结合C++类与对象和数据库对体育器材进行管理,分为器材的借用归还,报废新购四为了方便平时管理器材,还需要设计一个体育器材管理功能模块,可以随时浏览器材的各方面信息,比如库存情况、器材新购价格和供应商(品牌)新购器材时,需要对器材进行信息录入,所以还需要添加一个信息录入功能模块,方便管理员对器材库存信息进行更新。除此之外,为了保证用户信息的准确性以及实时性,添加一个个人信息功能模块,方便随时修改用户信息,为系统的安全性提供了一些保障。请构建代码
时间: 2023-06-15 09:04:48 浏览: 36
以下是一个简单的体育器材管理系统的代码示例,包含了器材借用归还、报废新购、库存信息浏览、信息录入、个人信息等功能模块。该示例使用C++语言实现,使用SQLite数据库来存储数据。
```cpp
#include <iostream>
#include <string>
#include <vector>
#include "sqlite3.h" // SQLite数据库头文件
using namespace std;
// 定义器材类
class Equipment {
public:
Equipment(string name, string brand, int quantity, double price) {
this->name = name;
this->brand = brand;
this->quantity = quantity;
this->price = price;
}
string getName() {
return name;
}
string getBrand() {
return brand;
}
int getQuantity() {
return quantity;
}
double getPrice() {
return price;
}
private:
string name; // 器材名称
string brand; // 品牌
int quantity; // 库存数量
double price; // 新购价格
};
// 定义管理系统类
class ManagementSystem {
public:
ManagementSystem() {
// 连接数据库
int rc = sqlite3_open("equipment.db", &db);
if (rc != SQLITE_OK) {
cerr << "Cannot open database: " << sqlite3_errmsg(db) << endl;
exit(1);
}
// 创建表格
string sql = "CREATE TABLE IF NOT EXISTS equipment (name TEXT PRIMARY KEY, brand TEXT, quantity INTEGER, price REAL);";
rc = sqlite3_exec(db, sql.c_str(), NULL, NULL, NULL);
if (rc != SQLITE_OK) {
cerr << "Cannot create table: " << sqlite3_errmsg(db) << endl;
exit(1);
}
}
~ManagementSystem() {
// 关闭数据库
sqlite3_close(db);
}
// 器材借用
void borrowEquipment(string name, int quantity) {
sqlite3_stmt* stmt;
string sql = "SELECT quantity FROM equipment WHERE name=?;";
int rc = sqlite3_prepare_v2(db, sql.c_str(), -1, &stmt, NULL);
if (rc != SQLITE_OK) {
cerr << "Cannot prepare statement: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_bind_text(stmt, 1, name.c_str(), -1, SQLITE_STATIC);
if (rc != SQLITE_OK) {
cerr << "Cannot bind parameter: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_step(stmt);
if (rc == SQLITE_ROW) {
int currentQuantity = sqlite3_column_int(stmt, 0);
if (currentQuantity >= quantity) {
sql = "UPDATE equipment SET quantity=? WHERE name=?;";
rc = sqlite3_prepare_v2(db, sql.c_str(), -1, &stmt, NULL);
if (rc != SQLITE_OK) {
cerr << "Cannot prepare statement: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_bind_int(stmt, 1, currentQuantity - quantity);
if (rc != SQLITE_OK) {
cerr << "Cannot bind parameter: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_bind_text(stmt, 2, name.c_str(), -1, SQLITE_STATIC);
if (rc != SQLITE_OK) {
cerr << "Cannot bind parameter: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_step(stmt);
if (rc != SQLITE_DONE) {
cerr << "Cannot execute statement: " << sqlite3_errmsg(db) << endl;
return;
}
cout << "Borrow " << quantity << " " << name << " successfully." << endl;
} else {
cout << "Not enough " << name << " in stock." << endl;
}
} else {
cout << "Cannot find " << name << "." << endl;
}
sqlite3_finalize(stmt);
}
// 器材归还
void returnEquipment(string name, int quantity) {
sqlite3_stmt* stmt;
string sql = "SELECT quantity FROM equipment WHERE name=?;";
int rc = sqlite3_prepare_v2(db, sql.c_str(), -1, &stmt, NULL);
if (rc != SQLITE_OK) {
cerr << "Cannot prepare statement: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_bind_text(stmt, 1, name.c_str(), -1, SQLITE_STATIC);
if (rc != SQLITE_OK) {
cerr << "Cannot bind parameter: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_step(stmt);
if (rc == SQLITE_ROW) {
int currentQuantity = sqlite3_column_int(stmt, 0);
sql = "UPDATE equipment SET quantity=? WHERE name=?;";
rc = sqlite3_prepare_v2(db, sql.c_str(), -1, &stmt, NULL);
if (rc != SQLITE_OK) {
cerr << "Cannot prepare statement: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_bind_int(stmt, 1, currentQuantity + quantity);
