#include"FastLED.h" #define NUM_LEDS 42 #define DATA_PIN 3 #define LED_TYPE WS2812 #define COLOR_ORDER GRB int value = 5; //起始亮度 int deltaHue = 230; //相邻灯珠色差 //uint8_t max_bright = value; CRGB leds[NUM_LEDS]; void setup(){ pinMode(DATA_PIN,OUTPUT); LEDS.addLeds<LED_TYPE, DATA_PIN, COLOR_ORDER>(leds, NUM_LEDS); //FastLED.setBrightness(max_bright); Serial.begin(115200); } void loop(){ for(; value < 50; value++){ FastLED.setBrightness(value); fill_rainbow(leds, 42, 10, deltaHue); FastLED.show(); Serial.println(value); delay(50); } for(; value > 5; value--){ FastLED.setBrightness(value); fill_rainbow(leds, 42, 10, deltaHue); FastLED.show(); Serial.println(value); delay(50); } delay(100); }
时间: 2024-04-06 22:33:10 浏览: 57
这段代码使用FastLED库控制一个WS2812类型的LED灯带。它定义了一些常量,如LED数量、数据引脚、颜色类型和颜色顺序。它还定义了一些变量,如起始亮度和相邻灯珠色差,以及一个CRGB数组`leds`,用于存储颜色数据。
在`setup()`函数中,它设置了数据引脚为输出模式,并将`leds`数组作为参数传递给`LEDS.addLeds()`函数,以告诉FastLED库如何控制LED灯带。此外,它还启动了串口通信,以便我们可以在串口监视器中查看调试信息。
在`loop()`函数中,它使用`for`循环逐渐增加亮度,然后逐渐减小亮度,以模拟呼吸灯效果。在每个循环中,它使用`FastLED.setBrightness()`函数设置LED灯带的亮度,使用`fill_rainbow()`函数填充颜色,然后使用`FastLED.show()`函数将颜色数据发送到LED灯带。它还使用`Serial.println()`函数将当前亮度值打印到串口监视器中,以便我们可以检查它是否按预期工作。最后,它使用`delay()`函数暂停一段时间,以控制呼吸灯效果的速度。
相关问题
#include <FastLED.h> #define LED_PIN 3 #define LED_COUNT 42 #define BRIGHTNESS 255 #define LED_TYPE WS2812B #define COLOR_ORDER GRB CRGB leds[LED_COUNT]; void setup() { FastLED.addLeds<LED_TYPE, LED_PIN, COLOR_ORDER>(leds, LED_COUNT); FastLED.setBrightness(BRIGHTNESS); }增加另一条灯带
您可以使用以下步骤来添加另一条灯带:
1.确定您将使用的灯带的数量和类型,以及它们所需的引脚。
2.在您的代码中定义第二个灯带的LED数量和引脚,以及要用于该灯带的FastLED控制器。例如:
```
#define LED_PIN_1 3
#define LED_COUNT_1 42
#define LED_PIN_2 5
#define LED_COUNT_2 30
CRGB leds_1[LED_COUNT_1];
CRGB leds_2[LED_COUNT_2];
FastLED.addLeds<LED_TYPE, LED_PIN_1, COLOR_ORDER>(leds_1, LED_COUNT_1);
FastLED.addLeds<LED_TYPE, LED_PIN_2, COLOR_ORDER>(leds_2, LED_COUNT_2);
```
3.编写代码以控制两个灯带。您可以使用类似以下代码的函数来控制每个灯带:
```
void setLeds1Color(CRGB color) {
// Set the color of the LEDs in the first strip
for (int i = 0; i < LED_COUNT_1; i++) {
leds_1[i] = color;
}
// Show the updated colors on the LEDs
FastLED.show();
}
void setLeds2Color(CRGB color) {
// Set the color of the LEDs in the second strip
for (int i = 0; i < LED_COUNT_2; i++) {
leds_2[i] = color;
}
// Show the updated colors on the LEDs
FastLED.show();
}
```
4.在您的主循环中,调用这些函数以更改灯带的颜色:
```
void loop() {
// Set the color of the first strip to red
setLeds1Color(CRGB::Red);
// Wait for 1 second
delay(1000);
// Set the color of the second strip to blue
setLeds2Color(CRGB::Blue);
// Wait for 1 second
delay(1000);
}
```
这将使第一条灯带变为红色,第二条灯带变为蓝色,然后重复此过程。您可以根据需要更改颜色和时间间隔。
#include <FastLED.h> #define LED_PIN_1 3 #define LED_PIN_2 6 #define LED_COUNT_1 53 #define LED_COUNT_2 1 #define BRIGHTNESS 255 #define LED_TYPE WS2812B #define COLOR_ORDER GRB CRGB leds_1[LED_COUNT_1]; CRGB leds_2[LED_COUNT_2]; int pin_switch = 10; // 定义数字输入口pin10接收开关状态 int switch_state = 0; // 定义开关状态变量 void setup() { FastLED.addLeds<LED_TYPE, LED_PIN_1, COLOR_ORDER>(leds_1, LED_COUNT_1); FastLED.addLeds<LED_TYPE, LED_PIN_2, COLOR_ORDER>(leds_2, LED_COUNT_2); FastLED.setBrightness(BRIGHTNESS); Serial.begin(9600);//设置串口波特率为9600kbps pinMode(pin_switch, INPUT); // 初始化数字输入口 }第二条灯带慢慢点亮为黄色
您可以使用以下代码实现第二条灯带慢慢点亮为黄色:
```
void loop() {
switch_state = digitalRead(pin_switch); // 读取开关状态
if (switch_state == HIGH) { // 如果开关打开
for (int i = 0; i <= 255; i++) { // 从0渐变到255
leds_2[0] = CHSV(60, 255, i); // 设置黄色
FastLED.show(); // 更新LED灯带状态
delay(10); // 延时10毫秒
}
}
}
```
在主循环中,我们首先读取开关的状态,当开关打开时,我们使用一个循环从0渐变到255,每一次都设置第二条灯带的颜色为黄色并更新灯带状态,同时延时10毫秒,使得渐变效果更加平滑。
阅读全文
相关推荐
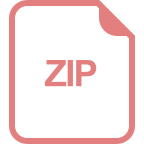
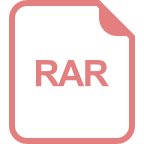
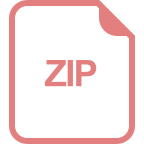


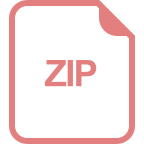
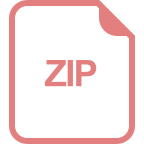
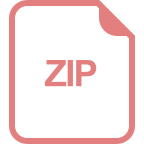
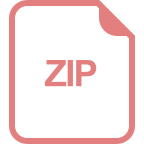
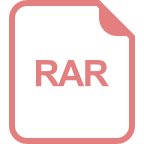
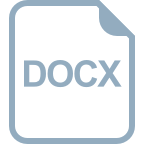
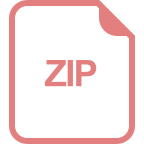
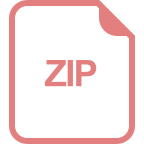
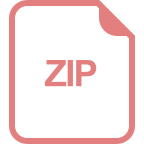