layui实现动态表格数据过程
时间: 2024-05-05 18:16:49 浏览: 18
1. 引入layui库和jQuery库。
```html
<!-- 引入layui库 -->
<link rel="stylesheet" href="layui/css/layui.css">
<script src="layui/layui.js"></script>
<!-- 引入jQuery库 -->
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
```
2. 创建一个表格容器。
```html
<div id="table"></div>
```
3. 初始化表格。
```javascript
layui.use(['table'], function() {
var table = layui.table;
// 定义列
var cols = [
{field: 'id', title: 'ID', width: 80},
{field: 'name', title: '姓名', width: 120},
{field: 'age', title: '年龄', width: 80},
{field: 'sex', title: '性别', width: 80},
{field: 'address', title: '地址', width: 200}
];
// 初始化表格
table.render({
elem: '#table', // 容器id
url: '/data', // 数据接口
cols: [cols], // 列
page: true // 是否开启分页
});
});
```
4. 从服务器获取数据。
```javascript
layui.use(['table'], function() {
var table = layui.table;
var index = layer.load(1); // 加载动画
// 定义列
var cols = [
{field: 'id', title: 'ID', width: 80},
{field: 'name', title: '姓名', width: 120},
{field: 'age', title: '年龄', width: 80},
{field: 'sex', title: '性别', width: 80},
{field: 'address', title: '地址', width: 200}
];
// 获取数据
$.ajax({
url: '/data',
type: 'GET',
dataType: 'json',
success: function(res) {
layer.close(index); // 关闭动画
table.render({
elem: '#table', // 容器id
data: res.data, // 数据
cols: [cols], // 列
page: true // 是否开启分页
});
}
});
});
```
5. 实现表格数据的动态更新。
```javascript
layui.use(['table'], function() {
var table = layui.table;
// 定义列
var cols = [
{field: 'id', title: 'ID', width: 80},
{field: 'name', title: '姓名', width: 120},
{field: 'age', title: '年龄', width: 80},
{field: 'sex', title: '性别', width: 80},
{field: 'address', title: '地址', width: 200}
];
// 初始化表格
var tableIns = table.render({
elem: '#table', // 容器id
url: '/data', // 数据接口
cols: [cols], // 列
page: true // 是否开启分页
});
// 刷新表格数据
function refreshTable() {
table.reload('table', {
url: '/data',
done: function() {
setTimeout(refreshTable, 5000); // 5秒后再次刷新
}
});
}
// 开始刷新表格数据
setTimeout(refreshTable, 5000); // 5秒后开始刷新
});
```
6. 完整的代码。
```html
<!DOCTYPE html>
<html>
<head>
<title>动态表格数据</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<!-- 引入layui库 -->
<link rel="stylesheet" href="layui/css/layui.css">
<script src="layui/layui.js"></script>
<!-- 引入jQuery库 -->
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
</head>
<body>
<div id="table"></div>
<script>
layui.use(['table'], function() {
var table = layui.table;
// 定义列
var cols = [
{field: 'id', title: 'ID', width: 80},
{field: 'name', title: '姓名', width: 120},
{field: 'age', title: '年龄', width: 80},
{field: 'sex', title: '性别', width: 80},
{field: 'address', title: '地址', width: 200}
];
// 初始化表格
var tableIns = table.render({
elem: '#table', // 容器id
url: '/data', // 数据接口
cols: [cols], // 列
page: true // 是否开启分页
});
// 刷新表格数据
function refreshTable() {
table.reload('table', {
url: '/data',
done: function() {
setTimeout(refreshTable, 5000); // 5秒后再次刷新
}
});
}
// 开始刷新表格数据
setTimeout(refreshTable, 5000); // 5秒后开始刷新
});
</script>
</body>
</html>
```
相关推荐
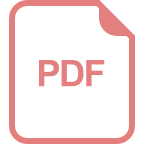
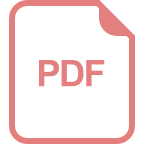
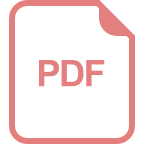














