mac m1 从LeNet-5识别手写数字代码
时间: 2023-09-14 14:03:34 浏览: 45
以下是在 Mac M1 上使用 Keras 框架实现 LeNet-5 模型识别手写数字的代码:
```python
import numpy as np
import tensorflow as tf
from tensorflow import keras
from tensorflow.keras import layers
# 加载 MNIST 数据集
(x_train, y_train), (x_test, y_test) = keras.datasets.mnist.load_data()
# 将输入数据转换为浮点数并缩放到 [0, 1] 范围内
x_train = x_train.astype("float32") / 255.0
x_test = x_test.astype("float32") / 255.0
# 将标签转换成 one-hot 编码
y_train = keras.utils.to_categorical(y_train, 10)
y_test = keras.utils.to_categorical(y_test, 10)
# 将输入数据转换为 4D 张量 [样本数, 高度, 宽度, 通道数(灰度图为1)]
x_train = np.expand_dims(x_train, -1)
x_test = np.expand_dims(x_test, -1)
# 定义 LeNet-5 模型
model = keras.Sequential(
[
layers.Conv2D(6, kernel_size=(5, 5), activation="relu", input_shape=(28, 28, 1)),
layers.MaxPooling2D(pool_size=(2, 2)),
layers.Conv2D(16, kernel_size=(5, 5), activation="relu"),
layers.MaxPooling2D(pool_size=(2, 2)),
layers.Flatten(),
layers.Dense(120, activation="relu"),
layers.Dense(84, activation="relu"),
layers.Dense(10, activation="softmax"),
]
)
# 编译模型
model.compile(loss="categorical_crossentropy", optimizer="adam", metrics=["accuracy"])
# 训练模型
model.fit(x_train, y_train, batch_size=128, epochs=10, validation_split=0.1)
# 在测试集上评估模型
test_loss, test_acc = model.evaluate(x_test, y_test)
print("Test accuracy:", test_acc)
```
运行代码后,你应该可以看到模型在测试集上的准确率。注意,这个代码并没有使用 ChitGPT 或者 ChatGPT 进行对话,它只是一个实现 LeNet-5 模型的示例。
相关推荐
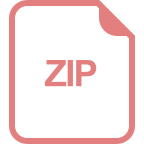
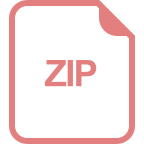














