springboot 集成rabbitMq 代码 和使用示例
时间: 2024-06-15 10:05:23 浏览: 16
当使用Spring Boot集成RabbitMQ时,需要进行以下几个步骤:
1. 添加依赖:在`pom.xml`文件中添加RabbitMQ的依赖项,例如:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-amqp</artifactId>
</dependency>
```
2. 配置连接信息:在`application.properties`或`application.yml`文件中配置RabbitMQ的连接信息,例如:
```yaml
spring.rabbitmq.host=your-rabbitmq-host
spring.rabbitmq.port=your-rabbitmq-port
spring.rabbitmq.username=your-username
spring.rabbitmq.password=your-password
```
3. 创建消息发送者和接收者:可以使用`RabbitTemplate`来发送和接收消息。例如,创建一个消息发送者:
```java
import org.springframework.amqp.rabbit.core.RabbitTemplate;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class MessageSender {
@Autowired
private RabbitTemplate rabbitTemplate;
public void sendMessage(String message) {
rabbitTemplate.convertAndSend("exchange-name", "routing-key", message);
}
}
```
创建一个消息接收者:
```java
import org.springframework.amqp.rabbit.annotation.RabbitListener;
import org.springframework.stereotype.Component;
@Component
public class MessageReceiver {
@RabbitListener(queues = "queue-name")
public void receiveMessage(String message) {
System.out.println("Received message: " + message);
}
}
```
4. 配置交换机和队列:可以使用`@Configuration`注解创建一个配置类,并在其中定义交换机和队列的绑定关系。例如:
```java
import org.springframework.amqp.core.Binding;
import org.springframework.amqp.core.BindingBuilder;
import org.springframework.amqp.core.DirectExchange;
import org.springframework.amqp.core.Queue;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class RabbitMQConfig {
@Bean
public DirectExchange exchange() {
return new DirectExchange("exchange-name");
}
@Bean
public Queue queue() {
return new Queue("queue-name");
}
@Bean
public Binding binding(Queue queue, DirectExchange exchange) {
return BindingBuilder.bind(queue).to(exchange).with("routing-key");
}
}
```
以上是一个简单的Spring Boot集成RabbitMQ的示例,你可以根据自己的需求进行配置和使用。
相关推荐
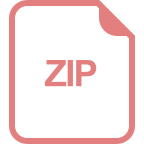
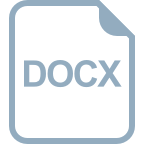














