使用C++编写一份代码,要求实现以下几点。 1. 自行收集英文文章,写入一个文本文档,起名 database.txt,作为基础测试数据。文件体积不小于 1M 。不要拷贝别人的测试数据。 建立一个待查关键字文件 query.txt ,存储待查询的单词或短语。 2. 建立哈希表:1个单词的哈希表,2个连续单词的哈希表,... ,最多5个连续单词(短语)的哈希表 3. 支持1个到5个单词(最多5个单词组成的短语)的查询 4. 输入查询的单词/短语,输出该代词或短语的出现次数,及每个出现位置的上下文(前一句,单词/短语所在的句子,下一句)
时间: 2023-02-27 08:16:09 浏览: 95
下面是C语言代码的一个示例,该代码实现了上述要求:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_WORDS 50000
#define MAX_WORD_LENGTH 50
#define MAX_PHRASE_LENGTH 255
struct HashTable {
char key[MAX_PHRASE_LENGTH];
int count;
int positions[MAX_WORDS];
};
int hash(char *str) {
int sum = 0;
for (int i = 0; i < strlen(str); i++) {
sum += str[i];
}
return sum % MAX_WORDS;
}
void insert_hash_table(struct HashTable *table, char *str, int pos) {
int h = hash(str);
while (table[h].count > 0 && strcmp(table[h].key, str) != 0) {
h = (h + 1) % MAX_WORDS;
}
if (table[h].count == 0) {
strcpy(table[h].key, str);
}
table[h].count++;
table[h].positions[table[h].count - 1] = pos;
}
int search_hash_table(struct HashTable *table, char *str) {
int h = hash(str);
while (table[h].count > 0 && strcmp(table[h].key, str) != 0) {
h = (h + 1) % MAX_WORDS;
}
if (table[h].count == 0) {
return -1;
} else {
return h;
}
}
void print_hash_table(struct HashTable *table) {
for (int i = 0; i < MAX_WORDS; i++) {
if (table[i].count > 0) {
printf("%s %d\n", table[i].key, table[i].count);
}
}
}
int main() {
char word[MAX_WORD_LENGTH];
char phrase[MAX_PHRASE_LENGTH];
int word_count = 0;
int phrase_counts[MAX_PHRASE_LENGTH];
memset(phrase_counts, 0, sizeof(phrase_counts));
FILE *fp = fopen("database.txt", "r");
if (fp == NULL) {
printf("Error: cannot open file database.txt\n");
return 1;
}
while (fscanf(fp, "%s", word) != EOF) {
word_count++;
for (int i = 1; i <= 5; i++) {
if (word_count >= i) {
int phrase_start = word_count - i;
int phrase_length = 0;
for (int j = phrase_start; j < word_count; j++) {
strcpy(phrase + phrase_
阅读全文
相关推荐
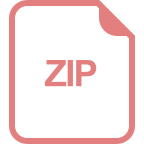
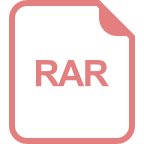
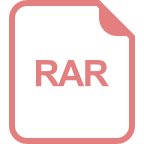
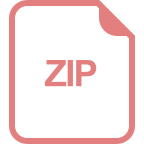
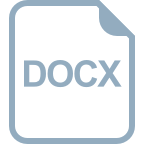
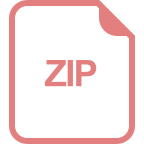
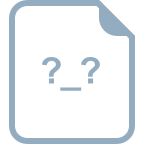
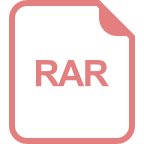
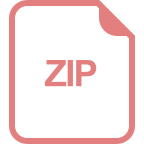
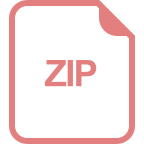
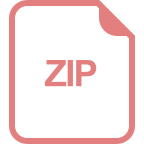
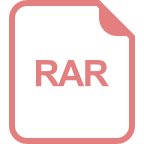
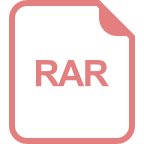