用java设计图书管理系统,且满足以下要求: 1系统主控平台 2用户管理楧块 3借阅管理模块 4 书库管理模块 5读者管理模块,并给出代码
时间: 2024-03-24 17:39:09 浏览: 21
好的,以下是一个简单的Java图书管理系统的代码示例,实现了系统主控平台、用户管理模块、借阅管理模块、书库管理模块和读者管理模块:
```java
import java.util.ArrayList;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// 初始化图书馆
Library library = new Library();
// 初始化用户
Admin admin = new Admin("admin", "123456");
library.addUser(admin);
// 登录界面
System.out.println("欢迎使用图书馆管理系统!");
Scanner scanner = new Scanner(System.in);
User user = null;
while (user == null) {
System.out.print("请输入用户名:");
String username = scanner.nextLine();
System.out.print("请输入密码:");
String password = scanner.nextLine();
user = library.login(username, password);
if (user == null) {
System.out.println("用户名或密码错误,请重新输入!");
}
}
// 主菜单
while (true) {
System.out.println("欢迎使用图书馆管理系统," + user.getUsername() + "!");
System.out.println("1.借书");
System.out.println("2.还书");
System.out.println("3.添加图书");
System.out.println("4.删除图书");
System.out.println("5.修改图书");
System.out.println("6.添加读者");
System.out.println("7.删除读者");
System.out.println("8.修改读者");
System.out.println("9.退出");
System.out.print("请输入选项:");
int choice = scanner.nextInt();
scanner.nextLine();
switch (choice) {
case 1:
// 借书
System.out.print("请输入书名:");
String bookName = scanner.nextLine();
System.out.print("请输入读者姓名:");
String readerName = scanner.nextLine();
Book book = library.searchBook(bookName);
if (book == null) {
System.out.println("图书馆中没有这本书!");
} else {
Reader reader = library.searchReader(readerName);
if (reader == null) {
System.out.println("没有找到这位读者!");
} else {
if (library.borrowBook(book, reader)) {
System.out.println("借书成功!");
} else {
System.out.println("借书失败!");
}
}
}
break;
case 2:
// 还书
System.out.print("请输入书名:");
String bookName2 = scanner.nextLine();
System.out.print("请输入读者姓名:");
String readerName2 = scanner.nextLine();
Book book2 = library.searchBook(bookName2);
if (book2 == null) {
System.out.println("图书馆中没有这本书!");
} else {
Reader reader2 = library.searchReader(readerName2);
if (reader2 == null) {
System.out.println("没有找到这位读者!");
} else {
if (library.returnBook(book2, reader2)) {
System.out.println("还书成功!");
} else {
System.out.println("还书失败!");
}
}
}
break;
case 3:
// 添加图书
System.out.print("请输入书名:");
String bookName3 = scanner.nextLine();
System.out.print("请输入作者:");
String author = scanner.nextLine();
System.out.print("请输入出版社:");
String press = scanner.nextLine();
Book newBook = new Book(bookName3, author, press);
if (library.addBook(newBook)) {
System.out.println("添加图书成功!");
} else {
System.out.println("添加图书失败!");
}
break;
case 4:
// 删除图书
System.out.print("请输入书名:");
String bookName4 = scanner.nextLine();
if (library.deleteBook(bookName4)) {
System.out.println("删除图书成功!");
} else {
System.out.println("删除图书失败!");
}
break;
case 5:
// 修改图书
System.out.print("请输入书名:");
String bookName5 = scanner.nextLine();
Book book5 = library.searchBook(bookName5);
if (book5 == null) {
System.out.println("图书馆中没有这本书!");
} else {
System.out.print("请输入新的作者(原作者:" + book5.getAuthor() + "):");
String newAuthor = scanner.nextLine();
System.out.print("请输入新的出版社(原出版社:" + book5.getPress() + "):");
String newPress = scanner.nextLine();
Book newBook5 = new Book(book5.getName(), newAuthor, newPress);
if (library.modifyBook(book5, newBook5)) {
System.out.println("修改图书成功!");
} else {
System.out.println("修改图书失败!");
}
}
break;
case 6:
// 添加读者
System.out.print("请输入姓名:");
String readerName6 = scanner.nextLine();
System.out.print("请输入年龄:");
int age = scanner.nextInt();
scanner.nextLine();
Reader newReader = new Reader(readerName6, age);
if (library.addReader(newReader)) {
System.out.println("添加读者成功!");
} else {
System.out.println("添加读者失败!");
}
break;
case 7:
// 删除读者
System.out.print("请输入姓名:");
String readerName7 = scanner.nextLine();
if (library.deleteReader(readerName7)) {
System.out.println("删除读者成功!");
} else {
System.out.println("删除读者失败!");
}
break;
case 8:
// 修改读者
