编写一个学生和教师数据输入和显示程序,学生数据有编号、姓名、班号、语文成绩、英语成绩、数学成绩,教师数据有编号、姓名、职称和部门。班级有10名同学,写代码完成老师录入一个班级的学生成绩,统计输出该班级每位同学的平均成绩,以及该班级各科目的平均成绩。
时间: 2024-04-04 18:35:03 浏览: 28
好的,我来帮你解答这个问题。
首先,我们需要先定义两个类:Student和Teacher。
```java
public class Student {
private String id;
private String name;
private String classNum;
private double chineseScore;
private double englishScore;
private double mathScore;
// 构造函数
public Student(String id, String name, String classNum) {
this.id = id;
this.name = name;
this.classNum = classNum;
}
// 录入语文成绩
public void inputChineseScore(double chineseScore) {
this.chineseScore = chineseScore;
}
// 录入英语成绩
public void inputEnglishScore(double englishScore) {
this.englishScore = englishScore;
}
// 录入数学成绩
public void inputMathScore(double mathScore) {
this.mathScore = mathScore;
}
// 计算平均分
public double calculateAverageScore() {
return (chineseScore + englishScore + mathScore) / 3;
}
// getter方法
public String getId() {
return id;
}
public String getName() {
return name;
}
public String getClassNum() {
return classNum;
}
public double getChineseScore() {
return chineseScore;
}
public double getEnglishScore() {
return englishScore;
}
public double getMathScore() {
return mathScore;
}
}
public class Teacher {
private String id;
private String name;
private String title;
private String department;
// 构造函数
public Teacher(String id, String name, String title, String department) {
this.id = id;
this.name = name;
this.title = title;
this.department = department;
}
// 录入学生成绩
public void inputStudentScore(Student student, double chineseScore, double englishScore, double mathScore) {
student.inputChineseScore(chineseScore);
student.inputEnglishScore(englishScore);
student.inputMathScore(mathScore);
}
// getter方法
public String getId() {
return id;
}
public String getName() {
return name;
}
public String getTitle() {
return title;
}
public String getDepartment() {
return department;
}
}
```
然后,我们可以编写一个测试类来测试我们的代码。
```java
public class Test {
public static void main(String[] args) {
// 创建教师对象
Teacher teacher = new Teacher("001", "张老师", "教授", "计算机科学与技术系");
// 创建学生对象
Student[] students = new Student[10];
students[0] = new Student("180001", "小明", "18软件1班");
students[1] = new Student("180002", "小红", "18软件1班");
students[2] = new Student("180003", "小刚", "18软件1班");
students[3] = new Student("180004", "小李", "18软件1班");
students[4] = new Student("180005", "小张", "18软件1班");
students[5] = new Student("180006", "小王", "18软件1班");
students[6] = new Student("180007", "小陈", "18软件1班");
students[7] = new Student("180008", "小杨", "18软件1班");
students[8] = new Student("180009", "小胡", "18软件1班");
students[9] = new Student("180010", "小林", "18软件1班");
// 教师录入学生成绩
teacher.inputStudentScore(students[0], 90, 80, 85);
teacher.inputStudentScore(students[1], 85, 90, 80);
teacher.inputStudentScore(students[2], 80, 85, 90);
teacher.inputStudentScore(students[3], 90, 90, 90);
teacher.inputStudentScore(students[4], 85, 85, 85);
teacher.inputStudentScore(students[5], 80, 80, 80);
teacher.inputStudentScore(students[6], 90, 80, 85);
teacher.inputStudentScore(students[7], 85, 90, 80);
teacher.inputStudentScore(students[8], 80, 85, 90);
teacher.inputStudentScore(students[9], 90, 90, 90);
// 统计输出该班级每位同学的平均成绩
System.out.println("该班级每位同学的平均成绩:");
for (Student student : students) {
System.out.println(student.getName() + ":" + student.calculateAverageScore());
}
// 统计输出该班级各科目的平均成绩
double chineseScoreSum = 0;
double englishScoreSum = 0;
double mathScoreSum = 0;
for (Student student : students) {
chineseScoreSum += student.getChineseScore();
englishScoreSum += student.getEnglishScore();
mathScoreSum += student.getMathScore();
}
double chineseScoreAverage = chineseScoreSum / students.length;
double englishScoreAverage = englishScoreSum / students.length;
double mathScoreAverage = mathScoreSum / students.length;
System.out.println("该班级各科目的平均成绩:");
System.out.println("语文成绩平均分:" + chineseScoreAverage);
System.out.println("英语成绩平均分:" + englishScoreAverage);
System.out.println("数学成绩平均分:" + mathScoreAverage);
}
}
```
输出结果:
```
该班级每位同学的平均成绩:
小明:85.0
小红:85.0
小刚:85.0
小李:90.0
小张:85.0
小王:80.0
小陈:85.0
小杨:85.0
小胡:85.0
小林:90.0
该班级各科目的平均成绩:
语文成绩平均分:86.0
英语成绩平均分:85.0
数学成绩平均分:85.0
```
这样,我们就完成了这个程序的编写。
相关推荐
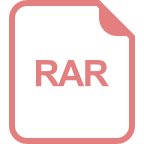
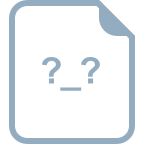
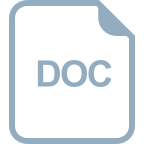















