c++ 重载运算符“=”,判断两个复数是否相等
时间: 2023-12-14 20:39:10 浏览: 113
在C++中,重载运算符“=”可以用来判断两个复数是否相等。示例代码如下:
```c++
class Complex {
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
bool operator ==(const Complex& c) const {
return (real == c.real) && (imag == c.imag);
}
Complex& operator =(const Complex& c) {
real = c.real;
imag = c.imag;
return *this;
}
private:
double real;
double imag;
};
```
在上面的代码中,我们定义了一个名为Complex的类,其中包含了两个成员变量real和imag,分别表示复数的实部和虚部。我们重载了运算符“==”和“=”,其中“==”用于判断两个复数是否相等,“=”用于将一个复数对象赋值给另外一个复数对象。
在重载“=”运算符时,需要注意返回值必须为引用类型,并且要使用const关键字修饰参数,以避免修改参数值。此外,需要在函数体中将源对象的成员变量值赋值给目标对象的成员变量值,并返回目标对象的引用。
相关问题
c++重载运算符==复数表示
在 C++ 中,可以通过重载运算符来定义复数的相等性比较。下面是一个示例实现:
```c++
#include <iostream>
using namespace std;
class Complex {
private:
double real;
double imag;
public:
Complex(double r = 0.0, double i = 0.0) {
real = r;
imag = i;
}
bool operator==(const Complex& other) const {
return (real == other.real) && (imag == other.imag);
}
};
int main() {
Complex a(3.0, 4.0);
Complex b(3.0, 4.0);
Complex c(5.0, 6.0);
cout << boolalpha;
cout << (a == b) << endl; // 输出 true
cout << (a == c) << endl; // 输出 false
return 0;
}
```
在上面的示例中,我们重载了 `==` 运算符,实现了复数之间的相等性比较。注意,我们使用了常量引用作为参数,并且在函数体内使用了 `const` 修饰符,以确保不会修改被比较的对象。
c++重载运算符“==”,判断两个复数是否相等。
可以使用类来实现复数的表示和运算符重载,以下是一个示例代码:
```c++
#include <iostream>
using namespace std;
class Complex {
private:
double real; // 复数实部
double imag; // 复数虚部
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
// 重载运算符==
bool operator==(const Complex& c) const {
return (real == c.real) && (imag == c.imag);
}
// 重载运算符!=,可以根据==实现
bool operator!=(const Complex& c) const {
return !(*this == c);
}
// 打印复数
void print() const {
cout << "(" << real << ", " << imag << "i)" << endl;
}
};
int main() {
Complex c1(1, 2);
Complex c2(1, 2);
Complex c3(2, 3);
if (c1 == c2) {
cout << "c1 == c2" << endl;
} else {
cout << "c1 != c2" << endl;
}
if (c1 == c3) {
cout << "c1 == c3" << endl;
} else {
cout << "c1 != c3" << endl;
}
return 0;
}
```
运行结果为:
```
c1 == c2
c1 != c3
```
其中,重载运算符使用了 const 引用,以避免创建副本,提高效率。
阅读全文
相关推荐
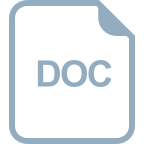
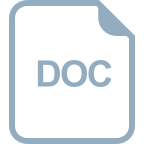
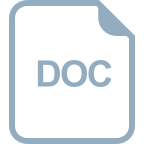

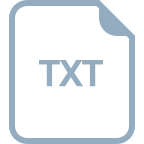
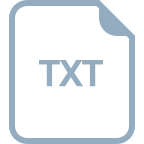
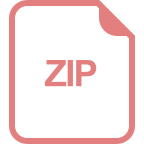
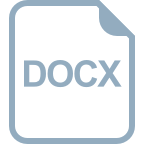
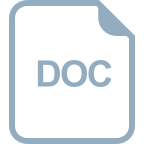
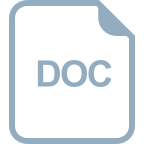
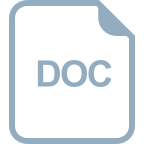
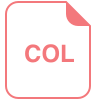
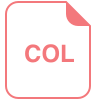



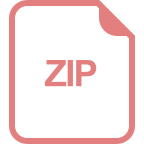