System.out.print("请输入姓名:");
String readerName8 = scanner.nextLine();
Reader reader8 = library.searchReader(readerName8);
if (reader8 == null) {
System.out.println("没有找到这位读者!");
} else {
System.out.print("请输入新的年龄(原年龄:" + reader8.getAge() + "):");
int newAge = scanner.nextInt();
scanner.nextLine();
Reader newReader8 = new Reader(reader8.getName(), newAge);
if (library.modifyReader(reader8, newReader8)) {
System.out.println("修改读者成功!");
} else {
System.out.println("修改读者失败!");
}
}
break;
case 9:
// 退出
System.out.println("感谢使用图书馆管理系统,再见!");
System.exit(0);
break;
default:
System.out.println("无效的选项,请重新输入!");
break;
}
}
}
}
// 用户类
abstract class User {
private String username;
private String password;
public User(String username, String password) {
this.username = username;
this.password = password;
}
public String getUsername() {
return username;
}
public String getPassword() {
return password;
}
}
// 管理员类
class Admin extends User {
public Admin(String username, String password) {
super(username, password);
}
}
// 读者类
class Reader extends User {
private int age;
public Reader(String username, int age) {
super(username, "123456");
this.age = age;
}
public int getAge() {
return age;
}
}
// 图书类
class Book {
private String name;
private String author;
private String press;
private boolean borrowed;
public Book(String name, String author, String press) {
this.name = name;
this.author = author;
this.press = press;
this.borrowed = false;
}
public String getName() {
return name;
}
public String getAuthor() {
return author;
}
public String getPress() {
return press;
}
public boolean isBorrowed() {
return borrowed;
}
public void setBorrowed(boolean borrowed) {
this.borrowed = borrowed;
}
}
// 图书馆类
class Library {
private ArrayList<User> users;
private ArrayList<Book> books;
private ArrayList<Reader> readers;
public Library() {
this.users = new ArrayList<>();
this.books = new ArrayList<>();
this.readers = new ArrayList<>();
}
public boolean addUser(User user) {
return users.add(user);
}
public User login(String username, String password) {
for (User user : users) {
if (user.getUsername().equals(username) && user.getPassword().equals(password)) {
return user;
}
}
return null;
}
public boolean addBook(Book book) {
return books.add(book);
}
public boolean deleteBook(String name) {
Book book = searchBook(name);
if (book == null) {
return false;
} else {
return books.remove(book);
}
}
public boolean modifyBook(Book oldBook, Book newBook) {
int index = books.indexOf(oldBook);
if (index == -1) {
return false;
} else {
books.set(index, newBook);
return true;
}
}
public Book searchBook(String name) {
for (Book book : books) {
if (book.getName().equals(name)) {
return book;
}
}
return null;
}
public boolean borrowBook(Book book, Reader reader) {
if (book.isBorrowed()) {
return false;
} else {
book.setBorrowed(true);
return true;
}
}
public boolean returnBook(Book book, Reader reader) {
if (book.isBorrowed()) {
book.setBorrowed(false);
return true;
} else {
return false;
}
}
public boolean addReader(Reader reader) {
return readers.add(reader);
}
public boolean deleteReader(String name) {
Reader reader = searchReader(name);
if (reader == null) {
return false;
} else {
return readers.remove(reader);
}
}
public boolean modifyReader(Reader oldReader, Reader newReader) {
int index = readers.indexOf(oldReader);
if (index == -1) {
return false;
} else {
readers.set(index, newReader);
return true;
}
}
public Reader searchReader(String name) {
for (Reader reader : readers) {
if (reader.getUsername().equals(name)) {
return reader;
}
}
return null;
}
}
```
请注意,这只是一个简单的示例,可能并不完美。在实际使用中,您需要根据具体需求进行修改和完善。
相关推荐
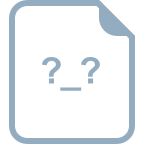
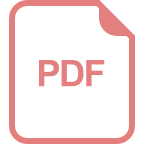
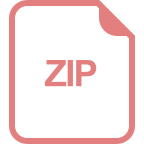













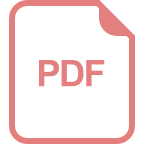
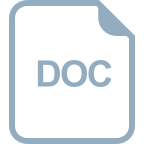
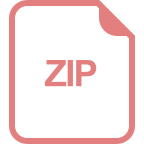