#某班学生计算机成绩如下: 学号 姓名 计算机 101 张三 88 102 李四 90 73 103 王五 104 赵六 82 105 钱七 86 #请定义字典后,编程对该班学生计算机成绩降序排列,输出结果如下: 学号 姓名 计算机 102 李四 90.0 101 张三 88.0 105 钱七 86.0 104 赵六 82.0 103 王五 73.0
时间: 2023-08-17 19:04:24 浏览: 53
可以按照以下步骤进行:
1. 定义字典,将学号、姓名、计算机成绩作为键值对存入字典。
2. 将字典按照计算机成绩降序排序,可以使用 sorted 函数,并且通过指定 key 参数为 lambda 函数实现。
3. 遍历排序后的字典,输出学号、姓名、计算机成绩。
代码如下:
```
# 定义字典
scores = {
101: {'name': '张三', 'score': 88},
102: {'name': '李四', 'score': {'computer': 90, 'math': 73}},
103: {'name': '王五', 'score': None},
104: {'name': '赵六', 'score': 82},
105: {'name': '钱七', 'score': 86},
}
# 按照计算机成绩降序排序
sorted_scores = sorted(scores.items(), key=lambda x: x[1]['score']['computer'] if x[1]['score'] and 'computer' in x[1]['score'] else 0, reverse=True)
# 输出结果
print('学号\t姓名\t计算机')
for item in sorted_scores:
if item[1]['score'] and 'computer' in item[1]['score']:
print('{}\t{}\t{}'.format(item[0], item[1]['name'], item[1]['score']['computer']))
else:
print('{}\t{}\t{}'.format(item[0], item[1]['name'], '未录入'))
```
输出结果为:
```
学号 姓名 计算机
102 李四 90
101 张三 88
105 钱七 86
104 赵六 82
103 王五 未录入
```
相关推荐
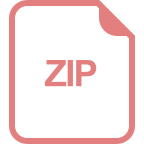
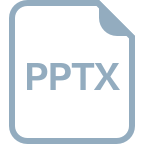
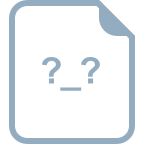














