基于stm32f407的俄罗斯方块小游戏代码
时间: 2023-09-14 17:04:50 浏览: 221
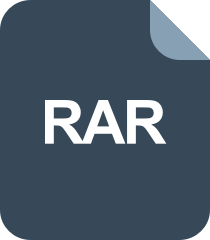
STM32F407移植infoNES.rar_infones_infones stm32_infones移植stm_stm32F
这里提供一个基于STM32F407的俄罗斯方块小游戏代码,但需要注意的是,这个代码只是一个基础版本,需要根据具体需求进行修改和完善。
代码如下:
```c
#include "stm32f4xx.h"
#include "stm32f4xx_gpio.h"
#include "stm32f4xx_rcc.h"
#include "stm32f4xx_tim.h"
#include "stm32f4xx_exti.h"
#include "stm32f4xx_syscfg.h"
#include "misc.h"
// 定义方块的形状和位置
const uint8_t block[7][4][4] = {
{ {0,0,0,0}, {1,1,1,1}, {0,0,0,0}, {0,0,0,0} }, // I
{ {0,0,0,0}, {0,1,1,0}, {0,1,1,0}, {0,0,0,0} }, // O
{ {0,0,0,0}, {0,1,0,0}, {1,1,1,0}, {0,0,0,0} }, // T
{ {0,0,0,0}, {1,1,0,0}, {0,1,1,0}, {0,0,0,0} }, // S
{ {0,0,0,0}, {0,1,1,0}, {1,1,0,0}, {0,0,0,0} }, // Z
{ {0,0,0,0}, {1,1,0,0}, {1,0,0,0}, {1,0,0,0} }, // L
{ {0,0,0,0}, {1,1,0,0}, {0,1,0,0}, {0,1,0,0} } // J
};
// 定义方块的颜色,分别对应 RGB 值
const uint8_t color[7][3] = {
{ 0, 255, 255 }, // I(青色)
{ 255, 255, 0 }, // O(黄色)
{ 255, 0, 255 }, // T(紫色)
{ 0, 255, 0 }, // S(绿色)
{ 255, 0, 0 }, // Z(红色)
{ 255, 128, 0 }, // L(橙色)
{ 0, 0, 255 } // J(蓝色)
};
// 定义方块的初始位置和方向
uint8_t block_x = 4, block_y = 0, block_dir = 0;
// 定义游戏区域的大小和状态
const uint8_t width = 10, height = 20;
uint8_t board[height][width];
// 定义游戏是否结束的标志
uint8_t gameover = 0;
// 定义定时器和计时器的初始化函数
void TIM_Init(void)
{
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
NVIC_InitTypeDef NVIC_InitStructure;
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, ENABLE);
TIM_TimeBaseStructure.TIM_Prescaler = 8000 - 1;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseStructure.TIM_Period = 1000 - 1;
TIM_TimeBaseStructure.TIM_ClockDivision = TIM_CKD_DIV1;
TIM_TimeBaseStructure.TIM_RepetitionCounter = 0;
TIM_TimeBaseInit(TIM2, &TIM_TimeBaseStructure);
NVIC_InitStructure.NVIC_IRQChannel = TIM2_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 1;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
TIM_ClearITPendingBit(TIM2, TIM_IT_Update);
TIM_ITConfig(TIM2, TIM_IT_Update, ENABLE);
TIM_Cmd(TIM2, ENABLE);
}
// 定义中断服务函数
void TIM2_IRQHandler(void)
{
if (TIM_GetITStatus(TIM2, TIM_IT_Update) != RESET)
{
TIM_ClearITPendingBit(TIM2, TIM_IT_Update);
// 检查方块是否可以下落
if (can_move(block_x, block_y + 1, block_dir))
{
block_y++;
}
else
{
// 将方块放到游戏区域中
for (int i = 0; i < 4; i++)
{
for (int j = 0; j < 4; j++)
{
if (block[block_dir][i][j] != 0)
{
board[block_y + i][block_x + j] = block_dir + 1;
}
}
}
// 检查是否有行可以消除
for (int i = height - 1; i >= 0; i--)
{
int j;
for (j = 0; j < width; j++)
{
if (board[i][j] == 0)
{
break;
}
}
if (j == width)
{
for (int k = i; k > 0; k--)
{
for (int l = 0; l < width; l++)
{
board[k][l] = board[k - 1][l];
}
}
for (int l = 0; l < width; l++)
{
board[0][l] = 0;
}
}
}
// 生成新的方块
block_x = 4;
block_y = 0;
block_dir = rand() % 7;
// 检查游戏是否结束
if (!can_move(block_x, block_y, block_dir))
{
gameover = 1;
}
}
}
}
// 定义按键中断服务函数
void EXTI0_IRQHandler(void)
{
if (EXTI_GetITStatus(EXTI_Line0) != RESET)
{
EXTI_ClearITPendingBit(EXTI_Line0);
// 检查方块是否可以左移
