我需要一段Springboot使用定时任务的代码
时间: 2023-09-26 21:07:04 浏览: 141
### 回答1:
好的,我可以为您提供一段 Vue 3 中的模糊查询代码。
首先,您需要在模板中添加一个输入框,并为其绑定一个响应式数据,例如 `query`:
```html
<template>
<input v-model="query" placeholder="请输入查询关键字" />
</template>
```
然后,您可以使用 computed 属性来计算出符合查询条件的数据,例如:
```js
<script>
export default {
### 回答2:
在Spring Boot中使用定时任务非常简单,只需要遵循以下步骤:
1. 添加依赖:在pom.xml文件中添加Spring Boot的定时任务依赖。
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
```
2. 创建一个定时任务类:在你的Java包中创建一个继承自`org.springframework.scheduling.annotation.Scheduled`的类。
```java
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class MyScheduledTask {
// 每天12点执行一次定时任务
@Scheduled(cron = "0 0 12 * * ?")
public void doTask() {
// 执行你的定时任务逻辑
System.out.println("定时任务执行中...");
}
}
```
3. 启用定时任务:在你的Spring Boot应用主类或配置类上添加`@EnableScheduling`注解以启用定时任务。
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.scheduling.annotation.EnableScheduling;
@SpringBootApplication
@EnableScheduling
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
上述代码展示了一个简单的定时任务,在每天的12点执行一次。你可以根据需要修改`@Scheduled`注解的cron表达式来调整定时任务的执行时间。
通过这种方式,你可以轻松地在Spring Boot项目中使用定时任务。
### 回答3:
下面是一个使用Spring Boot的定时任务代码示例:
1. 首先,确保你的项目中已经引入了Spring Boot的相关依赖。在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
```
2. 创建一个定时任务类,比如`MyTask`,并使用`@Component`注解将其纳入Spring容器管理:
```java
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class MyTask {
@Scheduled(fixedRate = 1000) // 每隔1秒执行一次定时任务
public void doTask() {
// 定时任务要执行的逻辑代码
System.out.println("Hello, World!");
}
}
```
3. 在Spring Boot应用的主类中,添加`@EnableScheduling`注解启用定时任务的功能:
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.scheduling.annotation.EnableScheduling;
@SpringBootApplication
@EnableScheduling
public class MyApp {
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
}
}
```
这样,定时任务就会在应用启动后每隔1秒执行一次`MyTask`类中的`doTask`方法。你可以根据需要修改注解`@Scheduled`的参数,比如设置固定的延迟时间、定时执行的时间表达式等。
注意:在使用定时任务时,需要确保项目中已经启用了Spring的定时任务功能。你可以在应用的配置文件(比如application.properties或application.yml)中添加以下配置:
```yaml
spring:
task:
scheduling:
enabled: true
```
这样就完成了Spring Boot使用定时任务的代码。希望能对你有所帮助!
阅读全文
相关推荐

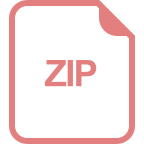
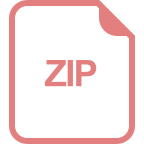
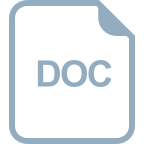
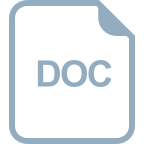
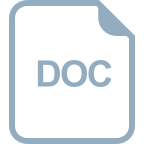
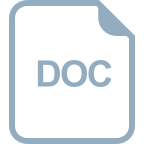
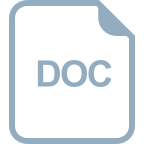
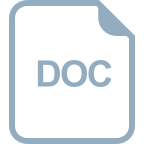
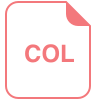





