如何对Pandas中的DataFrame进行数据筛选?
时间: 2024-09-09 07:08:10 浏览: 52
Pandas中的DataFrame是一个二维标签数据结构,你可以使用多种方法进行数据筛选。以下是几种常见的筛选方法:
1. 使用`loc`和`iloc`:
- `loc`用于基于标签的索引,它可以根据行标签和列标签来进行数据筛选。
```python
df.loc[row_indexer, column_indexer] # 行标签和列标签
```
- `iloc`用于基于整数的索引,它通过行号和列号来选择数据。
```python
df.iloc[row_indexer, column_indexer] # 行号和列号
```
2. 使用布尔索引:
- 布尔索引是一种非常灵活的筛选方式,你可以创建一个布尔数组来表示每一行是否满足特定条件。
```python
df[df['column_name'] > some_value] # 根据列值筛选满足条件的行
```
3. 使用条件组合:
- Pandas支持使用`&`(和)和`|`(或)运算符来组合多个条件。
```python
df[(df['column1'] > value1) & (df['column2'] < value2)] # 多个条件组合筛选
```
4. 使用`.query()`方法:
- 这是一种简洁的筛选方法,允许你使用字符串形式的查询表达式来进行筛选。
```python
df.query('column1 > @value1 and column2 < @value2') # 使用字符串形式的查询表达式
```
5. 使用`.drop()`和`.keep()`方法:
- 你可以使用`drop`方法根据标签删除某些行或列,而`keep`方法则用于指定保留哪些行或列。
```python
df.drop(labels, axis=0, inplace=False) # 删除行
df.drop(labels, axis=1, inplace=False) # 删除列
df.keep(labels, axis=0, inplace=False) # 保留行
df.keep(labels, axis=1, inplace=False) # 保留列
```
6. 使用切片:
- 类似于Python列表,你也可以对DataFrame进行切片操作,这会返回部分数据的视图。
```python
df[start:stop:step] # 行切片
df[column_start:column_stop:column_step] # 列切片
```
阅读全文
相关推荐
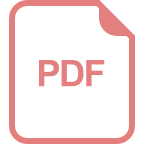
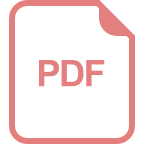
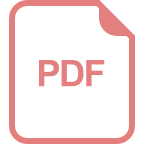
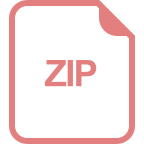
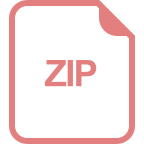
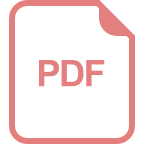
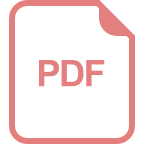
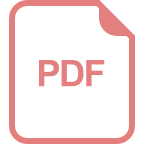
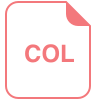








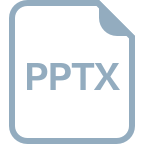