unordered_map如何通过键值对 由值获得键
时间: 2024-03-24 10:34:57 浏览: 57
unordered_map是C++标准库中的一个关联容器,它提供了一种通过键值对来存储和访问元素的方式。在unordered_map中,每个元素都是一个键值对,其中键是唯一的,而值可以重复。
要通过值获取键,可以使用unordered_map的迭代器进行遍历,找到对应的键。具体步骤如下:
1. 遍历unordered_map中的每个键值对,可以使用auto关键字来自动推导迭代器类型。
2. 使用迭代器的second成员来访问值,判断是否与目标值相等。
3. 如果相等,则使用迭代器的first成员来获取对应的键。
下面是一个示例代码:
```cpp
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, std::string> myMap;
myMap[1] = "apple";
myMap[2] = "banana";
myMap[3] = "orange";
std::string targetValue = "banana";
int targetKey = -1;
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
if (it->second == targetValue) {
targetKey = it->first;
break;
}
}
if (targetKey != -1) {
std::cout << "The key corresponding to the value \"" << targetValue << "\" is: " << targetKey << std::endl;
} else {
std::cout << "The value \"" << targetValue << "\" does not exist in the unordered_map." << std::endl;
}
return 0;
}
```
输出结果为:
```
The key corresponding to the value "banana" is: 2
```
阅读全文
相关推荐
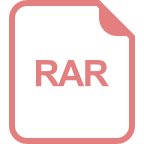
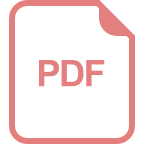

