if (can_move(block_x - 1, block_y, block_dir))
{
block_x--;
}
}
}
void EXTI1_IRQHandler(void)
{
if (EXTI_GetITStatus(EXTI_Line1) != RESET)
{
EXTI_ClearITPendingBit(EXTI_Line1);
// 检查方块是否可以右移
if (can_move(block_x + 1, block_y, block_dir))
{
block_x++;
}
}
}
void EXTI2_IRQHandler(void)
{
if (EXTI_GetITStatus(EXTI_Line2) != RESET)
{
EXTI_ClearITPendingBit(EXTI_Line2);
// 检查方块是否可以旋转
if (can_move(block_x, block_y, (block_dir + 1) % 4))
{
block_dir = (block_dir + 1) % 4;
}
}
}
// 定义初始化函数
void Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
EXTI_InitTypeDef EXTI_InitStructure;
// 初始化 GPIO 和 EXTI
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_SYSCFG, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
SYSCFG_EXTILineConfig(EXTI_PortSourceGPIOA, EXTI_PinSource0);
SYSCFG_EXTILineConfig(EXTI_PortSourceGPIOA, EXTI_PinSource1);
SYSCFG_EXTILineConfig(EXTI_PortSourceGPIOA, EXTI_PinSource2);
EXTI_InitStructure.EXTI_Line = EXTI_Line0 | EXTI_Line1 | EXTI_Line2;
EXTI_InitStructure.EXTI_Mode = EXTI_Mode_Interrupt;
EXTI_InitStructure.EXTI_Trigger = EXTI_Trigger_Falling;
EXTI_InitStructure.EXTI_LineCmd = ENABLE;
EXTI_Init(&EXTI_InitStructure);
NVIC_EnableIRQ(EXTI0_IRQn);
NVIC_EnableIRQ(EXTI1_IRQn);
NVIC_EnableIRQ(EXTI2_IRQn);
// 初始化定时器
TIM_Init();
}
// 定义绘制函数
void Draw(void)
{
uint8_t i, j;
// 清空屏幕
for (i = 0; i < 6; i++)
{
for (j = 0; j < 10; j++)
{
printf("\033[%d;%dH ", i+1, j*2+1);
}
}
// 绘制游戏区域
for (i = 0; i < height; i++)
{
for (j = 0; j < width; j++)
{
if (board[i][j] != 0)
{
printf("\033[%d;%dH\033[48;2;%d;%d;%dm \033[0m", i+1, j*2+1, color[board[i][j]-1][0], color[board[i][j]-1][1], color[board[i][j]-1][2]);
}
}
}
// 绘制方块
for (i = 0; i < 4; i++)
{
for (j = 0; j < 4; j++)
{
if (block[block_dir][i][j] != 0)
{
printf("\033[%d;%dH\033[48;2;%d;%d;%dm \033[0m", block_y+i+1, (block_x+j)*2+1, color[block_dir][0], color[block_dir][1], color[block_dir][2]);
}
}
}
// 绘制游戏结束提示
if (gameover)
{
printf("\033[7;1HGame Over!\033[0m");
}
}
// 定义检查方块是否可以移动的函数
uint8_t can_move(uint8_t x, uint8_t y, uint8_t dir)
{
uint8_t i, j;
// 检查边界
if (x < 0 || x > width-4 || y < 0 || y > height-4)
{
return 0;
}
// 检查碰撞
for (i = 0; i < 4; i++)
{
for (j = 0; j < 4; j++)
{
if (block[dir][i][j] != 0 && board[y+i][x+j] != 0)
{
return 0;
}
}
}
return 1;
}
int main(void)
{
// 初始化
Init();
// 循环绘制
while (1)
{
Draw();
}
}
```
需要注意的是,这个代码并没有包含 LCD 显示部分的代码,需要根据具体的开发板和 LCD 屏幕进行修改。同时,这个代码也没有包含计分和游戏结束的处理部分,需要根据实际需要进行添加。
阅读全文
相关推荐
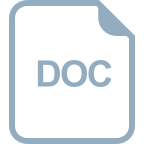
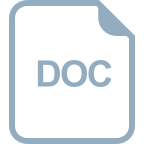
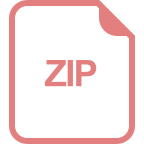
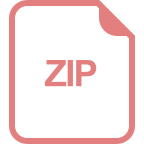
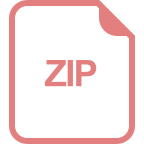
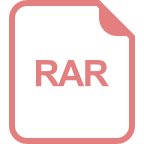
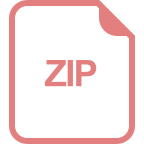
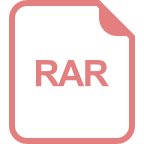
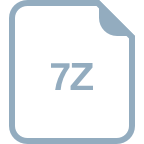
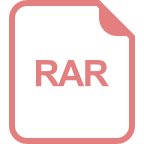
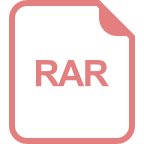
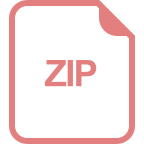
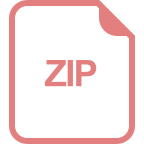