c++, 通过键值访问元素
时间: 2024-09-12 19:14:26 浏览: 34
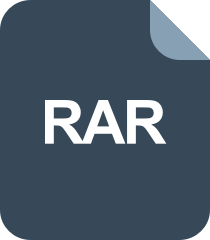
C++解析json字符串
C++中通过键值访问元素通常涉及到使用关联容器,最常见的是`std::map`和`std::unordered_map`。`std::map`是基于红黑树实现的,而`std::unordered_map`是基于哈希表实现的。它们都提供了通过键值(key)快速访问元素的能力。
下面是使用`std::map`和`std::unordered_map`的基本示例:
```cpp
#include <iostream>
#include <map>
#include <unordered_map>
int main() {
// 使用std::map
std::map<std::string, int> myMap;
myMap["apple"] = 5; // 插入键值对
myMap["banana"] = 3;
// 通过键值访问元素
int appleCount = myMap["apple"]; // 直接通过键访问对应的值
std::cout << "apple count: " << appleCount << std::endl;
// 使用std::unordered_map
std::unordered_map<std::string, int> myUnorderedMap;
myUnorderedMap["apple"] = 5; // 插入键值对
myUnorderedMap["banana"] = 3;
// 通过键值访问元素
int bananaCount = myUnorderedMap["banana"]; // 直接通过键访问对应的值
std::cout << "banana count: " << bananaCount << std::endl;
return 0;
}
```
需要注意的是,`std::map`由于其内部结构,访问元素的时间复杂度是O(log n),而`std::unordered_map`的时间复杂度是平均情况下O(1)。
阅读全文
相关推荐
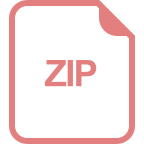
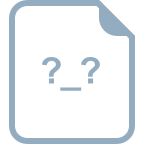


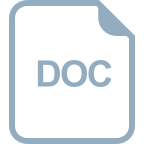
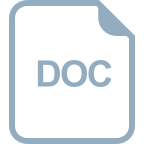
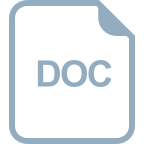
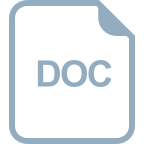
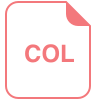
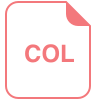







