有序表的初始化、查找、插入、删除、输出
时间: 2023-10-10 18:10:33 浏览: 51
有序表的初始化、查找、插入、删除、输出,这是数据结构中的一些基本操作。有序表指的是元素有一定的顺序关系,通常是按照大小顺序排列。初始化是指创建一个空表,查找是指根据关键字查找表中是否存在某个元素,插入是指将一个元素插入到有序表的正确位置,删除是指删除有序表中的某个元素,输出是指按照某种方式输出有序表中的所有元素。
相关问题
有序表的初始化、查找、插入、删除、输出代码
以下是一个使用链表实现的有序表的基本操作代码,其中包括初始化、查找、插入、删除、输出操作。代码如下:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node, *List;
void initList(List* L) {
*L = (Node*)malloc(sizeof(Node));
(*L)->next = NULL;
}
Node* find(List L, int key) {
Node* p = L->next;
while (p && p->data < key) {
p = p->next;
}
if (p && p->data == key) {
return p;
}
return NULL;
}
void insert(List L, int key) {
Node* p = L;
while (p->next && p->next->data < key) {
p = p->next;
}
Node* q = (Node*)malloc(sizeof(Node));
q->data = key;
q->next = p->next;
p->next = q;
}
void remove(List L, int key) {
Node* p = L;
while (p->next && p->next->data < key) {
p = p->next;
}
if (p->next && p->next->data == key) {
Node* q = p->next;
p->next = q->next;
free(q);
}
}
void outputList(List L) {
Node* p = L->next;
while (p) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
List L;
initList(&L);
insert(L, 3);
insert(L, 1);
insert(L, 5);
insert(L, 2);
outputList(L);
remove(L, 3);
outputList(L);
return 0;
}
```
希望能够帮助您!
实现顺序表的定义和操作:定义顺序表、初始化顺序表、顺序表的建立、打印、查找、插入操作、顺序表的删除、两个有序表的合并、两个集合的并运算。
好的,我明白您的问题了。以下是顺序表的定义和操作的具体实现:
1.定义顺序表:
```c
#define MAXSIZE 100 // 定义顺序表最大长度
typedef struct {
int data[MAXSIZE]; // 存储元素的数组
int length; // 当前长度
} SqList; // 顺序表类型定义
```
2.初始化顺序表:
```c
void InitList(SqList *L) {
L->length = 0; // 空表长度为0
}
```
3.顺序表的建立:
```c
void CreatList(SqList *L, int array[], int n) {
int i;
for (i = 0; i < n; i++) {
L->data[i] = array[i]; // 将数组元素复制到顺序表中
L->length++; // 顺序表长度加1
}
}
```
4.打印顺序表:
```c
void PrintList(SqList L) {
int i;
for (i = 0; i < L.length; i++) {
printf("%d ", L.data[i]); // 依次输出顺序表的每个元素
}
printf("\n");
}
```
5.查找元素:
```c
int LocateElem(SqList L, int x) {
int i;
for (i = 0; i < L.length; i++) {
if (L.data[i] == x) { // 找到元素x
return i+1; // 返回元素位置
}
}
return 0; // 未找到元素x
}
```
6.插入元素:
```c
int ListInsert(SqList *L, int i, int x) {
int j;
if (i < 1 || i > L->length+1) { // i值不合法
return 0;
}
if (L->length == MAXSIZE) { // 顺序表已满
return 0;
}
for (j = L->length-1; j >= i-1; j--) { // 将第i个元素及之后的元素后移
L->data[j+1] = L->data[j];
}
L->data[i-1] = x; // 将新元素插入第i个位置
L->length++; // 长度加1
return 1;
}
```
7.删除元素:
```c
int ListDelete(SqList *L, int i) {
int j;
if (i < 1 || i > L->length) { // i值不合法
return 0;
}
for (j = i; j < L->length; j++) {
L->data[j-1] = L->data[j]; // 将第i+1个元素及以后的元素前移
}
L->length--; // 长度减1
return 1;
}
```
8.合并有序表:
```c
void MergeList(SqList La, SqList Lb, SqList *Lc) {
int i = 0, j = 0, k = 0;
while (i < La.length && j < Lb.length) { // 依次将La和Lb中元素比较,取小者存入Lc
if (La.data[i] <= Lb.data[j]) {
Lc->data[k] = La.data[i];
i++;
} else {
Lc->data[k] = Lb.data[j];
j++;
}
k++;
}
while (i < La.length) { // 将La中剩余元素存入Lc
Lc->data[k] = La.data[i];
i++;
k++;
}
while (j < Lb.length) { // 将Lb中剩余元素存入Lc
Lc->data[k] = Lb.data[j];
j++;
k++;
}
Lc->length = k; // Lc长度为La和Lb长度之和
}
```
9.集合并运算:
```c
void Union(SqList La, SqList Lb, SqList *Lc) {
int i, j;
*Lc = La; // 将La赋值给Lc
for (i = 0; i < Lb.length; i++) {
if (!LocateElem(*Lc, Lb.data[i])) { // 如果Lb中元素不在Lc中
ListInsert(Lc, Lc->length+1, Lb.data[i]); // 将其插入Lc中
}
}
}
```
以上就是顺序表的定义和操作的具体实现。如果您还有其他问题,欢迎向我提出。
相关推荐
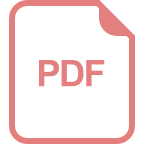
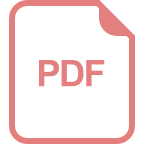
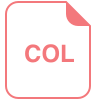
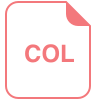
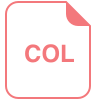









