rtthread 操作系统下串口接收带校验位数据程序
时间: 2023-08-30 08:12:36 浏览: 66
下面是一个在 RT-Thread 操作系统下接收带校验位的串口数据的示例程序:
```c
#include <rtthread.h>
#include <rtdevice.h>
#define BUF_SIZE 64
static char recv_buf[BUF_SIZE];
static rt_event_t event;
/* 串口接收回调函数 */
static void uart_rx_callback(struct rt_serial_device *serial, rt_size_t size)
{
/* 接收数据 */
rt_device_read(serial, 0, recv_buf, size);
/* 发送事件,通知数据已经接收到 */
rt_event_send(&event, 0x01);
}
/* 串口接收线程 */
static void uart_rx_thread_entry(void *parameter)
{
rt_device_t uart_dev = (rt_device_t)parameter;
rt_err_t result;
int i;
while (1)
{
/* 等待数据接收事件 */
result = rt_event_recv(&event, 0x01, RT_EVENT_FLAG_OR | RT_EVENT_FLAG_CLEAR, RT_WAITING_FOREVER, RT_NULL);
if (result == RT_EOK)
{
/* 处理接收到的数据 */
for (i = 0; i < BUF_SIZE - 1; i++)
{
if (recv_buf[i] == '\n')
{
/* 校验数据 */
if (recv_buf[i - 1] == '0' && recv_buf[i - 2] == 'x')
{
/* 数据合法,处理数据 */
rt_kprintf("Received data: 0x%c%c\n", recv_buf[i - 3], recv_buf[i - 4]);
}
break;
}
}
}
}
}
/* 应用程序入口 */
int main(void)
{
rt_device_t uart_dev;
rt_err_t result;
/* 创建事件 */
result = rt_event_init(&event, "event", RT_IPC_FLAG_FIFO);
if (result != RT_EOK)
{
rt_kprintf("Failed to create event, code: %d\n", result);
return -1;
}
/* 查找串口设备 */
uart_dev = rt_device_find("uart1");
if (uart_dev == RT_NULL)
{
rt_kprintf("Failed to find uart1 device\n");
return -1;
}
/* 打开串口设备 */
result = rt_device_open(uart_dev, RT_DEVICE_OFLAG_RDWR | RT_DEVICE_FLAG_INT_RX);
if (result != RT_EOK)
{
rt_kprintf("Failed to open uart1 device, code: %d\n", result);
return -1;
}
/* 设置串口参数 */
rt_serial_control(uart_dev, RT_DEVICE_CTRL_CLR_ERRORS, RT_NULL);
rt_serial_control(uart_dev, RT_DEVICE_CTRL_CONFIG, (void *)"115200,N,8,1");
rt_serial_set_rx_indicate(uart_dev, uart_rx_callback);
/* 创建串口接收线程 */
rt_thread_t tid = rt_thread_create("uart_rx", uart_rx_thread_entry, uart_dev, 1024, 8, 20);
if (tid == RT_NULL)
{
rt_kprintf("Failed to create uart_rx thread\n");
return -1;
}
/* 启动串口接收线程 */
rt_thread_startup(tid);
return 0;
}
```
在此示例程序中,我们创建了一个事件用于通知主线程数据已经接收到;在串口接收回调函数中,我们使用 `rt_device_read()` 函数接收数据,并发送事件通知主线程数据已经接收到;在串口接收线程中,我们等待事件并处理接收到的数据,如果数据合法则进行处理。
注意,此示例程序中的校验方式为判断数据是否以 `0x` 开头并且后两位为校验位。如果需要使用其他校验方式,请根据实际情况修改代码。
相关推荐
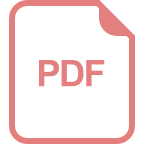
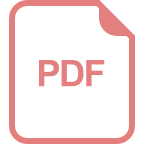














