用c语言写一个图书信息管理系统要求首界面有登录,注册,退出的功能,登录成功后界面有录入图书信息,查找图书信息,修改图书信息,分类统计图书数量的功能
时间: 2024-03-26 17:38:00 浏览: 21
好的,我可以帮您解决这个问题。首先,您需要使用C语言编写一个控制台应用程序,该程序将实现您所述的功能。下面是一个简单的实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct book {
char title[100];
char author[100];
char publisher[100];
int year;
int copies;
};
struct user {
char username[100];
char password[100];
};
int main() {
int choice;
printf("Welcome to the Book Management System!\n");
printf("1. Login\n");
printf("2. Register\n");
printf("3. Quit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
if (choice == 1) {
// Login
struct user users[100];
int num_users = 0;
FILE *fp = fopen("users.dat", "rb");
if (fp != NULL) {
fread(&num_users, sizeof(int), 1, fp);
fread(users, sizeof(struct user), num_users, fp);
fclose(fp);
}
char username[100], password[100];
printf("Enter your username: ");
scanf("%s", username);
printf("Enter your password: ");
scanf("%s", password);
int i;
for (i = 0; i < num_users; i++) {
if (strcmp(users[i].username, username) == 0 &&
strcmp(users[i].password, password) == 0) {
printf("Login successful!\n");
break;
}
}
if (i == num_users) {
printf("Invalid username/password!\n");
exit(1);
}
// Main menu
printf("1. Add book\n");
printf("2. Find book\n");
printf("3. Update book\n");
printf("4. Statistics\n");
printf("5. Logout\n");
printf("Enter your choice: ");
scanf("%d", &choice);
struct book books[100];
int num_books = 0;
fp = fopen("books.dat", "rb");
if (fp != NULL) {
fread(&num_books, sizeof(int), 1, fp);
fread(books, sizeof(struct book), num_books, fp);
fclose(fp);
}
while (choice != 5) {
switch (choice) {
case 1: {
// Add book
printf("Enter title: ");
scanf("%s", books[num_books].title);
printf("Enter author: ");
scanf("%s", books[num_books].author);
printf("Enter publisher: ");
scanf("%s", books[num_books].publisher);
printf("Enter year: ");
scanf("%d", &books[num_books].year);
printf("Enter copies: ");
scanf("%d", &books[num_books].copies);
num_books++;
fp = fopen("books.dat", "wb");
fwrite(&num_books, sizeof(int), 1, fp);
fwrite(books, sizeof(struct book), num_books, fp);
fclose(fp);
break;
}
case 2: {
// Find book
char title[100];
printf("Enter title: ");
scanf("%s", title);
int i;
for (i = 0; i < num_books; i++) {
if (strcmp(books[i].title, title) == 0) {
printf("Title: %s\n", books[i].title);
printf("Author: %s\n", books[i].author);
printf("Publisher: %s\n", books[i].publisher);
printf("Year: %d\n", books[i].year);
printf("Copies: %d\n", books[i].copies);
break;
}
}
if (i == num_books) {
printf("Book not found!\n");
}
break;
}
case 3: {
// Update book
char title[100];
printf("Enter title: ");
scanf("%s", title);
int i;
for (i = 0; i < num_books; i++) {
if (strcmp(books[i].title, title) == 0) {
printf("Enter new title: ");
scanf("%s", books[i].title);
printf("Enter new author: ");
scanf("%s", books[i].author);
printf("Enter new publisher: ");
scanf("%s", books[i].publisher);
printf("Enter new year: ");
scanf("%d", &books[i].year);
printf("Enter new copies: ");
scanf("%d", &books[i].copies);
break;
}
}
if (i == num_books) {
printf("Book not found!\n");
} else {
fp = fopen("books.dat", "wb");
fwrite(&num_books, sizeof(int), 1, fp);
fwrite(books, sizeof(struct book), num_books, fp);
fclose(fp);
}
break;
}
case 4: {
// Statistics
int num_copies = 0;
int year_counts[100] = {0};
int i;
for (i = 0; i < num_books; i++) {
num_copies += books[i].copies;
year_counts[books[i].year]++;
}
printf("Total number of copies: %d\n", num_copies);
printf("Number of books per year:\n");
for (i = 0; i < 100; i++) {
if (year_counts[i] > 0) {
printf("%d: %d\n", i, year_counts[i]);
}
}
break;
}
default: {
printf("Invalid choice!\n");
break;
}
}
// Main menu
printf("1. Add book\n");
printf("2. Find book\n");
printf("3. Update book\n");
printf("4. Statistics\n");
printf("5. Logout\n");
printf("Enter your choice: ");
scanf("%d", &choice);
}
printf("Logout successful!\n");
} else if (choice == 2) {
// Register
struct user users[100];
int num_users = 0;
FILE *fp = fopen("users.dat", "rb");
if (fp != NULL) {
fread(&num_users, sizeof(int), 1, fp);
fread(users, sizeof(struct user), num_users, fp);
fclose(fp);
}
char username[100], password[100];
printf("Enter your username: ");
scanf("%s", username);
int i;
for (i = 0; i < num_users; i++) {
if (strcmp(users[i].username, username) == 0) {
printf("Username already exists!\n");
exit(1);
}
}
printf("Enter your password: ");
scanf("%s", password);
strcpy(users[num_users].username, username);
strcpy(users[num_users].password, password);
num_users++;
fp = fopen("users.dat", "wb");
fwrite(&num_users, sizeof(int), 1, fp);
fwrite(users, sizeof(struct user), num_users, fp);
fclose(fp);
printf("Registration successful!\n");
} else if (choice == 3) {
// Quit
printf("Goodbye!\n");
exit(0);
} else {
printf("Invalid choice!\n");
exit(1);
}
return 0;
}
```
该程序使用了结构体来表示图书和用户信息,并将它们保存在二进制文件中。用户可以登录、注册、退出,登录成功后可以添加、查找、更新图书信息,以及进行分类统计。如果您需要对该程序进行进一步修改和扩展,可以根据您的需求进行改动。
相关推荐
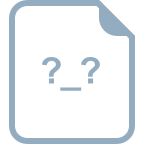
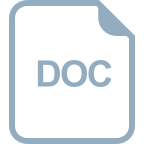
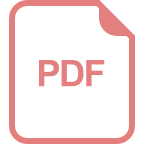















