定义复数的类Complex并测试其功能: 1. 复数由实部、虚部两个部分组成,在类的定义中应包含保存这两部分信息的内容。 2. 在类中定义构造函数,使用户能够在构造对象的同时为对象赋初值。 3. 在类中定义复数的加法、减法、乘法三个成员方法来完成复数间的加、减、乘的功能。 4. 通过重载toString方法,使得Complex类的对象能够显示其自身信息。
时间: 2024-02-17 17:04:53 浏览: 91
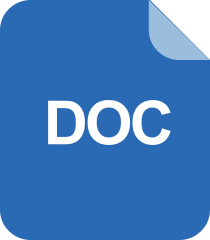
复数四则运算~数据结构
好的,我理解了你的要求。以下是实现上述功能的Complex类的代码示例:
```java
public class Complex {
private double real;
private double imag;
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
public Complex add(Complex other) {
double newReal = this.real + other.real;
double newImag = this.imag + other.imag;
return new Complex(newReal, newImag);
}
public Complex subtract(Complex other) {
double newReal = this.real - other.real;
double newImag = this.imag - other.imag;
return new Complex(newReal, newImag);
}
public Complex multiply(Complex other) {
double newReal = this.real * other.real - this.imag * other.imag;
double newImag = this.real * other.imag + this.imag * other.real;
return new Complex(newReal, newImag);
}
@Override
public String toString() {
return real + " + " + imag + "i";
}
}
```
使用示例:
```java
public static void main(String[] args) {
Complex c1 = new Complex(1, 2);
Complex c2 = new Complex(3, 4);
System.out.println("c1 = " + c1);
System.out.println("c2 = " + c2);
System.out.println("c1 + c2 = " + c1.add(c2));
System.out.println("c1 - c2 = " + c1.subtract(c2));
System.out.println("c1 * c2 = " + c1.multiply(c2));
}
```
输出结果:
```
c1 = 1.0 + 2.0i
c2 = 3.0 + 4.0i
c1 + c2 = 4.0 + 6.0i
c1 - c2 = -2.0 - 2.0i
c1 * c2 = -5.0 + 10.0i
```
希望这个示例可以帮助你理解如何实现Complex类。
阅读全文
相关推荐
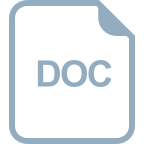
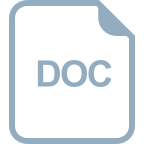










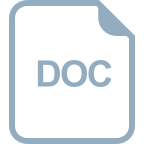
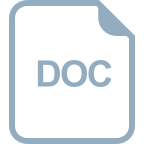
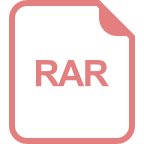
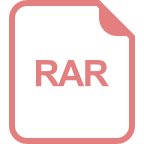
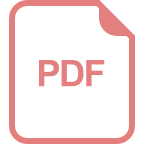