python循环队列list
时间: 2023-12-16 17:26:59 浏览: 29
循环队列是一种特殊的队列,它可以在固定大小的空间内实现队列的基本操作。在Python中,我们可以使用list来实现循环队列。下面是一个使用list实现循环队列的例子:
```python
class MyCircularQueue:
def __init__(self, k: int):
"""
Initialize your data structure here. Set the size of the queue to be k.
"""
self.queue = [0] * k
self.head = 0
self.tail = 0
self.max_size = k
def enQueue(self, value: int) -> bool:
"""
Insert an element into the circular queue. Return true if the operation is successful.
"""
if self.isFull():
return False
self.queue[self.tail] = value
self.tail = (self.tail + 1) % self.max_size
return True
def deQueue(self) -> bool:
"""
Delete an element from the circular queue. Return true if the operation is successful.
"""
if self.isEmpty():
return False
self.head = (self.head + 1) % self.max_size
return True
def Front(self) -> int:
"""
Get the front item from the queue.
"""
if self.isEmpty():
return -1
return self.queue[self.head]
def Rear(self) -> int:
"""
Get the last item from the queue.
"""
if self.isEmpty():
return -1
return self.queue[(self.tail - 1 + self.max_size) % self.max_size]
def isEmpty(self) -> bool:
"""
Checks whether the circular queue is empty or not.
"""
return self.head == self.tail
def isFull(self) -> bool:
"""
Checks whether the circular queue is full or not.
"""
return (self.tail + 1) % self.max_size == self.head
```
在这个例子中,我们使用了一个list来存储队列元素,同时使用head和tail两个指针来指示队列的头和尾。当队列满时,我们可以通过tail指针的位置来判断队列是否已满。当队列为空时,我们可以通过head和tail指针的位置来判断队列是否为空。在入队和出队操作中,我们需要更新head和tail指针的位置,并且需要使用取模运算来实现循环队列的特性。
相关推荐
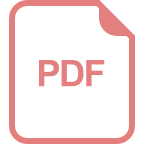
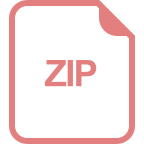
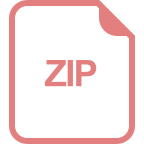














