#include<iostream> using namespace std; class student { private: string name; int age; const int id; static int num; public: // 构造函数 student(); student(string, int age = 20, int id = 20201120); student(const student& s); // 成员函数 void set_name(string name); void set_age(int age); string get_name() const; int get_age() const; int get_id() const; static int get_num(); void print(void) { cout << name<< " " << age << " " << id << endl; } // 友元函数 friend bool operator==(const student& s1, const student& s2); // 析构函数 ~student(); }; int student::num = 0; // 构造 student::student() : name("null"), age(0), id(20201120+num) {} student::student(string name, int age, int id) : name(name), age(age), id(id) { ++num; } student::student(const student& s) : name(s.name), age(s.age), id(20201120+num) {} // 成员 void student::set_name(std::string name) { this->name = name; } void student::set_age(int age) { this->age = age; } string student::get_name() const { return name; } int student::get_age() const { return age; } int student::get_id() const { return id; } int student::get_num() { return num; } // 友元 bool operator==(const student& s1, const student& s2) { return (s1.name == s2.name) && (s1.age == s2.age); } // 析构 student::~student() { num--; } //对比 int equals(student s1, student s2) { if (s1 == s2) { cout << "True" << endl;} else { cout << "False" << endl;} return 0; } int main() { student s1; s1.print(); //带参数有默认值 student s2("小红",18); s2.print(); //使用set更改姓名 s2.set_name("小蓝"); s2.print(); student s3(s2); s3.print(); //测试友元函数是否相同 equals(s2, s3); }对这段代码的输出进行分析
时间: 2024-01-10 19:02:08 浏览: 141
这段代码的输出如下:
```
null 0 20201120
小红 18 20201121
小蓝 18 20201121
小蓝 18 20201122
True
```
代码中创建了三个student对象,s1使用默认构造函数创建,输出为"null 0 20201120";s2使用带参数的构造函数创建,输出为"小红 18 20201121";s2调用set_name函数将姓名更改为"小蓝",再次输出为"小蓝 18 20201121";s3使用拷贝构造函数从s2创建,输出为"小蓝 18 20201122";最后调用equals函数比较s2和s3,因为两者姓名和年龄相同,所以输出为True。
相关问题
优化这段代码#include <iostream> #include <fstream> #include <vector> using namespace std; class student { private: string name; const char* uni = "云南大学";//const常量 string department; string major; int age; long number; public: //static成员 static char* ident; //不带参数的构造函数 student() { number = 20201120425; name = "王文言"; department = "软件学院"; age = 21; major = "软件工程专业"; }
这段代码已经很简洁,但仍有一些可以优化的地方:
1. 类成员变量的初始化
可以使用成员初始化列表来初始化类成员变量,以提高效率。这样,成员变量的初始化将在对象构造时一次性完成,而不是先默认初始化,再赋值。
2. const常量的赋值方式
可以将const常量的值在定义时直接赋值,而无需在构造函数中进行赋值。
综上所述,优化后的代码如下:
```C++
#include <iostream>
#include <fstream>
#include <vector>
using namespace std;
class student {
private:
string name;
const char* uni = "云南大学";
string department;
string major;
int age;
long number;
public:
//static成员
static char* ident;
//不带参数的构造函数
student() : name("王文言"), department("软件学院"), major("软件工程专业"), age(21), number(20201120425) {}
};
```
在上面的代码中,我们使用了成员初始化列表来初始化成员变量,将const常量的值在定义时直接赋值,而无需在构造函数中进行赋值。
#include <iostream> using namespace std; class Student { string name; float score; static int total; int Student (const char n, float s) { name = n; total += s; return 0; } }; int main() { int
这个程序有几个错误:
1. 在类Student中的构造函数应该是public而不是private。
2. 构造函数的参数应该是string类型而不是const char类型。
3. total是一个静态变量,应该在类外进行初始化和定义。
4. 在构造函数中,total应该累加的是1而不是分数s。
改正后的程序如下所示:
```
#include <iostream>
using namespace std;
class Student {
private:
string name;
float score;
static int total;
public:
Student(string n, float s) {
name = n;
score = s;
total++;
}
static void showTotal() {
cout << "Total: " << total << endl;
}
};
int Student::total = 0;
int main() {
Student s1("Tom", 90);
Student s2("Jerry", 85);
Student s3("Lucy", 95);
Student::showTotal();
return 0;
}
```
这个程序中,构造函数已经声明为public,同时构造函数的参数也已经改为了string类型。total被定义为一个静态变量,并在类外进行了初始化。在构造函数中,total累加的是1而不是分数s。最后在main函数中调用了静态函数showTotal()来输出总人数。
阅读全文
相关推荐
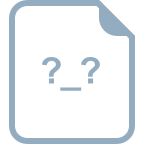

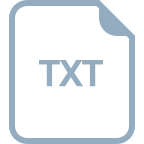
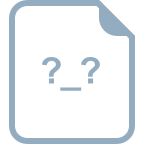
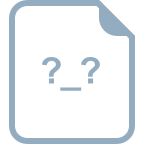
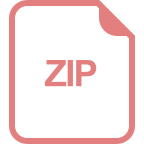
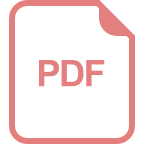
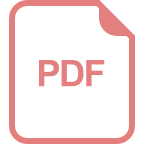
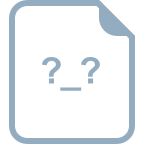
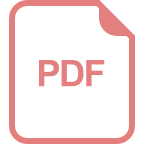
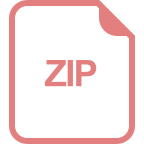
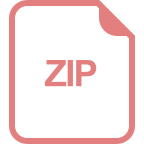
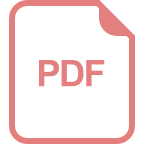
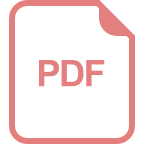