循环队列python
时间: 2023-11-06 16:08:01 浏览: 85
好的,关于循环队列的实现,可以使用Python中的列表来实现。具体实现方法如下:
```python
class CircularQueue:
def __init__(self, k: int):
self.k = k
self.queue = [None] * k
self.head = -1
self.tail = -1
def enqueue(self, value: int) -> bool:
if self.isFull():
return False
if self.isEmpty():
self.head = 0
self.tail = (self.tail + 1) % self.k
self.queue[self.tail] = value
return True
def dequeue(self) -> bool:
if self.isEmpty():
return False
if self.head == self.tail:
self.head, self.tail = -1, -1
return True
self.head = (self.head + 1) % self.k
return True
def Front(self) -> int:
if self.isEmpty():
return -1
return self.queue[self.head]
def Rear(self) -> int:
if self.isEmpty():
return -1
return self.queue[self.tail]
def isEmpty(self) -> bool:
return self.head == -1
def isFull(self) -> bool:
return (self.tail + 1) % self.k == self.head
```
其中,`k`表示队列的容量,`queue`是一个长度为`k`的列表,`head`和`tail`分别表示队列的头和尾。具体实现方法如下:
- `enqueue(value)`:入队操作,如果队列已满,则返回`False`;否则,将元素插入到队尾,并更新`tail`指针。
- `dequeue()`:出队操作,如果队列为空,则返回`False`;否则,将队头元素出队,并更新`head`指针。
- `Front()`:获取队头元素,如果队列为空,则返回`-1`。
- `Rear()`:获取队尾元素,如果队列为空,则返回`-1`。
- `isEmpty()`:判断队列是否为空。
- `isFull()`:判断队列是否已满。
阅读全文
相关推荐
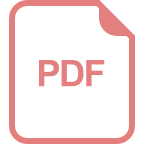



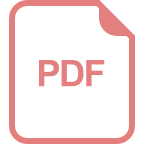











