上升到0.86结束,加入很小的白噪声,训练集的白噪声较小;训练集的精确度要稍微高于验证集
时间: 2023-12-05 17:05:18 浏览: 114
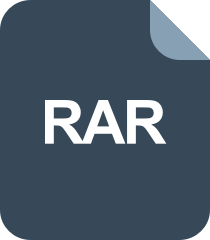
caffe 绘制训练集和测试集的loss和accuracy对比曲线

好的,我可以为您提供一个简单的示例代码来生成训练集和验证集的精确度曲线。在这个示例中,我们将使用PyTorch和Scikit-learn来生成数据和模型。
首先,我们需要生成一些模拟数据。我们可以使用Scikit-learn中提供的`make_classification`函数来生成二元分类数据。
```python
from sklearn.datasets import make_classification
import numpy as np
# Generate simulated data
X, y = make_classification(n_samples=1000, n_features=10, n_informative=8,
n_redundant=2, n_classes=2, random_state=42)
# Add some noise to the data
noise = np.random.normal(0, 0.1, size=X.shape)
X += noise
```
接下来,我们将使用PyTorch来定义一个简单的神经网络模型。在这个示例中,我们将使用一个具有两个隐藏层的全连接神经网络。
```python
import torch
import torch.nn as nn
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(10, 20)
self.fc2 = nn.Linear(20, 10)
self.fc3 = nn.Linear(10, 2)
self.relu = nn.ReLU()
def forward(self, x):
x = self.fc1(x)
x = self.relu(x)
x = self.fc2(x)
x = self.relu(x)
x = self.fc3(x)
return x
```
接下来,我们将使用PyTorch和Scikit-learn来训练和测试模型。我们将使用Adam优化器和交叉熵损失函数来训练模型。我们将记录训练和验证的准确性,并在训练完成后绘制准确性曲线。
```python
import torch.optim as optim
from sklearn.model_selection import train_test_split
import matplotlib.pyplot as plt
# Split data into training and validation sets
X_train, X_val, y_train, y_val = train_test_split(X, y, test_size=0.2, random_state=42)
# Convert data to PyTorch tensors
X_train = torch.from_numpy(X_train).float()
y_train = torch.from_numpy(y_train).long()
X_val = torch.from_numpy(X_val).float()
y_val = torch.from_numpy(y_val).long()
# Define the model
model = Net()
# Define the optimizer and loss function
optimizer = optim.Adam(model.parameters(), lr=0.01)
criterion = nn.CrossEntropyLoss()
# Train the model
train_acc = []
val_acc = []
for epoch in range(100):
# Train the model
model.train()
optimizer.zero_grad()
outputs = model(X_train)
loss = criterion(outputs, y_train)
loss.backward()
optimizer.step()
# Evaluate the model on training and validation sets
model.eval()
train_pred = model(X_train).argmax(dim=1)
train_accuracy = (train_pred == y_train).sum().item() / len(y_train)
val_pred = model(X_val).argmax(dim=1)
val_accuracy = (val_pred == y_val).sum().item() / len(y_val)
train_acc.append(train_accuracy)
val_acc.append(val_accuracy)
print(f"Epoch {epoch + 1}: Train accuracy: {train_accuracy:.4f}, Val accuracy: {val_accuracy:.4f}")
# Plot the accuracy curves
plt.plot(train_acc, label='Train')
plt.plot(val_acc, label='Validation')
plt.xlabel('Epoch')
plt.ylabel('Accuracy')
plt.legend()
plt.show()
```
这个示例代码将生成一个包含训练和验证准确性的曲线,并在训练完成后绘制。你可以自己修改代码来满足你的具体要求,比如加入噪声等。
阅读全文
相关推荐









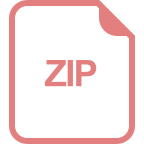
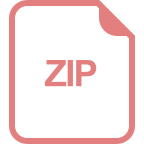
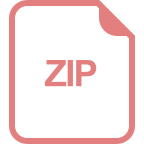
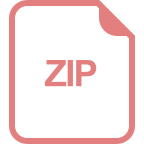