springboot使用bean创建队列、交换机、以及binding怎么用java配置类创建。
时间: 2023-04-04 09:02:50 浏览: 371
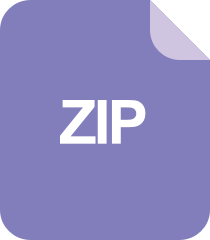
使用java类配置Bean
您好,关于springboot使用bean创建队列、交换机、以及binding的问题,可以通过在java配置类中使用@Bean注解来实现。具体实现步骤如下:
1. 创建一个配置类,使用@Configuration注解标注该类为配置类。
2. 在配置类中使用@Bean注解创建一个ConnectionFactory对象,用于连接RabbitMQ服务器。
3. 使用@Bean注解创建一个RabbitAdmin对象,用于管理RabbitMQ的队列、交换机等资源。
4. 使用@Bean注解创建一个Queue对象,用于创建队列。
5. 使用@Bean注解创建一个DirectExchange对象,用于创建交换机。
6. 使用@Bean注解创建一个Binding对象,将队列和交换机绑定在一起。
7. 最后,在需要使用队列的地方,可以通过@Autowired注解注入Queue对象,进行消息的发送和接收。
以上就是使用java配置类创建队列、交换机、以及binding的步骤。希望对您有所帮助。
阅读全文
相关推荐


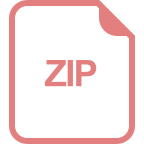
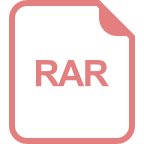
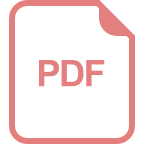
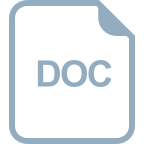
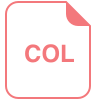
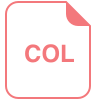






