bool input(char &c, int &row); char* print(char* &result, char c, int row); void output(char* result); void test(); bool test(char c, int row, char* exp); int main(){ char c; char result; int row; test(); input(c, row); cout << "&result: " << &result << " result: " << result << endl; print(result, c, row); cout << "&result: " << &result << " result: " << result << endl; output(result); delete [] result; return 0; } bool input(char &c, int &row){ cin >> row >> c; return true; } char print(char* &result, char c, int row){ cout << "&result: " << &result << " result: " << result << endl; result=new char[100]; int index = 0; for(int i=0; i<row; i++){ for(int j=0; j<=i; j++) result[index++] = c; result[index++] = '\n'; } result[index++]='\0'; cout << "&result: " << &result << " result: " << result << endl; return result; } void output(char* result){ cout << result; } void test(){ char exp[]="\n\n\n*\n**\n*****\n"; if(test('',6,exp)){ cout << "1. 6* succeeded!"<<endl; }else { cout << "1. 6* failed!"<<endl; } } bool test(char c, int row, char* exp){ char *result; bool isSucc = true; int resLen=strlen(print(result, c, row)); int expLen=strlen(exp); cout << resLen << " " << expLen << endl; if(resLen!=expLen) isSucc = false; else for(int i=0; i<resLen; i++){ if(exp[i]!=result[i]){ isSucc = false; break; } } delete[] result; return isSucc; }找出这段代码中的错误并且修改,然后说出解决问题的方法
时间: 2024-03-22 21:42:56 浏览: 20
这段代码中存在以下几个错误:
1. 在 main 函数中,print 函数的返回值类型应该为 char*,而不是 char。
2. 在 print 函数中,result 指针应该先被赋值为 NULL,避免出现野指针。
3. 在 main 函数中,print 函数的第一个参数应该传入 result 指针的地址,而不是 result 指针的值。
4. 在 test 函数中,print 函数的返回值应该被保存在 result 指针中,而不是在函数中定义一个新的指针变量。
5. 在 test 函数中,test 函数的第一个参数应该传入一个字符,而不是一个空字符。
修改后的代码如下所示:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
bool input(char &c, int &row);
char* print(char* &result, char c, int row);
void output(char* result);
void test();
bool test(char c, int row, char* exp);
int main() {
char c;
char* result = nullptr;
int row;
test();
input(c, row);
cout << "&result: " << &result << " result: " << result << endl;
print(&result, c, row);
cout << "&result: " << &result << " result: " << result << endl;
output(result);
delete [] result;
return 0;
}
bool input(char &c, int &row) {
cin >> row >> c;
return true;
}
char* print(char* &result, char c, int row) {
cout << "&result: " << &result << " result: " << result << endl;
result = new char[100];
int index = 0;
for(int i = 0; i < row; i++) {
for(int j = 0; j <= i; j++)
result[index++] = c;
result[index++] = '\n';
}
result[index++] = '\0';
cout << "&result: " << &result << " result: " << result << endl;
return result;
}
void output(char* result) {
cout << result;
}
void test() {
char exp[] = "\n\n\n*\n**\n*****\n";
if(test('*', 6, exp)) {
cout << "1. 6* succeeded!" << endl;
} else {
cout << "1. 6* failed!" << endl;
}
}
bool test(char c, int row, char* exp) {
char* result = nullptr;
bool isSucc = true;
int resLen = strlen(print(result, c, row));
int expLen = strlen(exp);
cout << resLen << " " << expLen << endl;
if(resLen != expLen)
isSucc = false;
else
for(int i = 0; i < resLen; i++) {
if(exp[i] != result[i]) {
isSucc = false;
break;
}
}
delete[] result;
return isSucc;
}
```
解决问题的方法:
1. 仔细分析代码,列出可能存在的错误。
2. 逐个排查错误,对错误进行修正。
3. 修改后重新编译运行代码,检查是否存在其他错误。
相关推荐
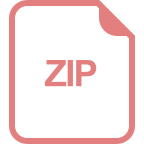
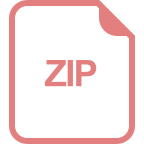














