从键盘输入两个字符串,找出两个字符串中相同的字符,并按升序输出。
时间: 2024-12-13 15:28:45 浏览: 8
当然,我们可以使用C语言来编写一个程序,该程序首先读取两个字符串,然后遍历第一个字符串中的每个字符,检查这个字符是否也存在于第二个字符串中。如果存在,就将其添加到结果数组中。最后,我们对结果数组进行排序并输出。
以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h> // 包含qsort函数
// 定义比较函数,用于排序字符
int compare(const void *a, const void *b) {
return (*(char*)a - *(char*)b);
}
void find_common_chars(char str1[], char str2[]) {
int len1 = strlen(str1);
int len2 = strlen(str2);
int common_len = min(len1, len2); // 找到较短字符串长度
char common_chars[common_len]; // 存储相同字符的数组
int index = 0;
for (int i = 0; i < common_len; i++) {
if (str1[i] == str2[i]) {
common_chars[index++] = str1[i];
}
}
// 对相同字符数组进行排序
qsort(common_chars, index, sizeof(char), compare);
printf("Common characters in ascending order: ");
for (int i = 0; i < index; i++) {
printf("%c ", common_chars[i]);
}
printf("\n");
}
int main() {
char str1[100], str2[100];
printf("Enter first string: ");
fgets(str1, sizeof(str1), stdin); // 避免换行符
str1[strlen(str1)-1] = '\0'; // 去除末尾的换行符
printf("Enter second string: ");
fgets(str2, sizeof(str2), stdin);
str2[strlen(str2)-1] = '\0';
find_common_chars(str1, str2);
return 0;
}
```
运行这段代码后,按照提示输入两个字符串,程序会找出并按升序输出它们共有的字符。
阅读全文
相关推荐
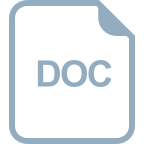
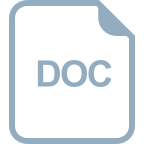
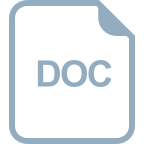



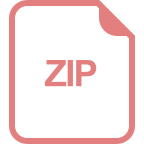
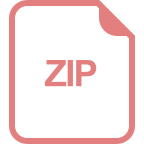
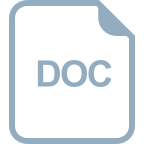
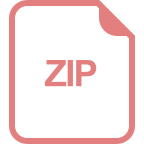



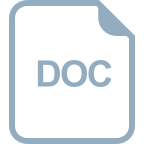
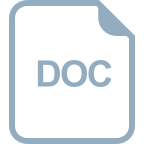
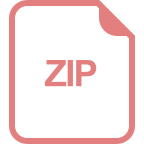