mybatis xml查询条件数组
时间: 2023-09-30 14:03:39 浏览: 113
在 Mybatis 的 XML 配置中,可以使用动态 SQL 标签实现查询条件数组的传递。以下是一个示例:
```xml
<select id="selectByExample" resultMap="BaseResultMap" parameterType="com.example.entity.Example">
SELECT
<include refid="Base_Column_List" />
FROM
example_table
<where>
<foreach collection="criteria" item="criterion" separator="and">
<if test="criterion.valid">
${criterion.condition}
</if>
</foreach>
</where>
</select>
```
在这个示例中,`criteria` 是一个包含查询条件的数组,每个条件都有一个 `valid` 属性用于判断该条件是否生效。`<foreach>` 标签用于循环遍历 `criteria` 数组中的每个条件,将有效的条件的 SQL 语句拼接到 `<where>` 标签中形成完整的 SQL 语句。需要注意的是,`<foreach>` 标签中的 `separator` 属性用于指定条件之间的连接符,这里使用的是 `and`。
相关问题
mybatis中动态查询数组条件
在 MyBatis 中,你可以使用动态 SQL 来构建包含数组条件的查询语句。以下是一种常见的方法:
1. 在你的 XML 映射文件中,使用 `<foreach>` 元素来遍历数组,并构建条件语句。示例代码如下:
```xml
<select id="selectByArrayCondition" resultType="YourResultType">
SELECT *
FROM your_table
WHERE id IN
<foreach item="item" index="index" collection="ids" open="(" separator="," close=")">
#{item}
</foreach>
</select>
```
在上面的示例中,`ids` 是传递给查询的数组参数,`your_table` 是你要查询的表名,`YourResultType` 是查询结果的类型。
2. 在你的 Mapper 接口中定义对应的方法:
```java
public interface YourMapper {
List<YourResultType> selectByArrayCondition(@Param("ids") Integer[] ids);
}
```
在上面的示例中,`ids` 参数用于传递数组条件。
3. 调用查询方法时,传递包含数组条件的参数。例如:
```java
Integer[] ids = {1, 2, 3};
List<YourResultType> results = yourMapper.selectByArrayCondition(ids);
```
这样,MyBatis 将会根据传递的数组参数构建相应的查询语句,并返回符合条件的结果集。
请注意,上述示例中仅展示了一种处理数组条件的方法。根据具体需求,你可能需要根据不同的情况进行适当的调整。
mybatis xml结构体数组查询
可以使用 MyBatis 的 foreach 标签来处理结构体数组查询。
假设有一个结构体数组:
```java
public class User {
private int id;
private String name;
// getters and setters
}
```
可以在 MyBatis 的 XML 文件中编写如下 SQL:
```xml
<select id="getUserList" resultType="User">
SELECT * FROM user WHERE id IN
<foreach collection="userList" item="user" open="(" separator="," close=")">
#{user.id}
</foreach>
</select>
```
其中,`collection` 属性指定结构体数组的名称,`item` 属性指定结构体数组中的元素的名称,`open` 属性指定数组元素的开头字符,`separator` 属性指定数组元素之间的分隔符,`close` 属性指定数组元素的结尾字符。
在 Java 代码中,通过传递一个名为 `userList` 的结构体数组,可以调用该 SQL:
```java
List<User> userList = new ArrayList<>();
userList.add(new User(1, "Alice"));
userList.add(new User(2, "Bob"));
List<User> result = sqlSession.selectList("getUserList", userList);
```
这样就可以查询出 id 为 1 和 2 的用户信息。
阅读全文
相关推荐
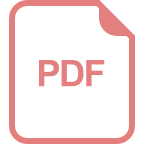












