根据输入的正整数y所代表的年份,计算输出该年份是否为闰年
时间: 2024-05-29 09:12:07 浏览: 64
闰年的判断规则是:
1. 能被4整除但不能被100整除的年份是闰年;
2. 能被400整除的年份也是闰年。
因此,可以使用以下代码来判断:
y = int(input("请输入一个年份:"))
if (y % 4 == 0 and y % 100 != 0) or (y % 400 == 0):
print(y, "是闰年")
else:
print(y, "不是闰年")
相关问题
用Java写一个代码根据输入的正整数y所代表的年份,计算输出该年份是否为闰年 闰年的判断标准: 能够被4整除且不能被100整除的年份 或者能够被400整除的年份 输入格式: 输入n取值范围是 【1..3000】 输出格式: 是闰年,输出 yes 非闰年,输出 no
在Java中,你可以编写如下的代码来检查一个年份是否是闰年:
```java
import java.util.Scanner;
public class LeapYearCalculator {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入一个年份(1到3000之间):");
int year = scanner.nextInt();
if (isValidLeapYear(year)) {
System.out.println(year + " 是闰年,输出 yes");
} else {
System.out.println(year + " 非闰年,输出 no");
}
scanner.close();
}
// 判断闰年的方法
public static boolean isValidLeapYear(int year) {
if (year <= 0 || year > 3000) {
return false; // 年份超出范围
}
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) { // 按照闰年的规则判断
return true;
} else {
return false;
}
}
}
```
在这个程序中,首先通过Scanner获取用户输入的年份,然后调用`isValidLeapYear`方法进行判断。如果满足闰年的条件,则输出"yes",否则输出"no"。
输入x,y,输出[x,y]区间中闰年个数,并在下一行输出所有闰年年份数字。 (当x,y输入为负数或浮点数时提示输入错误并提示重新输入) (无闰年年份则输出没有) (输入两个正整数x,y用空格隔开,换行后输出所有闰年年份数字)
这是一个编程任务,需要编写一个程序来计算指定范围内的闰年数量以及列出所有的闰年。在Python中,你可以按照以下步骤来实现:
1. 首先检查输入是否为正整数,如果不是,则打印错误消息并提示用户重新输入。
2. 使用`is_leap_year`函数判断一个年份是否为闰年,闰年的规则是能被4整除但不能被100整除,或者可以被400整除。
3. 使用循环遍历从x到y的所有年份,计算并记录闰年。
4. 输出结果,包括闰年的总数和具体的闰年列表。
下面是一个简单的Python示例代码:
```python
def is_leap_year(year):
if isinstance(year, int) and year > 0:
return year % 4 == 0 and (year % 100 != 0 or year % 400 == 0)
else:
print("输入错误,请输入正整数。")
return None
def count_and_list_leap_years(x, y):
leap_years = []
for year in range(x, y + 1):
if is_leap_year(year):
leap_years.append(year)
print(year, end=' ')
print("\n总共有{}个闰年。".format(len(leap_years)))
while True:
try:
x, y = map(int, input("请输入两个正整数x和y,用空格隔开:").split())
count_and_list_leap_years(x, y)
break
except ValueError:
print("输入错误,请输入整数。")
```
当你运行这个程序时,它会提示用户输入x和y,然后计算并显示指定范围内闰年的个数和具体年份。
相关推荐




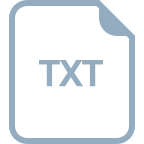
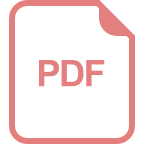
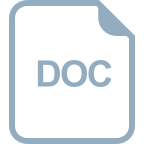
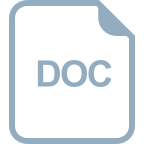


