如何调用kmeans函数
时间: 2023-05-27 10:06:46 浏览: 80
在Python中使用sklearn库中的kmeans函数来进行调用。具体步骤如下:
1. 导入sklearn库中的kmeans函数:
```python
from sklearn.cluster import KMeans
```
2. 创建一个KMeans对象:
```python
kmeans = KMeans(n_clusters=k, init='k-means++', max_iter=300, n_init=10, random_state=0)
```
参数含义:
- n_clusters:簇的个数
- init:初始化的方法,k-means++表示使用更好的初始化方法
- max_iter:最大迭代次数
- n_init:重新运行k-means算法的次数,以避免陷入局部最小值
- random_state:用于随机初始化质心的随机种子
3. 使用.fit()方法进行拟合:
```python
kmeans.fit(data)
```
其中data是要进行聚类的数据。
4. 使用.predict()方法获取每个数据点所属的簇:
```python
labels = kmeans.predict(data)
```
其中labels是一个数组,每个元素表示对应数据点所属的簇的编号。
相关问题
在matlab中调用kmeans函数
要在Matlab中调用kmeans函数,可以按照以下步骤进行:
1. 打开Matlab软件并创建一个新的文件。
2. 在文件中输入以下命令以生成一组数据:
data = [randn(100,2)*0.75+ones(100,2);...
randn(100,2)*0.5-ones(100,2)];
3. 输入以下命令以调用kmeans函数:
[idx,C] = kmeans(data,2);
其中,data是要进行聚类的数据,2是要聚类的簇数。
4. 输入以下命令以绘制聚类结果:
figure;
plot(data(idx==1,1),data(idx==1,2),'r.','MarkerSize',12)
hold on
plot(data(idx==2,1),data(idx==2,2),'b.','MarkerSize',12)
plot(C(:,1),C(:,2),'kx','MarkerSize',15,'LineWidth',3)
legend('Cluster 1','Cluster 2','Centroids','Location','NW')
title 'Cluster Assignments and Centroids'
hold off
该命令将绘制聚类结果图,显示两个簇及其质心。
这就是在Matlab中调用kmeans函数的基本步骤。您可以根据需要自定义数据和参数。
python手写kmeans进行图片聚类(不调用kmeans函数)
K-Means 是一种经典的聚类算法,它常被用于图像分割和图像聚类。下面是一个 Python 手写 K-Means 算法实现的示例代码,用于对图片进行聚类。
```python
import numpy as np
from PIL import Image
def kmeans(img, K=3, max_iter=100):
# 将图像转换为向量形式
img_vec = img.reshape((-1, 3))
# 随机初始化聚类中心
centroids = img_vec[np.random.choice(len(img_vec), K, replace=False)]
for i in range(max_iter):
# 计算每个点到聚类中心的距离
distances = np.linalg.norm(img_vec[:, np.newaxis, :] - centroids, axis=2)
# 将每个点归类到距离最近的聚类中心
labels = np.argmin(distances, axis=1)
# 更新聚类中心
for j in range(K):
centroids[j] = np.mean(img_vec[labels == j], axis=0)
# 将每个点的颜色替换为所属聚类中心的颜色
img_vec[...] = centroids[labels]
return img_vec.reshape(img.shape)
# 读取图像
img = np.array(Image.open('test.jpg'))
# 调用 kmeans 函数进行聚类
clustered_img = kmeans(img)
# 显示聚类后的图像
Image.fromarray(clustered_img).show()
```
在上面的代码中,我们首先将图像转换为向量形式,然后随机初始化 K 个聚类中心。接着,我们执行 max_iter 次迭代,每次迭代都计算每个点到聚类中心的距离,并将每个点归类到距离最近的聚类中心。最后,我们根据每个点所属的聚类中心的颜色更新该点的颜色,并将其转换回图像形式。
需要注意的是,这个简单的 K-Means 算法实现可能存在局部最优解的问题,因此在实际应用中可能需要多次运行算法,或者使用改进的 K-Means 算法。
相关推荐
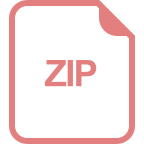
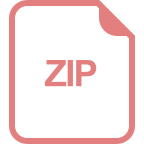












