怎么在摄像头上利用opencv进行透视矫正
时间: 2024-05-08 15:16:46 浏览: 119
要在摄像头上利用OpenCV进行透视矫正,可以按照以下步骤进行:
1. 读取摄像头的视频流,可以使用cv2.VideoCapture(0)函数打开默认的摄像头。
2. 通过cv2.namedWindow()函数创建一个窗口,用于显示矫正后的图像。
3. 通过cv2.setMouseCallback()函数设置鼠标回调函数,以便在图像上选择四个点,这四个点将用于定义透视变换。
4. 根据选择的四个点计算透视变换矩阵,可以使用cv2.getPerspectiveTransform()函数。
5. 应用透视变换矩阵,将原始图像进行矫正,可以使用cv2.warpPerspective()函数。
6. 在窗口中显示矫正后的图像,可以使用cv2.imshow()函数。
7. 使用cv2.waitKey()函数等待用户按下键盘上的某个键,以便退出程序。
下面是一个示例代码:
```
import cv2
import numpy as np
def mouse_callback(event, x, y, flags, params):
if event == cv2.EVENT_LBUTTONDOWN:
if len(params['points']) < 4:
params['points'].append((x, y))
cap = cv2.VideoCapture(0)
cv2.namedWindow('Perspective Correction')
cv2.setMouseCallback('Perspective Correction', mouse_callback, {'points': []})
while True:
ret, frame = cap.read()
if len(params['points']) == 4:
src_points = np.float32(params['points'])
dst_points = np.float32([[0, 0], [frame.shape[1], 0], [frame.shape[1], frame.shape[0]], [0, frame.shape[0]]])
M = cv2.getPerspectiveTransform(src_points, dst_points)
corrected_frame = cv2.warpPerspective(frame, M, (frame.shape[1], frame.shape[0]))
cv2.imshow('Perspective Correction', corrected_frame)
else:
cv2.imshow('Perspective Correction', frame)
if cv2.waitKey(1) == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
```
在运行代码时,可以在打开的窗口中用鼠标选择四个点,然后程序会自动进行透视矫正,最终显示矫正后的图像。如果想退出程序,可以按下键盘上的“q”键。
阅读全文
相关推荐
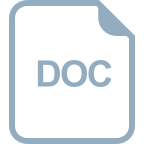
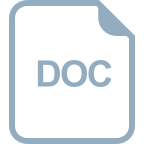
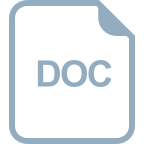




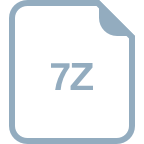
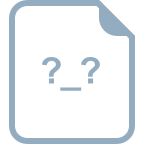
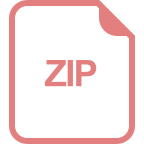
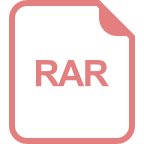
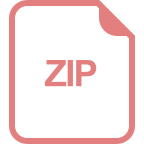
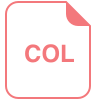
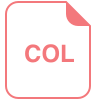
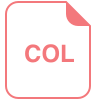
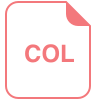
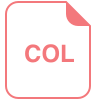
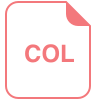
