7. 设计一个Java程序,实现在电脑D盘下创建my_java目录,在其目录下创建一个文件为HelloWorld.txt文件,判断他是文件还是目录,在my_java再创建一个目录IOTest,将HelloWorld.txt移动到IOTest目录下去,遍历并显示IOTest这个目录下的文件。
时间: 2024-03-24 20:37:07 浏览: 51
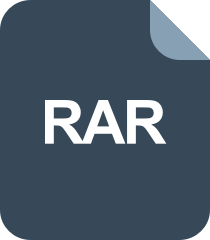
JAVA实现 根据所写文件的目录列出目录下的文件

好的,以下是实现上述要求的Java程序代码:
```java
import java.io.File;
import java.io.IOException;
public class IOExample {
public static void main(String[] args) {
// 在电脑D盘下创建my_java目录
File myJavaDir = new File("D:/my_java");
if (!myJavaDir.exists()) {
myJavaDir.mkdirs();
System.out.println("成功创建目录 D:/my_java");
} else {
System.out.println("目录 D:/my_java 已存在");
}
// 在my_java目录下创建HelloWorld.txt文件
File helloWorldFile = new File(myJavaDir, "HelloWorld.txt");
try {
if (helloWorldFile.createNewFile()) {
System.out.println("成功创建文件 " + helloWorldFile.getAbsolutePath());
} else {
System.out.println("文件 " + helloWorldFile.getAbsolutePath() + " 已存在");
}
} catch (IOException e) {
e.printStackTrace();
}
// 判断HelloWorld.txt是文件还是目录
if (helloWorldFile.isFile()) {
System.out.println(helloWorldFile.getName() + " 是文件");
} else if (helloWorldFile.isDirectory()) {
System.out.println(helloWorldFile.getName() + " 是目录");
}
// 在my_java目录下创建IOTest目录
File ioTestDir = new File(myJavaDir, "IOTest");
if (!ioTestDir.exists()) {
ioTestDir.mkdirs();
System.out.println("成功创建目录 " + ioTestDir.getAbsolutePath());
} else {
System.out.println("目录 " + ioTestDir.getAbsolutePath() + " 已存在");
}
// 将HelloWorld.txt移动到IOTest目录下
File newFile = new File(ioTestDir, helloWorldFile.getName());
if (helloWorldFile.renameTo(newFile)) {
System.out.println("成功将文件 " + helloWorldFile.getAbsolutePath() + " 移动到目录 " + ioTestDir.getAbsolutePath());
} else {
System.out.println("无法将文件 " + helloWorldFile.getAbsolutePath() + " 移动到目录 " + ioTestDir.getAbsolutePath());
}
// 遍历并显示IOTest目录下的文件
File[] files = ioTestDir.listFiles();
if (files != null) {
System.out.println("目录 " + ioTestDir.getAbsolutePath() + " 下的文件列表:");
for (File file : files) {
System.out.println("- " + file.getName());
}
}
}
}
```
上述代码会在电脑D盘下创建一个名为 `my_java` 的目录,在该目录下创建一个名为 `HelloWorld.txt` 的文件,并将其移动到名为 `IOTest` 的子目录下。最后,程序会遍历并显示 `IOTest` 目录下的所有文件。
阅读全文
相关推荐
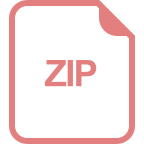
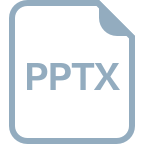
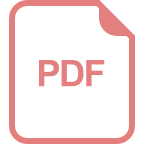
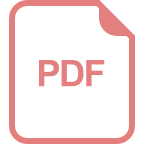
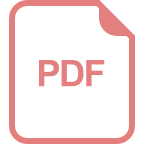
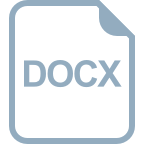
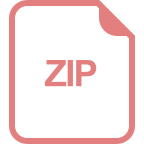
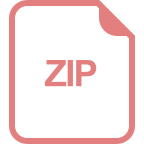
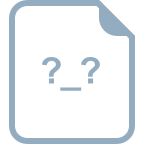
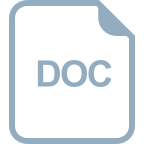
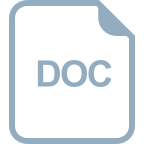
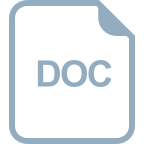
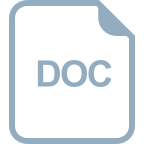
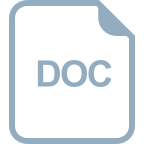
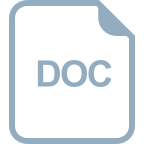