对tmdb电影榜单数据集,建立模型并进行评分预测,具体代码实现
时间: 2024-04-02 14:34:30 浏览: 261
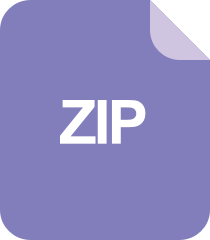
数据可视化 基于TMDB数据集的电影数据分析

好的,我可以为你介绍一个用于对电影评分进行预测的模型。此模型基于机器学习算法,使用TMDB电影数据集,将电影的各种属性作为输入特征,预测电影的评分。
首先,我们需要准备数据。可以从TMDB官网下载数据集,或者使用Kaggle上提供的TMDB电影数据集。下载后,我们可以使用Pandas库来读取数据集,然后进行数据清洗和特征提取。
```python
import pandas as pd
# 读取数据
data = pd.read_csv('tmdb_5000_movies.csv')
# 清洗数据
data = data.dropna()
data = data.drop(['homepage', 'original_title', 'overview', 'spoken_languages', 'status', 'tagline', 'title'], axis=1)
# 特征提取
genres = data['genres'].apply(lambda x: [i['name'] for i in eval(x)])
keywords = data['keywords'].apply(lambda x: [i['name'] for i in eval(x)])
production_companies = data['production_companies'].apply(lambda x: [i['name'] for i in eval(x)])
production_countries = data['production_countries'].apply(lambda x: [i['name'] for i in eval(x)])
data = data.drop(['genres', 'keywords', 'production_companies', 'production_countries'], axis=1)
data = pd.concat([data, genres, keywords, production_companies, production_countries], axis=1)
```
接下来,我们可以将数据集划分为训练集和测试集,并使用Scikit-learn库中的随机森林算法来训练模型。
```python
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestRegressor
from sklearn.metrics import mean_squared_error
# 划分数据集
X = data.drop(['vote_average'], axis=1)
y = data['vote_average']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 训练模型
model = RandomForestRegressor(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# 预测评分
y_pred = model.predict(X_test)
mse = mean_squared_error(y_test, y_pred)
print("Mean Squared Error:", mse)
```
最后,我们可以使用模型对新电影进行评分预测。
```python
# 预测新电影评分
new_movie = {'budget': 100000000, 'popularity': 100, 'runtime': 120, 'Action': 1, 'Adventure': 1, 'Comedy': 0, 'Crime': 0, 'Documentary': 0, 'Drama': 1, 'Family': 0, 'Fantasy': 1, 'History': 0, 'Horror': 0, 'Music': 0, 'Mystery': 0, 'Romance': 0, 'Science Fiction': 1, 'TV Movie': 0, 'Thriller': 1, 'War': 0, 'Western': 0, 'based on novel': 0, 'duringcreditsstinger': 0, 'independent film': 0, 'murder': 0, 'sequel': 0, 'sport': 0, 'superhero': 0, 'new york': 0, 'los angeles': 1}
new_movie = pd.DataFrame([new_movie])
rating = model.predict(new_movie)
print("Predicted Rating:", rating)
```
这里,我们使用了一些电影属性,如预算、流行度、时长以及电影的类型、关键字和制片公司等进行预测。随机森林算法是一种强大的机器学习算法,可以处理多种类型的数据,并在许多任务中表现良好。
希望这个代码示例能够帮助你了解如何使用机器学习算法进行电影评分预测。
阅读全文
相关推荐
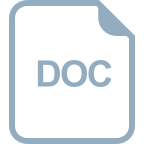
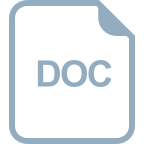










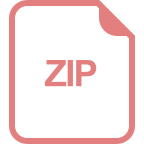
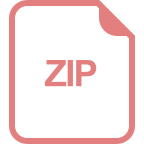
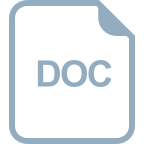
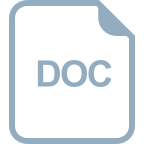
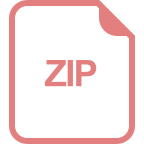
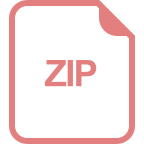