if (rc != SQLITE_OK) {
cerr << "Cannot bind parameter: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_bind_text(stmt, 2, name.c_str(), -1, SQLITE_STATIC);
if (rc != SQLITE_OK) {
cerr << "Cannot bind parameter: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_step(stmt);
if (rc != SQLITE_DONE) {
cerr << "Cannot execute statement: " << sqlite3_errmsg(db) << endl;
return;
}
cout << "Return " << quantity << " " << name << " successfully." << endl;
} else {
cout << "Cannot find " << name << "." << endl;
}
sqlite3_finalize(stmt);
}
// 报废器材
void scrapEquipment(string name) {
sqlite3_stmt* stmt;
string sql = "DELETE FROM equipment WHERE name=?;";
int rc = sqlite3_prepare_v2(db, sql.c_str(), -1, &stmt, NULL);
if (rc != SQLITE_OK) {
cerr << "Cannot prepare statement: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_bind_text(stmt, 1, name.c_str(), -1, SQLITE_STATIC);
if (rc != SQLITE_OK) {
cerr << "Cannot bind parameter: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_step(stmt);
if (rc != SQLITE_DONE) {
cerr << "Cannot execute statement: " << sqlite3_errmsg(db) << endl;
return;
}
cout << "Scrap " << name << " successfully." << endl;
sqlite3_finalize(stmt);
}
// 新购器材
void purchaseEquipment(string name, string brand, int quantity, double price) {
sqlite3_stmt* stmt;
string sql = "INSERT INTO equipment (name, brand, quantity, price) VALUES (?, ?, ?, ?);";
int rc = sqlite3_prepare_v2(db, sql.c_str(), -1, &stmt, NULL);
if (rc != SQLITE_OK) {
cerr << "Cannot prepare statement: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_bind_text(stmt, 1, name.c_str(), -1, SQLITE_STATIC);
if (rc != SQLITE_OK) {
cerr << "Cannot bind parameter: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_bind_text(stmt, 2, brand.c_str(), -1, SQLITE_STATIC);
if (rc != SQLITE_OK) {
cerr << "Cannot bind parameter: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_bind_int(stmt, 3, quantity);
if (rc != SQLITE_OK) {
cerr << "Cannot bind parameter: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_bind_double(stmt, 4, price);
if (rc != SQLITE_OK) {
cerr << "Cannot bind parameter: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_step(stmt);
if (rc != SQLITE_DONE) {
cerr << "Cannot execute statement: " << sqlite3_errmsg(db) << endl;
return;
}
cout << "Purchase " << quantity << " " << name << " successfully." << endl;
sqlite3_finalize(stmt);
}
// 浏览器材库存信息
void viewInventory() {
sqlite3_stmt* stmt;
string sql = "SELECT name, brand, quantity, price FROM equipment;";
int rc = sqlite3_prepare_v2(db, sql.c_str(), -1, &stmt, NULL);
if (rc != SQLITE_OK) {
cerr << "Cannot prepare statement: " << sqlite3_errmsg(db) << endl;
return;
}
cout << "Name\tBrand\tQuantity\tPrice" << endl;
while ((rc = sqlite3_step(stmt)) == SQLITE_ROW) {
string name = (char*)sqlite3_column_text(stmt, 0);
string brand = (char*)sqlite3_column_text(stmt, 1);
int quantity = sqlite3_column_int(stmt, 2);
double price = sqlite3_column_double(stmt, 3);
cout << name << "\t" << brand << "\t" << quantity << "\t\t" << price << endl;
}
if (rc != SQLITE_DONE) {
cerr << "Cannot execute statement: " << sqlite3_errmsg(db) << endl;
return;
}
sqlite3_finalize(stmt);
}
// 个人信息管理
void managePersonalInfo() {
cout << "Please enter your name: ";
string name;
cin >> name;
sqlite3_stmt* stmt;
string sql = "SELECT age, gender, phone FROM personal_info WHERE name=?;";
int rc = sqlite3_prepare_v2(db, sql.c_str(), -1, &stmt, NULL);
if (rc != SQLITE_OK) {
cerr << "Cannot prepare statement: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_bind_text(stmt, 1, name.c_str(), -1, SQLITE_STATIC);
if (rc != SQLITE_OK) {
cerr << "Cannot bind parameter: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_step(stmt);
if (rc == SQLITE_ROW) {
