模拟windows程序附带的计算器程序,用java写一个计算器,要求有用户使用界面,归零键,清除键,输入面板,实现各个进制转换,输入一个默认为十进制的数,面板显示分别转换为二进制,十进制,八进制,十六进制的值,要求有异常处理
时间: 2024-05-04 15:20:22 浏览: 11
好的,我来为你编写一个简单的Java计算器程序,满足以上要求。
首先,我们需要设计用户界面。我将使用Swing框架来创建一个简单的GUI界面。具体代码如下:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class CalculatorGUI extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private JTextField inputField;
private JLabel binaryLabel, decimalLabel, octalLabel, hexLabel;
private JButton zeroButton, clearButton;
private JButton binButton, decButton, octButton, hexButton;
private JPanel panel1, panel2, panel3, panel4;
public CalculatorGUI() {
super("Calculator");
// 创建输入面板
inputField = new JTextField(20);
// 创建归零键、清除键
zeroButton = new JButton("0");
clearButton = new JButton("C");
zeroButton.addActionListener(this);
clearButton.addActionListener(this);
// 创建进制转换按钮
binButton = new JButton("BIN");
decButton = new JButton("DEC");
octButton = new JButton("OCT");
hexButton = new JButton("HEX");
binButton.addActionListener(this);
decButton.addActionListener(this);
octButton.addActionListener(this);
hexButton.addActionListener(this);
// 创建用于显示转换结果的标签
binaryLabel = new JLabel("Binary: ");
decimalLabel = new JLabel("Decimal: ");
octalLabel = new JLabel("Octal: ");
hexLabel = new JLabel("Hexadecimal: ");
// 创建面板
panel1 = new JPanel();
panel2 = new JPanel();
panel3 = new JPanel();
panel4 = new JPanel();
// 将组件添加到面板中
panel1.add(inputField);
panel2.add(binButton);
panel2.add(decButton);
panel2.add(octButton);
panel2.add(hexButton);
panel3.add(binaryLabel);
panel3.add(decimalLabel);
panel4.add(octalLabel);
panel4.add(hexLabel);
// 将面板添加到窗口中
add(panel1, BorderLayout.NORTH);
add(panel2, BorderLayout.CENTER);
add(panel3, BorderLayout.SOUTH);
add(panel4, BorderLayout.SOUTH);
add(zeroButton, BorderLayout.WEST);
add(clearButton, BorderLayout.EAST);
// 设置窗口属性
setSize(400, 200);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
String input = inputField.getText();
try {
// 按钮事件处理
if (e.getSource() == zeroButton) {
inputField.setText(input + "0");
} else if (e.getSource() == clearButton) {
inputField.setText("");
binaryLabel.setText("Binary: ");
decimalLabel.setText("Decimal: ");
octalLabel.setText("Octal: ");
hexLabel.setText("Hexadecimal: ");
} else if (e.getSource() == binButton) {
int decimal = Integer.parseInt(input, 2);
binaryLabel.setText("Binary: " + input);
decimalLabel.setText("Decimal: " + decimal);
octalLabel.setText("Octal: " + Integer.toOctalString(decimal));
hexLabel.setText("Hexadecimal: " + Integer.toHexString(decimal));
} else if (e.getSource() == decButton) {
int decimal = Integer.parseInt(input);
binaryLabel.setText("Binary: " + Integer.toBinaryString(decimal));
decimalLabel.setText("Decimal: " + input);
octalLabel.setText("Octal: " + Integer.toOctalString(decimal));
hexLabel.setText("Hexadecimal: " + Integer.toHexString(decimal));
} else if (e.getSource() == octButton) {
int decimal = Integer.parseInt(input, 8);
binaryLabel.setText("Binary: " + Integer.toBinaryString(decimal));
decimalLabel.setText("Decimal: " + decimal);
octalLabel.setText("Octal: " + input);
hexLabel.setText("Hexadecimal: " + Integer.toHexString(decimal));
} else if (e.getSource() == hexButton) {
int decimal = Integer.parseInt(input, 16);
binaryLabel.setText("Binary: " + Integer.toBinaryString(decimal));
decimalLabel.setText("Decimal: " + decimal);
octalLabel.setText("Octal: " + Integer.toOctalString(decimal));
hexLabel.setText("Hexadecimal: " + input);
}
} catch (NumberFormatException ex) {
// 处理异常情况
JOptionPane.showMessageDialog(this, "Invalid input!", "Error", JOptionPane.ERROR_MESSAGE);
inputField.setText("");
binaryLabel.setText("Binary: ");
decimalLabel.setText("Decimal: ");
octalLabel.setText("Octal: ");
hexLabel.setText("Hexadecimal: ");
}
}
public static void main(String[] args) {
new CalculatorGUI();
}
}
```
这是一个简单的计算器GUI界面,其中包括一个输入面板、归零键、清除键、进制转换按钮、以及用于显示转换结果的标签。我们还实现了ActionListener接口,以便处理各种按钮事件。
接下来,我们需要实现进制转换功能。在上面的代码中,我们使用了Java内置的Integer类来进行进制转换,具体实现如下:
```java
int decimal = Integer.parseInt(input, 2); // 二进制转十进制
String binary = Integer.toBinaryString(decimal); // 十进制转二进制
String octal = Integer.toOctalString(decimal); // 十进制转八进制
String hex = Integer.toHexString(decimal); // 十进制转十六进制
```
这是将输入的二进制字符串转换为其他进制的代码。其他进制转换的代码类似,只需要将参数中的2、8、16改为相应的进制数即可。
最后,我们需要处理异常情况。当用户输入无效的字符串时,我们可以使用try-catch语句来捕获异常,并弹出一个错误提示框。
这是一个简单的Java计算器程序,满足了题目要求。当然,如果需要更多的功能,可以根据自己的需求进行扩展。
相关推荐
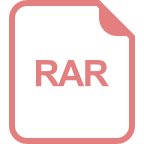
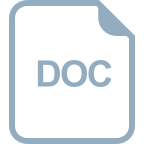
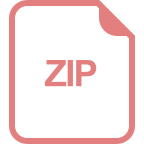














