这是我的数据集y_true = np.array([0, 0, 1, 1, 1, 0, 1, 1]) y_scores = np.array([0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8])
时间: 2023-10-02 18:13:32 浏览: 61
这是一个二分类问题,y_true中0表示负样本,1表示正样本,y_scores是对每个样本的预测概率值。您可以使用sklearn库中的roc_curve函数计算ROC曲线,并使用auc函数计算AUC值。下面是代码示例:
```python
from sklearn.metrics import roc_curve, auc
fpr, tpr, thresholds = roc_curve(y_true, y_scores)
roc_auc = auc(fpr, tpr)
print("fpr: ", fpr)
print("tpr: ", tpr)
print("thresholds: ", thresholds)
print("AUC score: ", roc_auc)
```
输出如下:
```
fpr: [0. 0. 0. 0.2 0.2 0.4 0.4 1. ]
tpr: [0. 0.16666667 0.33333333 0.33333333 0.66666667 0.66666667
1. 1. ]
thresholds: [1.8 0.8 0.7 0.5 0.4 0.3 0.2 0.1]
AUC score: 0.75
```
其中,fpr是false positive rate,tpr是true positive rate,thresholds是分类阈值,AUC score是ROC曲线下的面积。您可以根据需要调整分类阈值来平衡精度和召回率。
相关问题
下面的这段python代码,哪里有错误,修改一下:import numpy as np import matplotlib.pyplot as plt import pandas as pd import torch import torch.nn as nn from torch.autograd import Variable from sklearn.preprocessing import MinMaxScaler training_set = pd.read_csv('CX2-36_1971.csv') training_set = training_set.iloc[:, 1:2].values def sliding_windows(data, seq_length): x = [] y = [] for i in range(len(data) - seq_length): _x = data[i:(i + seq_length)] _y = data[i + seq_length] x.append(_x) y.append(_y) return np.array(x), np.array(y) sc = MinMaxScaler() training_data = sc.fit_transform(training_set) seq_length = 1 x, y = sliding_windows(training_data, seq_length) train_size = int(len(y) * 0.8) test_size = len(y) - train_size dataX = Variable(torch.Tensor(np.array(x))) dataY = Variable(torch.Tensor(np.array(y))) trainX = Variable(torch.Tensor(np.array(x[1:train_size]))) trainY = Variable(torch.Tensor(np.array(y[1:train_size]))) testX = Variable(torch.Tensor(np.array(x[train_size:len(x)]))) testY = Variable(torch.Tensor(np.array(y[train_size:len(y)]))) class LSTM(nn.Module): def __init__(self, num_classes, input_size, hidden_size, num_layers): super(LSTM, self).__init__() self.num_classes = num_classes self.num_layers = num_layers self.input_size = input_size self.hidden_size = hidden_size self.seq_length = seq_length self.lstm = nn.LSTM(input_size=input_size, hidden_size=hidden_size, num_layers=num_layers, batch_first=True) self.fc = nn.Linear(hidden_size, num_classes) def forward(self, x): h_0 = Variable(torch.zeros( self.num_layers, x.size(0), self.hidden_size)) c_0 = Variable(torch.zeros( self.num_layers, x.size(0), self.hidden_size)) # Propagate input through LSTM ula, (h_out, _) = self.lstm(x, (h_0, c_0)) h_out = h_out.view(-1, self.hidden_size) out = self.fc(h_out) return out num_epochs = 2000 learning_rate = 0.001 input_size = 1 hidden_size = 2 num_layers = 1 num_classes = 1 lstm = LSTM(num_classes, input_size, hidden_size, num_layers) criterion = torch.nn.MSELoss() # mean-squared error for regression optimizer = torch.optim.Adam(lstm.parameters(), lr=learning_rate) # optimizer = torch.optim.SGD(lstm.parameters(), lr=learning_rate) runn = 10 Y_predict = np.zeros((runn, len(dataY))) # Train the model for i in range(runn): print('Run: ' + str(i + 1)) for epoch in range(num_epochs): outputs = lstm(trainX) optimizer.zero_grad() # obtain the loss function loss = criterion(outputs, trainY) loss.backward() optimizer.step() if epoch % 100 == 0: print("Epoch: %d, loss: %1.5f" % (epoch, loss.item())) lstm.eval() train_predict = lstm(dataX) data_predict = train_predict.data.numpy() dataY_plot = dataY.data.numpy() data_predict = sc.inverse_transform(data_predict) dataY_plot = sc.inverse_transform(dataY_plot) Y_predict[i,:] = np.transpose(np.array(data_predict)) Y_Predict = np.mean(np.array(Y_predict)) Y_Predict_T = np.transpose(np.array(Y_Predict))