int age = sqlite3_column_int(stmt, 0);
string gender = (char*)sqlite3_column_text(stmt, 1);
string phone = (char*)sqlite3_column_text(stmt, 2);
cout << "Your age is " << age << "." << endl;
cout << "Your gender is " << gender << "." << endl;
cout << "Your phone number is " << phone << "." << endl;
cout << "Do you want to update your information? (Y/N): ";
char choice;
cin >> choice;
if (choice == 'Y' || choice == 'y') {
cout << "Please enter your age: ";
int newAge;
cin >> newAge;
cout << "Please enter your gender: ";
string newGender;
cin >> newGender;
cout << "Please enter your phone number: ";
string newPhone;
cin >> newPhone;
sql = "UPDATE personal_info SET age=?, gender=?, phone=? WHERE name=?;";
rc = sqlite3_prepare_v2(db, sql.c_str(), -1, &stmt, NULL);
if (rc != SQLITE_OK) {
cerr << "Cannot prepare statement: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_bind_int(stmt, 1, newAge);
if (rc != SQLITE_OK) {
cerr << "Cannot bind parameter: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_bind_text(stmt, 2, newGender.c_str(), -1, SQLITE_STATIC);
if (rc != SQLITE_OK) {
cerr << "Cannot bind parameter: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_bind_text(stmt, 3, newPhone.c_str(), -1, SQLITE_STATIC);
if (rc != SQLITE_OK) {
cerr << "Cannot bind parameter: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_bind_text(stmt, 4, name.c_str(), -1, SQLITE_STATIC);
if (rc != SQLITE_OK) {
cerr << "Cannot bind parameter: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_step(stmt);
if (rc != SQLITE_DONE) {
cerr << "Cannot execute statement: " << sqlite3_errmsg(db) << endl;
return;
}
cout << "Update personal information successfully." << endl;
}
} else {
cout << "Cannot find your information." << endl;
}
sqlite3_finalize(stmt);
}
// 添加新用户
void addNewUser() {
cout << "Please enter your name: ";
string name;
cin >> name;
cout << "Please enter your age: ";
int age;
cin >> age;
cout << "Please enter your gender: ";
string gender;
cin >> gender;
cout << "Please enter your phone number: ";
string phone;
cin >> phone;
sqlite3_stmt* stmt;
string sql = "INSERT INTO personal_info (name, age, gender, phone) VALUES (?, ?, ?, ?);";
int rc = sqlite3_prepare_v2(db, sql.c_str(), -1, &stmt, NULL);
if (rc != SQLITE_OK) {
cerr << "Cannot prepare statement: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_bind_text(stmt, 1, name.c_str(), -1, SQLITE_STATIC);
if (rc != SQLITE_OK) {
cerr << "Cannot bind parameter: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_bind_int(stmt, 2, age);
if (rc != SQLITE_OK) {
cerr << "Cannot bind parameter: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_bind_text(stmt, 3, gender.c_str(), -1, SQLITE_STATIC);
if (rc != SQLITE_OK) {
cerr << "Cannot bind parameter: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_bind_text(stmt, 4, phone.c_str(), -1, SQLITE_STATIC);
if (rc != SQLITE_OK) {
cerr << "Cannot bind parameter: " << sqlite3_errmsg(db) << endl;
return;
}
rc = sqlite3_step(stmt);
if (rc != SQLITE_DONE) {
cerr << "Cannot execute statement: " << sqlite3_errmsg(db) << endl;
return;
}
cout << "Add new user successfully." << endl;
sqlite3_finalize(stmt);
}
private:
sqlite3* db; // SQLite数据库对象
};
int main() {
ManagementSystem system;
// 测试功能
system.purchaseEquipment("Basketball", "Nike", 10, 20.0);
system.purchaseEquipment("Football", "Adidas", 20, 30.0);
system.borrowEquipment("Basketball", 5);
system.borrowEquipment("Basketball", 10);
system.returnEquipment("Basketball", 3);
system.scrapEquipment("Football");
system.viewInventory();
system.addNewUser();
system.managePersonalInfo();
return 0;
}
```
注意,该示例代码仅为演示用途,实际开发中还需要进行更多的测试和完善。同时,还需要注意数据库的安全性和稳定性,例如加密、备份等方面。
相关推荐
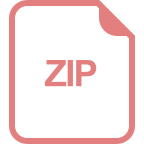
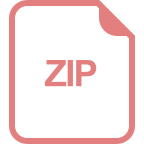















