vue3中在当前A页面如何修改非当前B页面data中的值?
时间: 2024-09-30 21:09:23 浏览: 12
在Vue3中,由于组件之间的数据独立,通常无法直接从一个页面(A组件)修改另一个页面(B组件)的数据。这是为了防止意外的副作用并且保持组件间的封装性。如果你需要在A页面影响B页面的数据,你可以通过以下几种方式:
1. **使用vuex**:Vuex是一个专为Vue.js应用程序开发的状态管理模式。你可以将共享状态存储在store中,然后A页面触发action更新store,B页面监听这个状态变化。
```javascript
// store.js
import { createStore } from 'vuex'
...
const state = {
valueForB: ''
}
...
actions: {
updateValue({ commit }, newValue) {
commit('updateValueInB', newValue)
}
}
// A.vue
this.$store.dispatch('updateValue', 'new value')
// B.vue
export default {
computed: {
valueFromA() {
return this.$store.state.valueForB;
}
},
watch: {
'$store.state.valueForB': 'onValueChange'
},
methods: {
onValueChange(newValue) {
// 新值已改变,这里处理相关逻辑
}
}
}
```
2. **使用事件总线**:创建一个全局事件中心,让A页面发布事件,B页面订阅并处理这个事件。
```javascript
// eventBus.js
import Vue from 'vue'
const bus = new Vue()
// A.vue
bus.$emit('update-value-in-b', 'new value')
// B.vue
export default {
mounted() {
bus.$on('update-value-in-b', newValue => {
// 更新B页面数据
})
},
beforeDestroy() {
bus.$off('update-value-in-b')
}
}
```
这两种方式都实现了组件间的数据通信,但都需要明确的设计和管理,以避免混乱和不必要的复杂性。
相关推荐
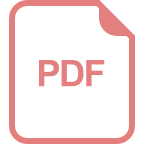
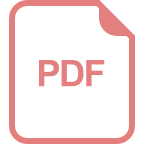
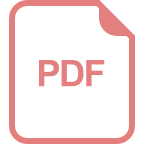














