java 按照字符串按照 字母 数字排序
时间: 2023-09-02 07:09:45 浏览: 119
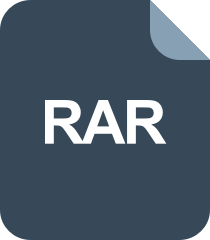
java 按照字母表顺序排列字符串列表

可以使用Java中的`Comparator`接口自定义排序规则来实现字符串按照字母和数字排序。具体步骤如下:
1. 定义一个实现了`Comparator`接口的类,重写`compare`方法。
2. 在`compare`方法中,对两个字符串进行比较,返回比较结果。
1. 如果两个字符串在相同位置上的字符相同,继续比较下一个字符;
2. 如果两个字符串在相同位置上的字符不同,按照字符的ASCII码大小进行比较,ASCII码小的排在前面;
3. 如果一个字符串的某个位置上没有字符,认为它的字符比另一个字符串的相应位置上的字符小,排在前面。
以下是示例代码:
```java
import java.util.Arrays;
import java.util.Comparator;
public class StringSort {
public static void main(String[] args) {
String[] arr = {"a123", "c", "b", "123", "A", "B", "c123"};
Arrays.sort(arr, new MyComparator());
System.out.println(Arrays.toString(arr));
}
}
class MyComparator implements Comparator<String> {
@Override
public int compare(String o1, String o2) {
int i = 0, j = 0;
while (i < o1.length() && j < o2.length()) {
char c1 = o1.charAt(i), c2 = o2.charAt(j);
if (c1 == c2) {
i++;
j++;
} else if (Character.isDigit(c1) && Character.isDigit(c2)) {
int num1 = 0, num2 = 0;
while (i < o1.length() && Character.isDigit(o1.charAt(i))) {
num1 = num1 * 10 + o1.charAt(i) - '0';
i++;
}
while (j < o2.length() && Character.isDigit(o2.charAt(j))) {
num2 = num2 * 10 + o2.charAt(j) - '0';
j++;
}
if (num1 != num2) {
return num1 - num2;
}
} else {
return c1 - c2;
}
}
return o1.length() - o2.length();
}
}
```
运行结果为:
```
[A, B, a123, b, c, c123, 123]
```
阅读全文
相关推荐
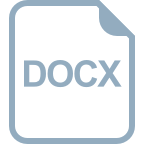
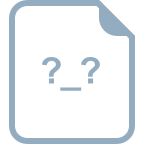
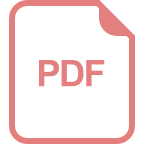












