#include <stdio.h> #include <stdlib.h> #include <time.h> // 定义员工结构体 struct Employee { int id; // 员工编号 char name[20]; // 员工姓名 char gender[5]; // 员工性别 int age; // 员工年龄 int performance; // 员工业绩 char contact[20]; // 员工联系方式 }; // 打印员工信息 void printEmployee(struct Employee *e) { printf("%d\t %s\t %s\t %d\t %d\t %s\n", e->id, e->name, e->gender, e->age, e->performance, e->contact); } int main() { int n; // 员工数量 printf("请输入员工数量:"); scanf("%d", &n); struct Employee employees[n]; for (int i = 0; i < n; i++) { printf("请输入第 %d 位员工的信息:\n", i+1); printf("\t编号:"); scanf("%d", &(employees[i].id)); printf("\t姓名:"); scanf("%s", employees[i].name); printf("\t性别:"); scanf("%s", employees[i].gender); printf("\t年龄:"); scanf("%d", &(employees[i].age)); printf("\t业绩:"); scanf("%d", &(employees[i].performance)); printf("\t联系方式:"); scanf("%s", employees[i].contact); } // 随机分组 int groupNum = n / 5; // 小组数量 srand(time(NULL)); // 设置随机种子为当前时间 printf("本次共分为 %d 个小组,各组员工信息如下:\n", groupNum); for (int i = 0; i < groupNum; i++) { printf("第 %d 个小组:\n", i+1); for (int j = 0; j < 5; j++) { int k = rand() % n; printEmployee(&employees[k]); } if (n % 5 != 0 && i == groupNum - 1) { // 处理剩余的员工 for (int j = 0; j < n % 5; j++) { int k = rand() % n; printEmployee(&employees[k]); } } } return 0; }帮我删除注释,并更换数组名为简单的名字
时间: 2024-04-01 13:37:08 浏览: 129
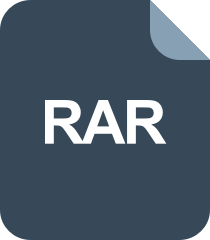
C语言头文件 LIST.H
下面是删除注释并更换数组名为简单的名字后的代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
struct Employee {
int id;
char name[20];
char gender[5];
int age;
int performance;
char contact[20];
};
void printEmployee(struct Employee *e) {
printf("%d\t %s\t %s\t %d\t %d\t %s\n", e->id, e->name, e->gender, e->age, e->performance, e->contact);
}
int main() {
int n;
printf("请输入员工数量:");
scanf("%d", &n);
struct Employee emp[n];
for (int i = 0; i < n; i++) {
printf("请输入第 %d 位员工的信息:\n", i+1);
printf("\t编号:");
scanf("%d", &(emp[i].id));
printf("\t姓名:");
scanf("%s", emp[i].name);
printf("\t性别:");
scanf("%s", emp[i].gender);
printf("\t年龄:");
scanf("%d", &(emp[i].age));
printf("\t业绩:");
scanf("%d", &(emp[i].performance));
printf("\t联系方式:");
scanf("%s", emp[i].contact);
}
int groupNum = n / 5;
srand(time(NULL));
printf("本次共分为 %d 个小组,各组员工信息如下:\n", groupNum);
for (int i = 0; i < groupNum; i++) {
printf("第 %d 个小组:\n", i+1);
for (int j = 0; j < 5; j++) {
int k = rand() % n;
printEmployee(&emp[k]);
}
if (n % 5 != 0 && i == groupNum - 1) {
for (int j = 0; j < n % 5; j++) {
int k = rand() % n;
printEmployee(&emp[k]);
}
}
}
return 0;
}
```
阅读全文
相关推荐
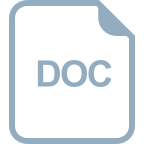
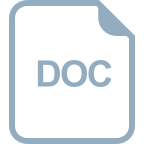
















