1. 定义复数(Complex)类 。 要求: (1)复数有实部和虚部属性; (2)定义类的构造方法; (3)实现复数加减法并输出复数属性的值。 (4)重载toString方法,用于对象属性值的输出。测试类
时间: 2024-02-18 09:00:08 浏览: 24
好的,以下是测试类的代码:
```java
public class ComplexTest {
public static void main(String[] args) {
Complex c1 = new Complex(1, 2);
Complex c2 = new Complex(3, 4);
Complex sum = c1.add(c2);
Complex diff = c1.subtract(c2);
System.out.println("c1 = " + c1);
System.out.println("c2 = " + c2);
System.out.println("sum = " + sum);
System.out.println("diff = " + diff);
}
}
```
你可以将这段代码保存为 ComplexTest.java 文件,然后在命令行中编译并运行它:
```
javac Complex.java ComplexTest.java
java ComplexTest
```
输出结果应该是:
```
c1 = 1.00 + 2.00i
c2 = 3.00 + 4.00i
sum = 4.00 + 6.00i
diff = -2.00 - 2.00i
```
相关问题
1. 定义复数(Complex)类 。 要求: (1)复数有实部和虚部属性; (2)定义类的构造方法; (3)实现复数加减法并输出复数属性的值。 (4)重载toString方法,用于对象属性值的输出。
好的,以下是一个简单的复数类的实现:
```java
public class Complex {
private double real; // 实部
private double imag; // 虚部
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
// 复数加法
public Complex add(Complex other) {
double newReal = this.real + other.real;
double newImag = this.imag + other.imag;
return new Complex(newReal, newImag);
}
// 复数减法
public Complex subtract(Complex other) {
double newReal = this.real - other.real;
double newImag = this.imag - other.imag;
return new Complex(newReal, newImag);
}
// 重载 toString 方法
@Override
public String toString() {
return String.format("%.2f + %.2fi", this.real, this.imag);
}
}
```
这个类有一个构造方法,用于初始化实部和虚部。它还有两个方法,用于实现复数的加减法。最后,它重载了 toString 方法,用于输出对象属性值。你可以通过下面的代码来测试这个类:
```java
public static void main(String[] args) {
Complex c1 = new Complex(1, 2);
Complex c2 = new Complex(3, 4);
Complex sum = c1.add(c2);
Complex diff = c1.subtract(c2);
System.out.println("c1 = " + c1);
System.out.println("c2 = " + c2);
System.out.println("sum = " + sum);
System.out.println("diff = " + diff);
}
```
输出结果应该是:
```
c1 = 1.00 + 2.00i
c2 = 3.00 + 4.00i
sum = 4.00 + 6.00i
diff = -2.00 - 2.00i
```
JAVA1.定义复数(Complex)类。要求:(1)复数有实部和虚部属性;(2)定义类的构造方法;(3)实现复数加减法并输出复数属性的值。(4)重载toString方法,用于对象属性值的输出。
下面是一个简单的Java实现,实现了复数类的定义、构造方法、复数加减法和toString方法的重载:
```java
public class Complex {
private double real; // 实部
private double imag; // 虚部
// 构造方法,初始化实部和虚部
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
// 复数加法
public Complex add(Complex other) {
double realSum = this.real + other.real;
double imagSum = this.imag + other.imag;
return new Complex(realSum, imagSum);
}
// 复数减法
public Complex subtract(Complex other) {
double realDiff = this.real - other.real;
double imagDiff = this.imag - other.imag;
return new Complex(realDiff, imagDiff);
}
// 重载toString方法,用于对象属性值的输出
public String toString() {
if (imag >= 0) {
return "(" + real + " + " + imag + "i)";
} else {
return "(" + real + " - " + (-imag) + "i)";
}
}
}
```
使用示例:
```java
public class Test {
public static void main(String[] args) {
Complex a = new Complex(1, 2);
Complex b = new Complex(2, -1);
Complex c = a.add(b);
Complex d = a.subtract(b);
System.out.println("a = " + a);
System.out.println("b = " + b);
System.out.println("a + b = " + c);
System.out.println("a - b = " + d);
}
}
```
输出结果:
```
a = (1.0 + 2.0i)
b = (2.0 - 1.0i)
a + b = (3.0 + 1.0i)
a - b = (-1.0 + 3.0i)
```
阅读全文
相关推荐
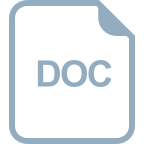
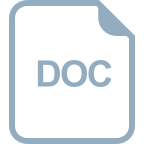
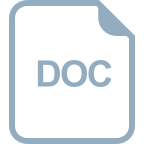









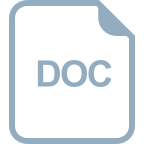
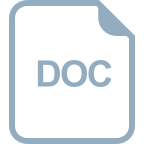
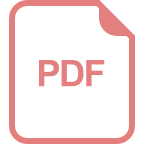
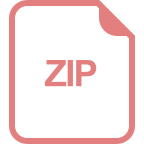