用Java语音设计哲学家进餐问题算法 设计要求:哲学家有N个,规定全体到齐后开始讨论,在讨论的间隙哲学家进餐,每人进餐时都需使用刀、叉合一把,所有哲学家刀和叉都拿到后才能进餐。哲学家的人数、餐桌上的布置自行设定,实现刀和叉的互斥使用算法的程序实现。要求用到PV操作
时间: 2023-11-22 08:53:40 浏览: 55
哲学家进餐问题是一个经典的并发问题,下面是一个使用Java语言实现的算法,使用PV操作来实现刀和叉的互斥使用:
```java
import java.util.concurrent.Semaphore;
public class DiningPhilosophers {
private Semaphore[] forks;
private Semaphore table;
private int numPhilosophers;
public DiningPhilosophers(int numPhilosophers) {
this.numPhilosophers = numPhilosophers;
forks = new Semaphore[numPhilosophers];
for (int i = 0; i < numPhilosophers; i++) {
forks[i] = new Semaphore(1);
}
table = new Semaphore(numPhilosophers - 1);
}
public void startDinner() {
Thread[] threads = new Thread[numPhilosophers];
for (int i = 0; i < numPhilosophers; i++) {
final int philosopherIndex = i;
threads[i] = new Thread(() -> {
try {
while (true) {
// philosopher thinks
System.out.println("Philosopher " + philosopherIndex + " is thinking...");
Thread.sleep((long) (Math.random() * 10000));
// philosopher is hungry
System.out.println("Philosopher " + philosopherIndex + " is hungry...");
// acquire table
table.acquire();
// acquire left fork
forks[philosopherIndex].acquire();
// acquire right fork
forks[(philosopherIndex + 1) % numPhilosophers].acquire();
// philosopher eats
System.out.println("Philosopher " + philosopherIndex + " is eating...");
Thread.sleep((long) (Math.random() * 10000));
// release right fork
forks[(philosopherIndex + 1) % numPhilosophers].release();
// release left fork
forks[philosopherIndex].release();
// release table
table.release();
}
} catch (InterruptedException e) {
e.printStackTrace();
}
});
threads[i].start();
}
}
public static void main(String[] args) {
DiningPhilosophers diningPhilosophers = new DiningPhilosophers(5);
diningPhilosophers.startDinner();
}
}
```
在这个算法中,我们使用了Semaphore类来实现PV操作。桌子上的信号量`table`的初始值为`numPhilosophers - 1`,这意味着只有当一些哲学家放下叉子和刀子之后,才有可能有另一个哲学家能够开始进餐。每个叉子和刀子都被表示为一个信号量,初始值为1,表示可用。
当哲学家想要进餐时,他必须先获得桌子上的信号量`table`,这是一个有限资源,只有在桌子上有空位时才能获得。然后他需要获得他左边的叉子和刀子,如果这些叉子和刀子都被其他哲学家使用,则他必须等待。一旦他获得了他左边的叉子和刀子,他需要获得他右边的叉子和刀子,如果这些叉子和刀子都被其他哲学家使用,则他必须等待。一旦他获得了他右边的叉子和刀子,他就可以开始进餐。进餐完成后,他需要放下他右边的叉子和刀子,然后放下他左边的叉子和刀子,最后放下桌子上的信号量`table`。
阅读全文
相关推荐
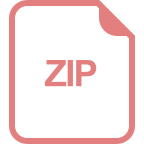
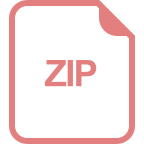
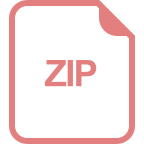














