编写一个名为TwoDimensionalShape的抽象类,拥有属性area和circumference表示面积和周长,以及抽象方法getArea()和getCircumference(),用于获取面积和周长。 2、 编写Printable接口,包括一个抽象方法printShapeInfo,用于输出图形信息。 3、 分别编写Rectangle、Triangle、Circular三个类,用于描述矩形、三角形和圆形,要求继承于TwoDimensionalShap类,并实现Printable接口,每个图形有各自的构造方法,矩形有length和width字段表示长和宽,三角形有base、hypotenus1和hypotenus2字段表示底和两条斜边,圆形有radius字段表示半径,按几何常识实现父类中所定义的抽象方法,printShapeInfo方法要求能够输出: (1) 图形的类型(使用getClass方法) (2) 图形的基本数据(使用自定义toString方法,输出图形的字段,输出示例:宽3.0, 长4.0) 4、 编写一个名为ShapeDetector的类,拥有三个重载的detectShape方法,分别为detectShape(Rectangle r)、detectShape(Triangle t)、detectShape(Circular c),分别输出所接收到的图形的类型。 5、 编写Test类,创建一个名为Shapes的TwoDimensionalShape型数组,并创建Rectangle、Triangle、Circular的对象存储在该数组中,用foreach循环遍历该数组,使用detectShape方法输出该对象的图形类型,用printShapeInfo方法输出该对象的图形信息,然后分别用getArea和getCircumference方法输出面积及周长。 6、(可选)给三个图形的构造方法加上验证功能,保证所输入三角形三边能够组成三角形,圆形半径、矩形的长和宽为正实数。 要求:程序文件放置在合适的包中,保证程序有良好的组织性,在编写过程中尽量保证程序的良好封装性。
时间: 2024-03-25 10:36:21 浏览: 54
好的,这是一个关于面向对象编程的问题,非常实用。以下是代码示例:
TwoDimensionalShape.java
```
public abstract class TwoDimensionalShape {
protected double area;
protected double circumference;
public abstract double getArea();
public abstract double getCircumference();
}
```
Printable.java
```
public interface Printable {
public abstract void printShapeInfo();
}
```
Rectangle.java
```
public class Rectangle extends TwoDimensionalShape implements Printable {
private double length;
private double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
@Override
public double getArea() {
area = length * width;
return area;
}
@Override
public double getCircumference() {
circumference = 2 * (length + width);
return circumference;
}
@Override
public void printShapeInfo() {
System.out.println("Type: " + this.getClass().getSimpleName());
System.out.println("Length: " + length);
System.out.println("Width: " + width);
}
}
```
Triangle.java
```
public class Triangle extends TwoDimensionalShape implements Printable {
private double base;
private double hypotenuse1;
private double hypotenuse2;
public Triangle(double base, double hypotenuse1, double hypotenuse2) {
this.base = base;
this.hypotenuse1 = hypotenuse1;
this.hypotenuse2 = hypotenuse2;
}
@Override
public double getArea() {
double s = (base + hypotenuse1 + hypotenuse2) / 2;
area = Math.sqrt(s * (s - base) * (s - hypotenuse1) * (s - hypotenuse2));
return area;
}
@Override
public double getCircumference() {
circumference = base + hypotenuse1 + hypotenuse2;
return circumference;
}
@Override
public void printShapeInfo() {
System.out.println("Type: " + this.getClass().getSimpleName());
System.out.println("Base: " + base);
System.out.println("Hypotenuse 1: " + hypotenuse1);
System.out.println("Hypotenuse 2: " + hypotenuse2);
}
}
```
Circular.java
```
public class Circular extends TwoDimensionalShape implements Printable {
private double radius;
public Circular(double radius) {
this.radius = radius;
}
@Override
public double getArea() {
area = Math.PI * radius * radius;
return area;
}
@Override
public double getCircumference() {
circumference = 2 * Math.PI * radius;
return circumference;
}
@Override
public void printShapeInfo() {
System.out.println("Type: " + this.getClass().getSimpleName());
System.out.println("Radius: " + radius);
}
}
```
ShapeDetector.java
```
public class ShapeDetector {
public void detectShape(Rectangle r) {
System.out.println("This is a rectangle.");
}
public void detectShape(Triangle t) {
System.out.println("This is a triangle.");
}
public void detectShape(Circular c) {
System.out.println("This is a circular.");
}
}
```
Test.java
```
public class Test {
public static void main(String[] args) {
TwoDimensionalShape[] shapes = new TwoDimensionalShape[3];
shapes[0] = new Rectangle(4, 3);
shapes[1] = new Triangle(3, 4, 5);
shapes[2] = new Circular(2);
ShapeDetector detector = new ShapeDetector();
for(TwoDimensionalShape shape : shapes) {
detector.detectShape(shape);
shape.printShapeInfo();
System.out.println("Area: " + shape.getArea());
System.out.println("Circumference: " + shape.getCircumference());
System.out.println();
}
}
}
```
阅读全文
相关推荐
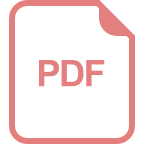
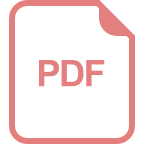
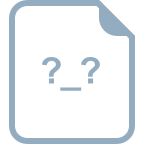

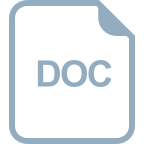


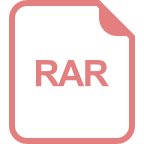
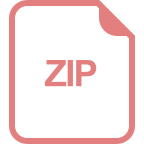
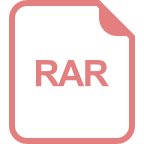
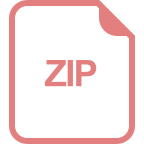
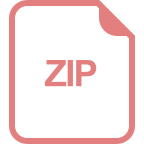
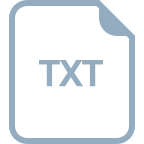
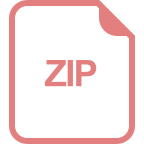
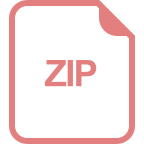