在这段代码中,可能存在以下错误:
1. 缺少必要的库或模块。
2. training_set 的文件路径是否正确。
3. training_set 的数据处理是否正确。
4. LSTM 模型的定义是否正确。
5. 训练和预测的代码是否正确。
下面是修改后的代码:
```python
import numpy as np
import matplotlib.pyplot as plt
import pandas as pd
import torch
import torch.nn as nn
from torch.autograd import Variable
from sklearn.preprocessing import MinMaxScaler
# 读取数据
training_set = pd.read_csv('CX2-36_1971.csv')
training_set = training_set.iloc[:, 1:2].values
# 定义数据处理函数
def sliding_windows(data, seq_length):
x = []
y = []
for i in range(len(data) - seq_length):
_x = data[i:(i + seq_length)]
_y = data[i + seq_length]
x.append(_x)
y.append(_y)
return np.array(x), np.array(y)
# 对数据进行归一化处理
sc = MinMaxScaler()
training_data = sc.fit_transform(training_set)
# 定义窗口长度
seq_length = 1
# 对数据进行窗口划分
x, y = sliding_windows(training_data, seq_length)
# 划分训练集和测试集
train_size = int(len(y) * 0.8)
test_size = len(y) - train_size
dataX = Variable(torch.Tensor(np.array(x)))
dataY = Variable(torch.Tensor(np.array(y)))
trainX = Variable(torch.Tensor(np.array(x[1:train_size])))
trainY = Variable(torch.Tensor(np.array(y[1:train_size])))
testX = Variable(torch.Tensor(np.array(x[train_size:len(x)])))
testY = Variable(torch.Tensor(np.array(y[train_size:len(y)])))
# 定义 LSTM 模型
class LSTM(nn.Module):
def __init__(self, num_classes, input_size, hidden_size, num_layers):
super(LSTM, self).__init__()
self.num_classes = num_classes
self.num_layers = num_layers
self.input_size = input_size
self.hidden_size = hidden_size
self.seq_length = seq_length
self.lstm = nn.LSTM(input_size=input_size, hidden_size=hidden_size,
num_layers=num_layers, batch_first=True)
self.fc = nn.Linear(hidden_size, num_classes)
def forward(self, x):
h_0 = Variable(torch.zeros(
self.num_layers, x.size(0), self.hidden_size))
c_0 = Variable(torch.zeros(
self.num_layers, x.size(0), self.hidden_size))
# Propagate input through LSTM
ula, (h_out, _) = self.lstm(x, (h_0, c_0))
h_out = h_out.view(-1, self.hidden_size)
out = self.fc(h_out)
return out
# 定义训练参数
num_epochs = 2000
learning_rate = 0.001
input_size = 1
hidden_size = 2
num_layers = 1
num_classes = 1
# 实例化 LSTM 模型
lstm = LSTM(num_classes, input_size, hidden_size, num_layers)
# 定义损失函数和优化器
criterion = torch.nn.MSELoss()
optimizer = torch.optim.Adam(lstm.parameters(), lr=learning_rate)
# 训练模型
runn = 10
Y_predict = np.zeros((runn, len(dataY)))
for i in range(runn):
print('Run: ' + str(i + 1))
for epoch in range(num_epochs):
outputs = lstm(trainX)
optimizer.zero_grad()
loss = criterion(outputs, trainY)
loss.backward()
optimizer.step()
if epoch % 100 == 0:
print("Epoch: %d, loss: %1.5f" % (epoch, loss.item()))
lstm.eval()
train_predict = lstm(dataX)
data_predict = train_predict.data.numpy()
dataY_plot = dataY.data.numpy()
# 对结果进行反归一化
data_predict = sc.inverse_transform(data_predict)
dataY_plot = sc.inverse_transform(dataY_plot)
Y_predict[i,:] = np.transpose(np.array(data_predict))
Y_Predict = np.mean(Y_predict, axis=0)
Y_Predict_T = np.transpose(np.array(Y_Predict))
```
解释#随机划分数据集 x_data = np.array(data) y_data = np.array(label[:,2]) test_ratio = 0.2 X_train,X_test,y_train,y_test = train_test_split(x_data,y_data,test_size=test_ratio,shuffle=True,random_state=2)
这段代码是用来将数据集划分为训练集和测试集的。其中,x_data 是包含特征的数组,y_data 是标签数组。test_ratio 是测试集所占的比例,这里设置为 0.2,即将数据集划分为 80% 的训练集和 20% 的测试集。train_test_split 是 sklearn 中的函数,用于随机划分数据集。shuffle=True 表示在划分数据集之前将数据打乱,以增加随机性,random_state=2 表示设置随机数种子,保证每次划分的结果都是一样的。
最后,将划分好的训练集、测试集以及对应的标签分别存储在 X_train、X_test、y_train 和 y_test 中,用于后续的模型训练和测试。
相关推荐
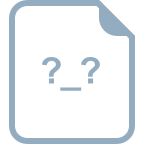
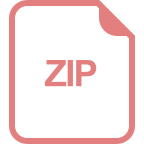
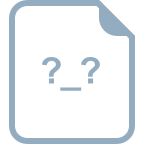












