python实现des加解密算法
时间: 2023-07-26 19:43:39 浏览: 78
Python中可以使用crypto库来实现DES加解密算法。以下是一个简单的示例代码:
```python
from Crypto.Cipher import DES
# 加密函数
def encryptDES(key, plaintext):
cipher = DES.new(key, DES.MODE_ECB)
# 需要将明文填充为8的倍数
plaintext += (8 - len(plaintext) % 8) * '\0'
ciphertext = cipher.encrypt(plaintext.encode('utf-8'))
# 将字节流转换为16进制字符串
return ciphertext.hex()
# 解密函数
def decryptDES(key, ciphertext):
cipher = DES.new(key, DES.MODE_ECB)
# 将16进制字符串转换为字节流
ciphertext = bytes.fromhex(ciphertext)
plaintext = cipher.decrypt(ciphertext).decode('utf-8')
# 去掉填充的空字符
return plaintext.rstrip('\0')
# 测试
key = b'12345678' # 8字节的密钥
plaintext = 'hello world'
ciphertext = encryptDES(key, plaintext)
print(ciphertext)
plaintext = decryptDES(key, ciphertext)
print(plaintext)
```
注意,DES算法被认为是不安全的,推荐使用更加安全的算法,比如AES。
阅读全文
相关推荐
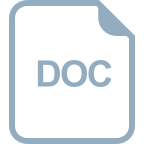
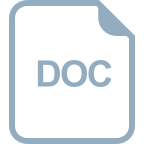
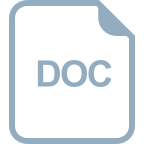



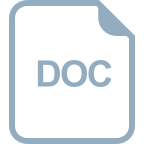




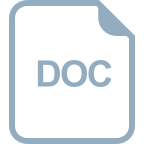
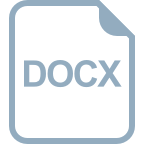
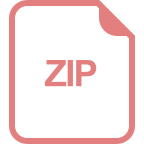